Change the following code so that the player can see only the neighboring fields at a distance of 3 units. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png"); public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; setFocusable(true); addKeyListener(new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { char move = switch (e.getKeyCode()) { case KeyEvent.VK_W -> 'W'; case KeyEvent.VK_S -> 'S'; case KeyEvent.VK_A -> 'A'; case KeyEvent.VK_D -> 'D'; default -> ' '; }; if (move != ' ') { player.move(move, labyrinth); dragon.move(labyrinth); repaint(); checkGameState(); } } }); } private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over."); System.exit(0); } } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize()); for (int i = 0; i < labyrinth.getSize(); i++) { for (int j = 0; j < labyrinth.getSize(); j++) { if (labyrinth.getCell(i, j).isWall()) { wallIcon.paintIcon(this, g, j * cellSize, i * cellSize); } else if(!labyrinth.getCell(i, j).isWall()){ emptyIcon.paintIcon(this, g, j * cellSize, i * cellSize); } } } playerIcon.paintIcon(this, g, player.getY() * cellSize, player.getX() * cellSize); dragonIcon.paintIcon(this, g, dragon.getY() * cellSize, dragon.getX() * cellSize); } @Override public Dimension getPreferredSize() { return new Dimension(320, 320); }} public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { if (size < 2) { throw new IllegalArgumentException("Labyrinth size must be at least 2x2."); } this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Change the following code so that the player can see only the neighboring fields at a distance of 3 units.
public class GameGUI extends JPanel {
private final Labyrinth labyrinth;
private final Player player;
private final Dragon dragon;
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");
private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png");
private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png");
private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png");
public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) {
this.labyrinth = labyrinth;
this.player = player;
this.dragon = dragon;
setFocusable(true);
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
char move = switch (e.getKeyCode()) {
case KeyEvent.VK_W -> 'W';
case KeyEvent.VK_S -> 'S';
case KeyEvent.VK_A -> 'A';
case KeyEvent.VK_D -> 'D';
default -> ' ';
};
if (move != ' ') {
player.move(move, labyrinth);
dragon.move(labyrinth);
repaint();
checkGameState();
}
}
});
}
private void checkGameState() {
if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) {
JOptionPane.showMessageDialog(this, "You escaped! Congratulations!");
System.exit(0);
}
if (Math.abs(player.getX() - dragon.getX()) <= 1 &&
Math.abs(player.getY() - dragon.getY()) <= 1) {
JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");
System.exit(0);
}
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());
for (int i = 0; i < labyrinth.getSize(); i++) {
for (int j = 0; j < labyrinth.getSize(); j++) {
if (labyrinth.getCell(i, j).isWall()) {
wallIcon.paintIcon(this, g, j * cellSize, i * cellSize);
} else if(!labyrinth.getCell(i, j).isWall()){
emptyIcon.paintIcon(this, g, j * cellSize, i * cellSize);
}
}
}
playerIcon.paintIcon(this, g, player.getY() * cellSize, player.getX() * cellSize);
dragonIcon.paintIcon(this, g, dragon.getY() * cellSize, dragon.getX() * cellSize);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(320, 320);
}
}
public class Labyrinth {
private final int size;
private final Cell[][] grid;
public Labyrinth(int size) {
if (size < 2) {
throw new IllegalArgumentException("Labyrinth size must be at least 2x2.");
}
this.size = size;
this.grid = new Cell[size][size];
generateLabyrinth();
}
}

Step by step
Solved in 2 steps

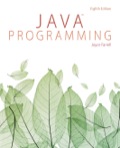
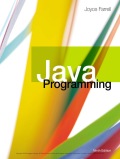
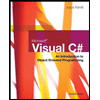
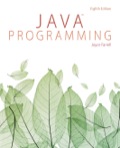
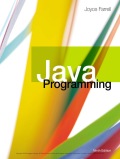
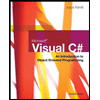
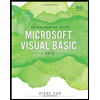
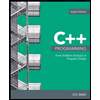