this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?
this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
using System;
class Program
{
publicstaticvoid Main(string[] args)
{
int number = 1;
while (number <= 88)
{
Console.WriteLine(number);
number = number + 2;
}
int[] randNo = newint[88];
Random r = new Random();
int i=0;
while (number <= 88)
{
randNo[i] = number;
number+=1;
i+=1;
}
for (i = 0; i < 3; i++)
{
Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]);
}
}
}
this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?
![### Educational Content on Random Number Generation in C#
Below is a C# program that demonstrates how to generate random numbers between a specific range, in this case, between 1 and 88.
#### Source Code
```csharp
using System;
class Program
{
public static void Main(string[] args)
{
int number = 1;
while (number <= 88)
{
Console.WriteLine(number);
number = number + 2;
}
int[] randNo = new int[88];
Random r = new Random();
int i = 0;
while (number <= 88)
{
randNo[i] = number;
number++;
i++;
}
for (i = 0; i < 3; i++)
{
Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]);
}
}
}
```
#### Program Explanation
1. **Initialization**: The program starts by importing the `System` namespace and defining the `Program` class with the `Main` method as the entry point.
2. **Odd Numbers Printing**:
- It declares an integer variable `number` and initializes it to 1.
- It uses a `while` loop to print all odd numbers between 1 and 88. This loop increments the `number` by 2 each iteration.
3. **Random Number Array**:
- It declares an array `randNo` to store integers, initializes an object `r` of the `Random` class, and sets the integer `i` to 0.
- Another `while` loop intends to iterate through numbers up to 88 and store them in the `randNo` array.
4. **Random Number Generation**:
- The final `for` loop iterates three times to print three random numbers from the range 1 to 88 using the `Next` method of the `Random` class.
#### Output
Here's what the program prints on execution. The right panel simulates the console output:
- Numbers between 1 and 88 incremented by 2.
- Random Numbers between 1 and 88 (three times).
```plaintext
1
3
5
...
85
87
Random Numbers between 1 and 88 are 0
Random Numbers between 1 and 88 are 0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a85ba4d-6f5c-41bf-97e5-182adb23b838%2F95139025-6ac1-4128-a921-46f4ab5a0973%2Fefrupl7_processed.png&w=3840&q=75)
Transcribed Image Text:### Educational Content on Random Number Generation in C#
Below is a C# program that demonstrates how to generate random numbers between a specific range, in this case, between 1 and 88.
#### Source Code
```csharp
using System;
class Program
{
public static void Main(string[] args)
{
int number = 1;
while (number <= 88)
{
Console.WriteLine(number);
number = number + 2;
}
int[] randNo = new int[88];
Random r = new Random();
int i = 0;
while (number <= 88)
{
randNo[i] = number;
number++;
i++;
}
for (i = 0; i < 3; i++)
{
Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]);
}
}
}
```
#### Program Explanation
1. **Initialization**: The program starts by importing the `System` namespace and defining the `Program` class with the `Main` method as the entry point.
2. **Odd Numbers Printing**:
- It declares an integer variable `number` and initializes it to 1.
- It uses a `while` loop to print all odd numbers between 1 and 88. This loop increments the `number` by 2 each iteration.
3. **Random Number Array**:
- It declares an array `randNo` to store integers, initializes an object `r` of the `Random` class, and sets the integer `i` to 0.
- Another `while` loop intends to iterate through numbers up to 88 and store them in the `randNo` array.
4. **Random Number Generation**:
- The final `for` loop iterates three times to print three random numbers from the range 1 to 88 using the `Next` method of the `Random` class.
#### Output
Here's what the program prints on execution. The right panel simulates the console output:
- Numbers between 1 and 88 incremented by 2.
- Random Numbers between 1 and 88 (three times).
```plaintext
1
3
5
...
85
87
Random Numbers between 1 and 88 are 0
Random Numbers between 1 and 88 are 0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
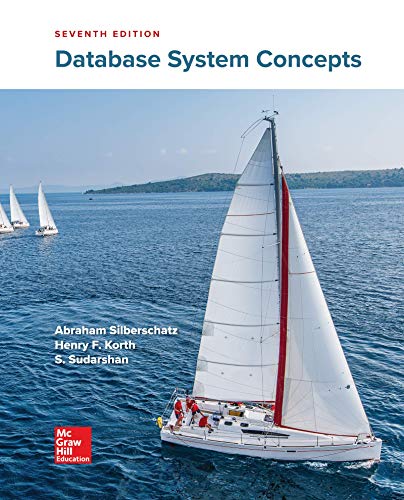
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
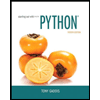
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
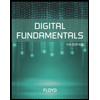
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
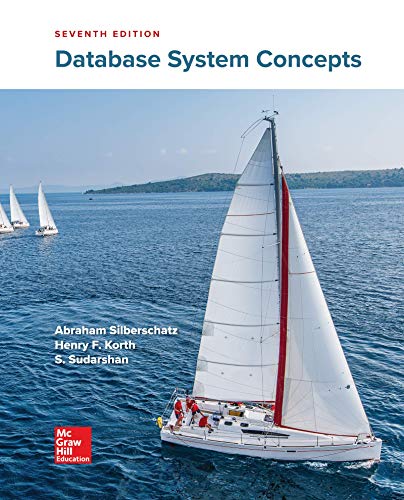
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
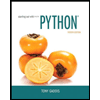
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
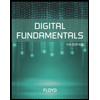
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
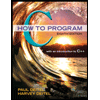
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
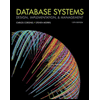
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
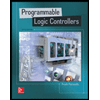
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education