The following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum score } return; // Exit after finding course } } // If course not found, add new course and score if (gameCount < 20) { courses[gameCount] = courseName; scores[gameCount] = gameScore; ++gameCount; } } void Player::ReportPlayer(int playerId) const { if (gameCount == 0) { std::cout << "Player P" << playerId << " has no games\n"; } else { std::cout << "Player P" << playerId << "\n"; for (int i = 0; i < gameCount; ++i) { std::cout << "\t" << courses[i] << " " << scores[i] << "\n"; } } } #include <iostream> #include <string> #include "player.h" int main() { Player players[10]; // Array to hold players std::string playerCode; // Corrected from str::string to std::string int inId, inScore; std::string inCourse; int maxPlayerIndex = -1; // Keep track of highest player id while (true) { std::cout << "Enter player code or Q to quit: "; std::cin >> playerCode; if (playerCode == 'q' || playerCode == 'Q') break; // Check if input is "P0" to "P9" if (playerCode.length() != 2 || playerCode[0] != 'P' || playerCode[1] < '0' || playerCode[1] > '9') { std::cout << "Invalid player code. Please enter P0-P9.\n"; continue; } // Convert player code to integer inId = playerCode[1] - '0'; maxPlayerIndex = std::max(maxPlayerIndex, inId); std::cin.ignore(); // Clear newline character from input buffer std::cout << "Enter course name: "; std::getline(std::cin, inCourse); std::cout << "Enter game score: "; std::cin >> inScore; std::cin.ignore(); // Clear input buffer again std::cout << std::endl; // Register game for player players[inId].CheckGame(inId, inCourse, inScore); } // Report all players from P0 to Pmax std::cout << "\nReport\n"; for (int i = 0; i <= maxPlayerIndex; ++i) { players[i].ReportPlayer(i); } return 0; } Are there any errors in this code that may prevent it from compiling?
The following is code for a disc golf program written in C++:
// player.h
#ifndef PLAYER_H
#define PLAYER_H
#include <string>
#include <iostream>
class Player {
private:
std::string courses[20]; // Array of course names
int scores[20]; // Array of scores
int gameCount; // Number of games played
public:
Player(); // Constructor
void CheckGame(int playerId, const std::string& courseName, int gameScore);
void ReportPlayer(int playerId) const;
};
#endif // PLAYER_H
// player.cpp
#include "player.h"
#include <iomanip>
Player::Player() : gameCount(0) {}
void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) {
for (int i = 0; i < gameCount; ++i) {
if (courses[i] == courseName) {
// If course has been played, then check for minimum score
if (gameScore < scores[i]) {
scores[i] = gameScore; // Update to new minimum score
}
return; // Exit after finding course
}
}
// If course not found, add new course and score
if (gameCount < 20) {
courses[gameCount] = courseName;
scores[gameCount] = gameScore;
++gameCount;
}
}
void Player::ReportPlayer(int playerId) const {
if (gameCount == 0) {
std::cout << "Player P" << playerId << " has no games\n";
} else {
std::cout << "Player P" << playerId << "\n";
for (int i = 0; i < gameCount; ++i) {
std::cout << "\t" << courses[i] << " " << scores[i] << "\n";
}
}
}
#include <iostream>
#include <string>
#include "player.h"
int main() {
Player players[10]; // Array to hold players
std::string playerCode; // Corrected from str::string to std::string
int inId, inScore;
std::string inCourse;
int maxPlayerIndex = -1; // Keep track of highest player id
while (true) {
std::cout << "Enter player code or Q to quit: ";
std::cin >> playerCode;
if (playerCode == 'q' || playerCode == 'Q') break;
// Check if input is "P0" to "P9"
if (playerCode.length() != 2 || playerCode[0] != 'P' || playerCode[1] < '0' || playerCode[1] > '9') {
std::cout << "Invalid player code. Please enter P0-P9.\n";
continue;
}
// Convert player code to integer
inId = playerCode[1] - '0';
maxPlayerIndex = std::max(maxPlayerIndex, inId);
std::cin.ignore(); // Clear newline character from input buffer
std::cout << "Enter course name: ";
std::getline(std::cin, inCourse);
std::cout << "Enter game score: ";
std::cin >> inScore;
std::cin.ignore(); // Clear input buffer again
std::cout << std::endl;
// Register game for player
players[inId].CheckGame(inId, inCourse, inScore);
}
// Report all players from P0 to Pmax
std::cout << "\nReport\n";
for (int i = 0; i <= maxPlayerIndex; ++i) {
players[i].ReportPlayer(i);
}
return 0;
}
Are there any errors in this code that may prevent it from compiling?

Step by step
Solved in 2 steps

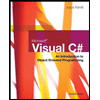
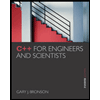
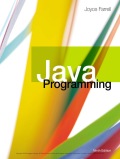
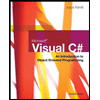
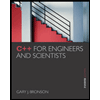
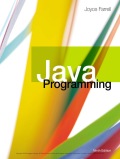
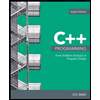
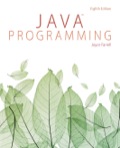