Part I: Brainstorming and Planning 1. Design Documentation (15 points) о UML Diagram: Create using Figma, ensuring access modifiers and data types are included. 。 Algorithm: Describe the store's functionality and operations. о Flow Chart: Create using Canva to visually represent the workflow, employing appropriate shapes and patterns. Part II: Implementation and Design 2. Store Functionality (15 points) 。 Implement a Store class that serves as a base for various store types. o Manage inventory and pricing for each item using an Array or ArrayList of store objects. Example: 3. User Interaction (15 points) Use an ArrayList to hold car objects for a CarStore class. Use an ArrayList to hold clothing objects for a ClothingStore class. 。 Prompt the user for store details (store type, name, and location). ° Collect customer details (name, age, address, phone number, email) using an instance of the Customer class. ° Utilize JavaFX for all program functionalities. о Enable users to purchase items continuously until they decide to finish shopping. о Calculate the total purchase amount, including a 7% sales tax. 。 Implement at least three discount options based on total spending: Spend $25 or more: 5% discount " Spend $50 or more: 10% discount Spend $100 or more: 20% discount 4. Data Handling (15 points) о Create a menu that allows users to select from the following options: " Create a store View inventory . View customers " Add customers • Upload inventory • Purchase items Upload video testimony Exit the program 5. Implementation Details (15 points) ° Store Class: ° 0 ° о • Fields: Store Type, Store Name, Store Location Methods: Accessors, mutators, toString for output formatting, and display inventory items. Constructors: " No-argument constructor for default values. Constructor with parameters for specific values. ClothingStore Class (inherits from Store): " Additional fields/methods specific to clothing items. · Constructors similar to the Store class. CarDealership Class (inherits from Store): • Additional fields/methods specific to cars. Constructors similar to the Store class. Customer Class: ' Fields: Name, Age, Address, Phone, Email Methods: Accessors, mutators, toString for output formatting. Constructors: Main Class: No-argument constructor for default values. Constructor with parameters for specific values. Use the final keyword for constants. Utilize an ArrayList or Array to hold store items. • Include a method to calculate the total price, formatted to two decimal places. 7. Input and Output (10 points) о Input: о " Validate user input to prevent invalid data types and handle exceptions. Prevent transactions involving unselected items or negative values. Implement exception handling as necessary. Output: " Display all purchased items, their prices (formatted to two decimal places), discounts, and tax amounts in a tabular format. Refer to program challenges in the textbook for examples. " Apply custom styling throughout your application.
Part I: Brainstorming and Planning 1. Design Documentation (15 points) о UML Diagram: Create using Figma, ensuring access modifiers and data types are included. 。 Algorithm: Describe the store's functionality and operations. о Flow Chart: Create using Canva to visually represent the workflow, employing appropriate shapes and patterns. Part II: Implementation and Design 2. Store Functionality (15 points) 。 Implement a Store class that serves as a base for various store types. o Manage inventory and pricing for each item using an Array or ArrayList of store objects. Example: 3. User Interaction (15 points) Use an ArrayList to hold car objects for a CarStore class. Use an ArrayList to hold clothing objects for a ClothingStore class. 。 Prompt the user for store details (store type, name, and location). ° Collect customer details (name, age, address, phone number, email) using an instance of the Customer class. ° Utilize JavaFX for all program functionalities. о Enable users to purchase items continuously until they decide to finish shopping. о Calculate the total purchase amount, including a 7% sales tax. 。 Implement at least three discount options based on total spending: Spend $25 or more: 5% discount " Spend $50 or more: 10% discount Spend $100 or more: 20% discount 4. Data Handling (15 points) о Create a menu that allows users to select from the following options: " Create a store View inventory . View customers " Add customers • Upload inventory • Purchase items Upload video testimony Exit the program 5. Implementation Details (15 points) ° Store Class: ° 0 ° о • Fields: Store Type, Store Name, Store Location Methods: Accessors, mutators, toString for output formatting, and display inventory items. Constructors: " No-argument constructor for default values. Constructor with parameters for specific values. ClothingStore Class (inherits from Store): " Additional fields/methods specific to clothing items. · Constructors similar to the Store class. CarDealership Class (inherits from Store): • Additional fields/methods specific to cars. Constructors similar to the Store class. Customer Class: ' Fields: Name, Age, Address, Phone, Email Methods: Accessors, mutators, toString for output formatting. Constructors: Main Class: No-argument constructor for default values. Constructor with parameters for specific values. Use the final keyword for constants. Utilize an ArrayList or Array to hold store items. • Include a method to calculate the total price, formatted to two decimal places. 7. Input and Output (10 points) о Input: о " Validate user input to prevent invalid data types and handle exceptions. Prevent transactions involving unselected items or negative values. Implement exception handling as necessary. Output: " Display all purchased items, their prices (formatted to two decimal places), discounts, and tax amounts in a tabular format. Refer to program challenges in the textbook for examples. " Apply custom styling throughout your application.
Chapter3: Data Representation
Section: Chapter Questions
Problem 3RP
Related questions
Question
Hello, wanting help in finding a solution to this java challenge.

Transcribed Image Text:Part I: Brainstorming and Planning
1. Design Documentation (15 points)
о UML Diagram: Create using Figma, ensuring access modifiers and data
types are included.
。 Algorithm: Describe the store's functionality and operations.
о Flow Chart: Create using Canva to visually represent the workflow,
employing appropriate shapes and patterns.
Part II: Implementation and Design
2. Store Functionality (15 points)
。 Implement a Store class that serves as a base for various store types.
o Manage inventory and pricing for each item using an Array or ArrayList of
store objects.
Example:
3. User Interaction (15 points)
Use an ArrayList to hold car objects for a CarStore class.
Use an ArrayList to hold clothing objects for a ClothingStore
class.
。 Prompt the user for store details (store type, name, and location).
°
Collect customer details (name, age, address, phone number, email) using
an instance of the Customer class.
°
Utilize JavaFX for all program functionalities.
о Enable users to purchase items continuously until they decide to finish
shopping.
о Calculate the total purchase amount, including a 7% sales tax.
。 Implement at least three discount options based on total spending:
Spend $25 or more: 5% discount
"
Spend $50 or more: 10% discount
Spend $100 or more: 20% discount
4. Data Handling (15 points)
о
Create a menu that allows users to select from the following options:
"
Create a store
View inventory
. View customers
"
Add customers
•
Upload inventory
•
Purchase items
Upload video testimony
Exit the program
5. Implementation Details (15 points)
° Store Class:
°
0
°
о
•
Fields: Store Type, Store Name, Store Location
Methods: Accessors, mutators, toString for output formatting, and
display inventory items.
Constructors:
"
No-argument constructor for default values.
Constructor with parameters for specific values.
ClothingStore Class (inherits from Store):
"
Additional fields/methods specific to clothing items.
· Constructors similar to the Store class.
CarDealership Class (inherits from Store):
•
Additional fields/methods specific to cars.
Constructors similar to the Store class.
Customer Class:
'
Fields: Name, Age, Address, Phone, Email
Methods: Accessors, mutators, toString for output formatting.
Constructors:
Main Class:
No-argument constructor for default values.
Constructor with parameters for specific values.
Use the final keyword for constants.
Utilize an ArrayList or Array to hold store items.
•
Include a method to calculate the total price, formatted to two
decimal places.
7. Input and Output (10 points)
о
Input:
о
"
Validate user input to prevent invalid data types and handle
exceptions.
Prevent transactions involving unselected items or negative values.
Implement exception handling as necessary.
Output:
"
Display all purchased items, their prices (formatted to two decimal
places), discounts, and tax amounts in a tabular format.
Refer to program challenges in the textbook for examples.
"
Apply custom styling throughout your application.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
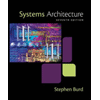
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
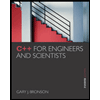
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
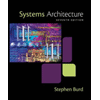
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
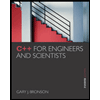
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
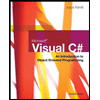
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,