Add a timer in the following code. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long elapsedTime; public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; String playerName = JOptionPane.showInputDialog("Enter your name:"); player.setName(playerName); elapsedTime = 0; timer = new Timer(1000, e -> { elapsedTime++; repaint(); }); timer.start(); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());}
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Add a timer in the following code.
public class GameGUI extends JPanel {
private final Labyrinth labyrinth;
private final Player player;
private final Dragon dragon;
private Timer timer;
private long elapsedTime;
public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) {
this.labyrinth = labyrinth;
this.player = player;
this.dragon = dragon;
String playerName = JOptionPane.showInputDialog("Enter your name:");
player.setName(playerName);
elapsedTime = 0;
timer = new Timer(1000, e -> {
elapsedTime++;
repaint();
});
timer.start();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());}

Step by step
Solved in 2 steps

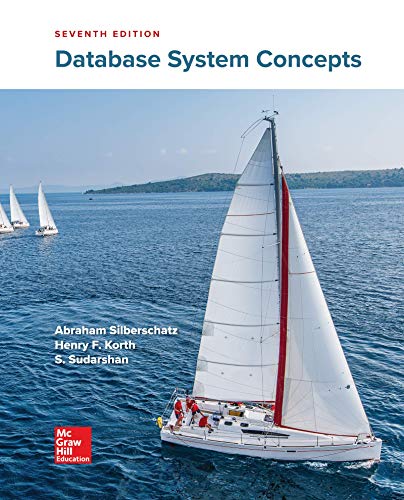
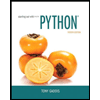
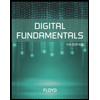
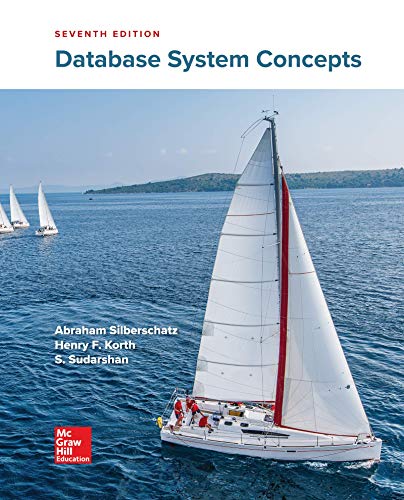
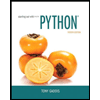
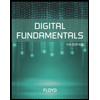
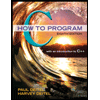
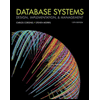
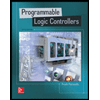