Input Your output starts with Expected output starts with 17 10 Credits: 94 Dice total: 94 Dice total: 94 Dice total: 64 Dice total: 64 Dice total: 94 Dice total: 2 Dice total: 64 Dice total: 84 Credits: 10€ Dice total: 74 Credits: 9 Dice total: 2 Credits: 9
import java.util.Scanner;
public class LabProgram {
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
int credits;
int seed;
GVDie die1, die2;
die1 = new GVDie();
die2 = new GVDie();
// Read random seed to support testing (do not alter)
seed = scnr.nextInt();
die1.setSeed(seed);
// Read starting credits
credits = scnr.nextInt();
int rounds = 0;
while (credits > 0) {
// Step 1: Roll both dice
die1.roll();
die2.roll();
int total = die1.getValue() + die2.getValue();
if (total == 7 || total == 11) {
// Player wins one credit
credits++;
} else if (total == 2 || total == 3 || total == 12) {
// Player loses one credit
credits--;
} else {
// Set the goal for future rolls
int goal = total;
// Step 2: Continue rolling until goal or 7 is rolled
while (true) {
die1.roll();
die2.roll();
total = die1.getValue() + die2.getValue();
System.out.println("Dice total: " + total);
if (total == 7) {
// Player loses one credit
credits--;
break;
} else if (total == goal) {
// Player wins one credit
credits++;
break;
}
}
}
System.out.println("Credits: " + credits);
rounds++;
}
System.out.println("Rounds: " + rounds);
}
}
import java.util.*;
public class GVDie implements Comparable <GVDie> {
// Static members are shared across all instances of class GVDie
private static Random rand = new Random();
private int myValue;
// Set initial die value
public GVDie() {
myValue = rand.nextInt(6) + 1;
}
// Roll the die to get 1 - 6
public void roll () {
myValue = rand.nextInt(6) + 1;
}
// Return current die value
public int getValue() {
return myValue;
}
// Set the random number generator seed to support testing
public void setSeed(int seed) {
rand.setSeed(seed);
}
// Allows dice to be compared if necessary
public int compareTo(GVDie d) {
return getValue() - d.getValue();
}
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

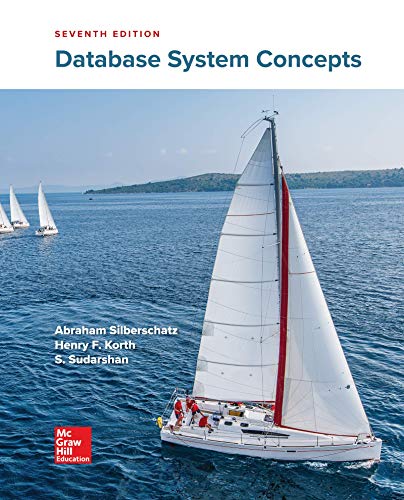
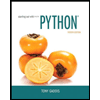
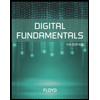
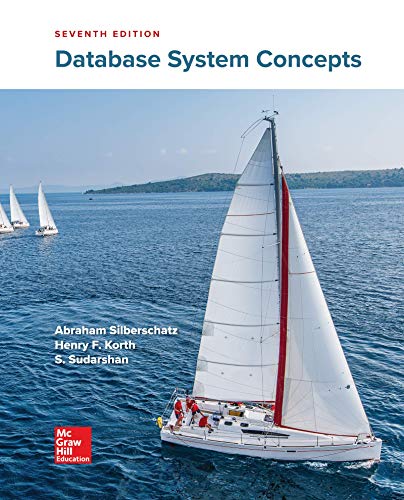
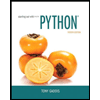
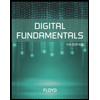
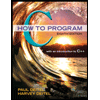
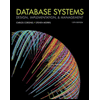
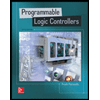