fix any duplicate error in this code import javax.swing.*;import java.awt.*;import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;class MazeSolver extends JFrame {private static int n;private static char[][] maze;private boolean[][] visited;private int startX, startY, goalX, goalY;private MazePanel mazePanel;public MazeSolver(String filename) throws IOException {readMaze(filename);visited = new boolean[n][n];setTitle("Maze Solver");setSize(n * 40, n * 40);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);mazePanel = new MazePanel(maze, n);add(mazePanel);setVisible(true);}public void readMaze(String filename) throws IOException {BufferedReader br = new BufferedReader(new FileReader(filename));n = Integer.parseInt(br.readLine());maze = new char[n][n];for (int i = 0; i < n; i++) {String line = br.readLine();for (int j = 0; j < n; j++) {maze[i][j] = line.charAt(j);if (maze[i][j] == 'S') {startX = i;startY = j;} else if (maze[i][j] == 'G') {goalX = i;goalY = j;}}}br.close();}private boolean solveMaze(int x, int y) {// Debug informationSystem.out.println("Visiting: (" + x + ", " + y + ")");if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {System.out.println("Out of bounds or already visited or wall at: (" + x + ", " + y + ")");return false;}if (x == goalX && y == goalY) {maze[x][y] = 'G';mazePanel.repaint();return true;}visited[x][y] = true;maze[x][y] = '*';mazePanel.repaint();try {Thread.sleep(100);} catch (InterruptedException e) {e.printStackTrace();}// Try to move in all directionsif (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {return true;}// Backtrackmaze[x][y] = '.';mazePanel.repaint();visited[x][y] = false;return false;}public void solveMaze() {if (solveMaze(startX, startY)) {System.out.println("Path found!");} else {System.out.println("No path found.");}}private boolean solveMaze(int x, int y) {if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {return false;}if (x == goalX && y == goalY) {maze[x][y] = 'G';mazePanel.repaint();return true;}visited[x][y] = true;maze[x][y] = '*';mazePanel.repaint();try {Thread.sleep(100);} catch (InterruptedException e) {e.printStackTrace();}if (this.solveMaze(x + 1, y) || this.solveMaze(x - 1, y) || this.solveMaze(x, y + 1) || this.solveMaze(x, y - 1)) {return true;}maze[x][y] = '.';mazePanel.repaint();visited[x][y] = false;return false;}public static void main(String[] args) throws IOException {MazeSolver solver = new MazeSolver("maze.txt");solver.solveMaze();{for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {System.out.print(maze[i][j] + " ");}System.out.println();}}}public void displayMaze() {}}class MazePanel extends JPanel {private final char[][] maze;private final int n;public MazePanel(char[][] maze, int n) {this.maze = maze;this.n = n;}@Overrideprotected void paintComponent(Graphics g) {super.paintComponent(g);int cellSize = 40;for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {switch (maze[i][j]) {case '#' -> g.setColor(Color.BLACK);case '.' -> g.setColor(Color.WHITE);case 'S' -> g.setColor(Color.BLUE);case 'G' -> g.setColor(Color.RED);case '*' -> g.setColor(Color.GREEN);default -> g.setColor(Color.WHITE);}g.fillRect(j * cellSize, i * cellSize, cellSize, cellSize);g.setColor(Color.GRAY);g.drawRect(j * cellSize, i * cellSize, cellSize, cellSize);}}}}
fix any duplicate error in this code import javax.swing.*;import java.awt.*;import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;class MazeSolver extends JFrame {private static int n;private static char[][] maze;private boolean[][] visited;private int startX, startY, goalX, goalY;private MazePanel mazePanel;public MazeSolver(String filename) throws IOException {readMaze(filename);visited = new boolean[n][n];setTitle("Maze Solver");setSize(n * 40, n * 40);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);mazePanel = new MazePanel(maze, n);add(mazePanel);setVisible(true);}public void readMaze(String filename) throws IOException {BufferedReader br = new BufferedReader(new FileReader(filename));n = Integer.parseInt(br.readLine());maze = new char[n][n];for (int i = 0; i < n; i++) {String line = br.readLine();for (int j = 0; j < n; j++) {maze[i][j] = line.charAt(j);if (maze[i][j] == 'S') {startX = i;startY = j;} else if (maze[i][j] == 'G') {goalX = i;goalY = j;}}}br.close();}private boolean solveMaze(int x, int y) {// Debug informationSystem.out.println("Visiting: (" + x + ", " + y + ")");if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {System.out.println("Out of bounds or already visited or wall at: (" + x + ", " + y + ")");return false;}if (x == goalX && y == goalY) {maze[x][y] = 'G';mazePanel.repaint();return true;}visited[x][y] = true;maze[x][y] = '*';mazePanel.repaint();try {Thread.sleep(100);} catch (InterruptedException e) {e.printStackTrace();}// Try to move in all directionsif (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {return true;}// Backtrackmaze[x][y] = '.';mazePanel.repaint();visited[x][y] = false;return false;}public void solveMaze() {if (solveMaze(startX, startY)) {System.out.println("Path found!");} else {System.out.println("No path found.");}}private boolean solveMaze(int x, int y) {if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {return false;}if (x == goalX && y == goalY) {maze[x][y] = 'G';mazePanel.repaint();return true;}visited[x][y] = true;maze[x][y] = '*';mazePanel.repaint();try {Thread.sleep(100);} catch (InterruptedException e) {e.printStackTrace();}if (this.solveMaze(x + 1, y) || this.solveMaze(x - 1, y) || this.solveMaze(x, y + 1) || this.solveMaze(x, y - 1)) {return true;}maze[x][y] = '.';mazePanel.repaint();visited[x][y] = false;return false;}public static void main(String[] args) throws IOException {MazeSolver solver = new MazeSolver("maze.txt");solver.solveMaze();{for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {System.out.print(maze[i][j] + " ");}System.out.println();}}}public void displayMaze() {}}class MazePanel extends JPanel {private final char[][] maze;private final int n;public MazePanel(char[][] maze, int n) {this.maze = maze;this.n = n;}@Overrideprotected void paintComponent(Graphics g) {super.paintComponent(g);int cellSize = 40;for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {switch (maze[i][j]) {case '#' -> g.setColor(Color.BLACK);case '.' -> g.setColor(Color.WHITE);case 'S' -> g.setColor(Color.BLUE);case 'G' -> g.setColor(Color.RED);case '*' -> g.setColor(Color.GREEN);default -> g.setColor(Color.WHITE);}g.fillRect(j * cellSize, i * cellSize, cellSize, cellSize);g.setColor(Color.GRAY);g.drawRect(j * cellSize, i * cellSize, cellSize, cellSize);}}}}
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
fix any duplicate error in this code
import javax.swing.*;
import java.awt.*;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
class MazeSolver extends JFrame {
private static int n;
private static char[][] maze;
private boolean[][] visited;
private int startX, startY, goalX, goalY;
private MazePanel mazePanel;
public MazeSolver(String filename) throws IOException {
readMaze(filename);
visited = new boolean[n][n];
setTitle("Maze Solver");
setSize(n * 40, n * 40);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
mazePanel = new MazePanel(maze, n);
add(mazePanel);
setVisible(true);
}
public void readMaze(String filename) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(filename));
n = Integer.parseInt(br.readLine());
maze = new char[n][n];
for (int i = 0; i < n; i++) {
String line = br.readLine();
for (int j = 0; j < n; j++) {
maze[i][j] = line.charAt(j);
if (maze[i][j] == 'S') {
startX = i;
startY = j;
} else if (maze[i][j] == 'G') {
goalX = i;
goalY = j;
}
}
}
br.close();
}
private boolean solveMaze(int x, int y) {
// Debug information
System.out.println("Visiting: (" + x + ", " + y + ")");
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
System.out.println("Out of bounds or already visited or wall at: (" + x + ", " + y + ")");
return false;
}
if (x == goalX && y == goalY) {
maze[x][y] = 'G';
mazePanel.repaint();
return true;
}
visited[x][y] = true;
maze[x][y] = '*';
mazePanel.repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Try to move in all directions
if (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {
return true;
}
// Backtrack
maze[x][y] = '.';
mazePanel.repaint();
visited[x][y] = false;
return false;
}
public void solveMaze() {
if (solveMaze(startX, startY)) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
private boolean solveMaze(int x, int y) {
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
return false;
}
if (x == goalX && y == goalY) {
maze[x][y] = 'G';
mazePanel.repaint();
return true;
}
visited[x][y] = true;
maze[x][y] = '*';
mazePanel.repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
if (this.solveMaze(x + 1, y) || this.solveMaze(x - 1, y) || this.solveMaze(x, y + 1) || this.solveMaze(x, y - 1)) {
return true;
}
maze[x][y] = '.';
mazePanel.repaint();
visited[x][y] = false;
return false;
}
public static void main(String[] args) throws IOException {
MazeSolver solver = new MazeSolver("maze.txt");
solver.solveMaze();
{
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(maze[i][j] + " ");
}
System.out.println();
}
}
}
public void displayMaze() {
}
}
class MazePanel extends JPanel {
private final char[][] maze;
private final int n;
public MazePanel(char[][] maze, int n) {
this.maze = maze;
this.n = n;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int cellSize = 40;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
switch (maze[i][j]) {
case '#' -> g.setColor(Color.BLACK);
case '.' -> g.setColor(Color.WHITE);
case 'S' -> g.setColor(Color.BLUE);
case 'G' -> g.setColor(Color.RED);
case '*' -> g.setColor(Color.GREEN);
default -> g.setColor(Color.WHITE);
}
g.fillRect(j * cellSize, i * cellSize, cellSize, cellSize);
g.setColor(Color.GRAY);
g.drawRect(j * cellSize, i * cellSize, cellSize, cellSize);
}
}
}
}
import java.awt.*;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
class MazeSolver extends JFrame {
private static int n;
private static char[][] maze;
private boolean[][] visited;
private int startX, startY, goalX, goalY;
private MazePanel mazePanel;
public MazeSolver(String filename) throws IOException {
readMaze(filename);
visited = new boolean[n][n];
setTitle("Maze Solver");
setSize(n * 40, n * 40);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
mazePanel = new MazePanel(maze, n);
add(mazePanel);
setVisible(true);
}
public void readMaze(String filename) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(filename));
n = Integer.parseInt(br.readLine());
maze = new char[n][n];
for (int i = 0; i < n; i++) {
String line = br.readLine();
for (int j = 0; j < n; j++) {
maze[i][j] = line.charAt(j);
if (maze[i][j] == 'S') {
startX = i;
startY = j;
} else if (maze[i][j] == 'G') {
goalX = i;
goalY = j;
}
}
}
br.close();
}
private boolean solveMaze(int x, int y) {
// Debug information
System.out.println("Visiting: (" + x + ", " + y + ")");
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
System.out.println("Out of bounds or already visited or wall at: (" + x + ", " + y + ")");
return false;
}
if (x == goalX && y == goalY) {
maze[x][y] = 'G';
mazePanel.repaint();
return true;
}
visited[x][y] = true;
maze[x][y] = '*';
mazePanel.repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Try to move in all directions
if (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {
return true;
}
// Backtrack
maze[x][y] = '.';
mazePanel.repaint();
visited[x][y] = false;
return false;
}
public void solveMaze() {
if (solveMaze(startX, startY)) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
private boolean solveMaze(int x, int y) {
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
return false;
}
if (x == goalX && y == goalY) {
maze[x][y] = 'G';
mazePanel.repaint();
return true;
}
visited[x][y] = true;
maze[x][y] = '*';
mazePanel.repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
if (this.solveMaze(x + 1, y) || this.solveMaze(x - 1, y) || this.solveMaze(x, y + 1) || this.solveMaze(x, y - 1)) {
return true;
}
maze[x][y] = '.';
mazePanel.repaint();
visited[x][y] = false;
return false;
}
public static void main(String[] args) throws IOException {
MazeSolver solver = new MazeSolver("maze.txt");
solver.solveMaze();
{
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(maze[i][j] + " ");
}
System.out.println();
}
}
}
public void displayMaze() {
}
}
class MazePanel extends JPanel {
private final char[][] maze;
private final int n;
public MazePanel(char[][] maze, int n) {
this.maze = maze;
this.n = n;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int cellSize = 40;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
switch (maze[i][j]) {
case '#' -> g.setColor(Color.BLACK);
case '.' -> g.setColor(Color.WHITE);
case 'S' -> g.setColor(Color.BLUE);
case 'G' -> g.setColor(Color.RED);
case '*' -> g.setColor(Color.GREEN);
default -> g.setColor(Color.WHITE);
}
g.fillRect(j * cellSize, i * cellSize, cellSize, cellSize);
g.setColor(Color.GRAY);
g.drawRect(j * cellSize, i * cellSize, cellSize, cellSize);
}
}
}
}
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
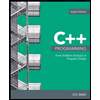
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
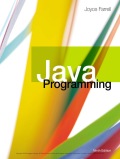
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
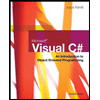
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
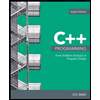
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
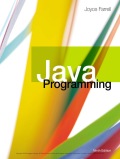
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
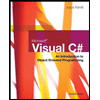
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
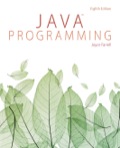
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage