In this assignment, you will implement a simple OS scheduler using C/C++. The scheduler's task is to receive a set of processes and their details and then decide the order of executing these processes based on the chosen algorithm. Finally, the scheduler will output the order of process execution, in addition to some stats about each of the processes. The scheduling algorithm chosen for this assignment will be a non-preemptive Ranking Scheduling Algorithm. The input will start with an integer N, representing the number of processes, followed by N lines (one for each process). For each line i, the line will start with a string s, representing the process name (can be multiple characters), followed by three numbers representing the arrival time, processing time, and the rank for the ith process, respectively. These values will be separated by tabs (i.e. \\t'). The values for input numbers can be up to 100,000,000. The main aspect of Ranking Scheduling Algorithm is the rank value for each process (3rd column). We always give priority for process that are ranked first (i.e. lower ranking value). However, If multiple processes have the same ranking value, we decide based on the arrival time. In the case where processes have the same ranking and arrival time, we choose the process that had its name listed first in the input file. For each process, your program should print a line showing the process's name, response time, turnaround time, and delay. The order of these lines should match the execution order. After that, your program should print a line indicating the order of executing the processes. See the sample output below for details. The input will be read from a file (in.txt), and the output should be written to a file (out.txt). The output format must strictly match the formatting shown in the sample output. ヨ Grading Rules: • • • Each student is expected to fully understand all the aspects and details of the entire code. The grading will take place in a Linux environment (Ubuntu, using the g++ compiler). You MUST implement the code using this same environment to get a full mark. Your grade will depend on multiple aspects, such as: 1. The correctness of your code's output across multiple secret test cases 2. Your code's ability to handle corner test cases like having many processes or large processing times. 3. Your answers to the questions during the discussion Sample Input (file in.txt): 5 A 033 C 4 4 4 B 2 6 6 D 6 5 5 00 8 2 2 Sample output (file out.txt): A: (response=0, turnaround=3, delay=0) B: (response=1, turnaround=7, delay=1) E: (response=1, turnaround=3, delay=1) C: (response=7, turnaround=11, delay=7) D: (response=9, turnaround=14, delay=9) ABECD
In this assignment, you will implement a simple OS scheduler using C/C++. The scheduler's task is to receive a set of processes and their details and then decide the order of executing these processes based on the chosen algorithm. Finally, the scheduler will output the order of process execution, in addition to some stats about each of the processes. The scheduling algorithm chosen for this assignment will be a non-preemptive Ranking Scheduling Algorithm. The input will start with an integer N, representing the number of processes, followed by N lines (one for each process). For each line i, the line will start with a string s, representing the process name (can be multiple characters), followed by three numbers representing the arrival time, processing time, and the rank for the ith process, respectively. These values will be separated by tabs (i.e. \\t'). The values for input numbers can be up to 100,000,000. The main aspect of Ranking Scheduling Algorithm is the rank value for each process (3rd column). We always give priority for process that are ranked first (i.e. lower ranking value). However, If multiple processes have the same ranking value, we decide based on the arrival time. In the case where processes have the same ranking and arrival time, we choose the process that had its name listed first in the input file. For each process, your program should print a line showing the process's name, response time, turnaround time, and delay. The order of these lines should match the execution order. After that, your program should print a line indicating the order of executing the processes. See the sample output below for details. The input will be read from a file (in.txt), and the output should be written to a file (out.txt). The output format must strictly match the formatting shown in the sample output. ヨ Grading Rules: • • • Each student is expected to fully understand all the aspects and details of the entire code. The grading will take place in a Linux environment (Ubuntu, using the g++ compiler). You MUST implement the code using this same environment to get a full mark. Your grade will depend on multiple aspects, such as: 1. The correctness of your code's output across multiple secret test cases 2. Your code's ability to handle corner test cases like having many processes or large processing times. 3. Your answers to the questions during the discussion Sample Input (file in.txt): 5 A 033 C 4 4 4 B 2 6 6 D 6 5 5 00 8 2 2 Sample output (file out.txt): A: (response=0, turnaround=3, delay=0) B: (response=1, turnaround=7, delay=1) E: (response=1, turnaround=3, delay=1) C: (response=7, turnaround=11, delay=7) D: (response=9, turnaround=14, delay=9) ABECD
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 7RQ
Related questions
Question
Touch and hold a clip to pin it. Unpinned clips will be deleted after 1 hour.Touch and hold a clip to pin it. Unpinned clips will be deleted after 1 hour.Touch and hold a clip to pin it. Unpinned clips will be deleted after 1 hour.Touch and hold a clip to pin it. Unpinned clips will be deleted after 1 hour.Touch and hold a clip to pin it. Unpinned clips will be deleted after 1 hour.

Transcribed Image Text:In this assignment, you will implement a simple OS scheduler using C/C++. The scheduler's task is to
receive a set of processes and their details and then decide the order of executing these processes
based on the chosen algorithm. Finally, the scheduler will output the order of process execution, in
addition to some stats about each of the processes.
The scheduling algorithm chosen for this assignment will be a non-preemptive Ranking Scheduling
Algorithm. The input will start with an integer N, representing the number of processes, followed by N
lines (one for each process). For each line i, the line will start with a string s, representing the process
name (can be multiple characters), followed by three numbers representing the arrival time, processing
time, and the rank for the ith process, respectively. These values will be separated by tabs (i.e. \\t'). The
values for input numbers can be up to 100,000,000.
The main aspect of Ranking Scheduling Algorithm is the rank value for each process (3rd column). We
always give priority for process that are ranked first (i.e. lower ranking value). However, If multiple
processes have the same ranking value, we decide based on the arrival time. In the case where
processes have the same ranking and arrival time, we choose the process that had its name listed first in
the input file.
For each process, your program should print a line showing the process's name, response time,
turnaround time, and delay. The order of these lines should match the execution order. After that, your
program should print a line indicating the order of executing the processes. See the sample output
below for details.
The input will be read from a file (in.txt), and the output should be written to a file (out.txt). The output
format must strictly match the formatting shown in the sample output.

Transcribed Image Text:ヨ
Grading Rules:
•
•
•
Each student is expected to fully understand all the aspects and details of the entire code.
The grading will take place in a Linux environment (Ubuntu, using the g++ compiler).
You MUST implement the code using this same environment to get a full mark.
Your grade will depend on multiple aspects, such as:
1. The correctness of your code's output across multiple secret test cases
2. Your code's ability to handle corner test cases like having many processes or large
processing times.
3. Your answers to the questions during the discussion
Sample Input (file in.txt):
5
A 033
C
4
4 4
B
2 6
6
D 6
5
5
00
8
2 2
Sample output (file out.txt):
A: (response=0, turnaround=3, delay=0)
B: (response=1, turnaround=7, delay=1)
E: (response=1, turnaround=3, delay=1)
C: (response=7, turnaround=11, delay=7)
D: (response=9, turnaround=14, delay=9)
ABECD
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
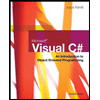
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
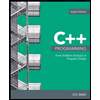
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
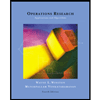
Operations Research : Applications and Algorithms
Computer Science
ISBN:
9780534380588
Author:
Wayne L. Winston
Publisher:
Brooks Cole
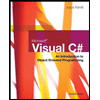
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
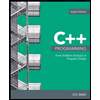
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
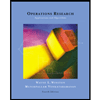
Operations Research : Applications and Algorithms
Computer Science
ISBN:
9780534380588
Author:
Wayne L. Winston
Publisher:
Brooks Cole
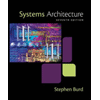
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
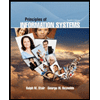
Principles of Information Systems (MindTap Course…
Computer Science
ISBN:
9781285867168
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning