import javax.swing.*; import java.awt.*; import java.util.List; import java.util.ArrayList; public class ControlPanel extends JFrame { private MiniMac miniMac; private JList memoryView; private JList programView; public ControlPanel() { miniMac = new MiniMac(); setupUI(); } private void setupUI() { // Setup UI components memoryView = new JList<>(); programView = new JList<>(); // Add action listeners to buttons JButton loadProgramButton = new JButton("Load Program"); loadProgramButton.addActionListener(e -> loadProgram()); // Layout components JPanel panel = new JPanel(new GridLayout(1, 2)); panel.add(new JScrollPane(memoryView)); panel.add(new JScrollPane(programView)); add(panel, BorderLayout.CENTER); add(loadProgramButton, BorderLayout.SOUTH); // Set frame properties setTitle("MiniMac Control Panel"); setSize(600, 400); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); } private void loadProgram() { // Sample method to load a program String program = "load 0 0\nload 1 10\nload 2 -1\nloop 10 { add 0 1 0; mul 0 2 0 }"; List instructions = MiniMacParser.parse(program); // Display program in program view DefaultListModel programModel = new DefaultListModel<>(); for (Instruction instruction : instructions) { programModel.addElement(instruction.toString()); } programView.setModel(programModel); // Execute program in MiniMac executeProgram(instructions); } private void executeProgram(List instructions) { for (Instruction instruction : instructions) { executeInstruction(instruction); } } private void executeInstruction(Instruction instruction) { String opcode = instruction.getOpcode(); int[] args = instruction.getArgs(); switch (opcode) { case "load": miniMac.load(args[0], args[1]); break; case "halt": miniMac.halt(); break; case "add": miniMac.add(args[0], args[1], args[2]); break; case "mul": miniMac.mul(args[0], args[1], args[2]); break; // Handle other instructions } // Update memory view updateMemoryView(); } private void updateMemoryView() { // Update memory view with MiniMac's memory contents DefaultListModel memoryModel = new DefaultListModel<>(); for (int i = 0; i < miniMac.getMemory().length; i++) { memoryModel.addElement("Memory[" + i + "]: " + miniMac.getMemory()[i]); } memoryView.setModel(memoryModel); } public static void main(String[] args) { SwingUtilities.invokeLater(ControlPanel::new); } } I added the other 3 files in the screenshots please check and help me to fix the code
import javax.swing.*;
import java.awt.*;
import java.util.List;
import java.util.ArrayList;
public class ControlPanel extends JFrame {
private MiniMac miniMac;
private JList<String> memoryView;
private JList<String> programView;
public ControlPanel() {
miniMac = new MiniMac();
setupUI();
}
private void setupUI() {
// Setup UI components
memoryView = new JList<>();
programView = new JList<>();
// Add action listeners to buttons
JButton loadProgramButton = new JButton("Load
loadProgramButton.addActionListener(e -> loadProgram());
// Layout components
JPanel panel = new JPanel(new GridLayout(1, 2));
panel.add(new JScrollPane(memoryView));
panel.add(new JScrollPane(programView));
add(panel, BorderLayout.CENTER);
add(loadProgramButton, BorderLayout.SOUTH);
// Set frame properties
setTitle("MiniMac Control Panel");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void loadProgram() {
// Sample method to load a program
String program = "load 0 0\nload 1 10\nload 2 -1\nloop 10 { add 0 1 0; mul 0 2 0 }";
List<Instruction> instructions = MiniMacParser.parse(program);
// Display program in program view
DefaultListModel<String> programModel = new DefaultListModel<>();
for (Instruction instruction : instructions) {
programModel.addElement(instruction.toString());
}
programView.setModel(programModel);
// Execute program in MiniMac
executeProgram(instructions);
}
private void executeProgram(List<Instruction> instructions) {
for (Instruction instruction : instructions) {
executeInstruction(instruction);
}
}
private void executeInstruction(Instruction instruction) {
String opcode = instruction.getOpcode();
int[] args = instruction.getArgs();
switch (opcode) {
case "load":
miniMac.load(args[0], args[1]);
break;
case "halt":
miniMac.halt();
break;
case "add":
miniMac.add(args[0], args[1], args[2]);
break;
case "mul":
miniMac.mul(args[0], args[1], args[2]);
break;
// Handle other instructions
}
// Update memory view
updateMemoryView();
}
private void updateMemoryView() {
// Update memory view with MiniMac's memory contents
DefaultListModel<String> memoryModel = new DefaultListModel<>();
for (int i = 0; i < miniMac.getMemory().length; i++) {
memoryModel.addElement("Memory[" + i + "]: " + miniMac.getMemory()[i]);
}
memoryView.setModel(memoryModel);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(ControlPanel::new);
}
}
I added the other 3 files in the screenshots please check and help me to fix the code
![1v class Instruction {
2
3
4
5
6
7
8
9
0
1
12
3
4
5
6
7
8
19
0
1
2
13
4
5
6
7
1
}
4
private String opcode;
private int[] args;
public Instruction(String opcode, int[] args) {
this.opcode = opcode;
this.args = args;
}
public String getOpcode() {
return opcode;
}
public int[] getArgs() {
return args;
}
@Override
public String toString() {
}
StringBuilder sb = new StringBuilder();
1
2
B import java.util.List;
4
5 public class MiniMacParser {
sb.append(opcode);
for (int arg : args) {
}
sb.append(" "). append(arg);
}
return sb.toString();
import java.util.ArrayList;
import java.util. Iterator;
}
public static List<Instruction> parse(String program) {
List<Instruction> instructions = new ArrayList<>();
String[] lines = program.split("\n");
for (String line : lines) {
instructions.add(parseInstruction(line.trim( ) ) );
}
return instructions;
private static Instruction parseInstruction(String line) {
String[] tokens = line.split("\\s+");
String opcode = tokens [0];
int[] args = new int[tokens.length - 1];
for (int i = 1; i < tokens.length; i++) {
}
args[i - 1] = Integer.parseInt(tokens[i]);
return new Instruction (opcode, args);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F325f30ca-56bc-46bd-9932-3ddac22cee03%2F7638d35f-94fd-447d-b836-dd2b8baee01a%2Ffdp91eq_processed.png&w=3840&q=75)
![import java.util.Arrays;
✓ public class MiniMac {
private Integer[] memory;
V
private int ip; // Instruction pointer
public MiniMac() {
memory = new Integer[32]; // Initial memory size
ip = 0; // Instruction pointer starts at 0
Arrays.fill(memory, 0); // Initialize memory to 0
}
public void load(int location, int value) {
memory [location] value;
}
public void halt() {
}
public void add(int src1, int src2, int dest) {
memory [dest] memory[src1] + memory[src2];
}
// Implementation to halt execution
}
public void mul(int src1, int src2, int dest) {
memory [dest] = memory [src1 * memory[src2];
=
}
// Other operations like bgt, blt, loop...
public Integer [] getMemory() {
return memory;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F325f30ca-56bc-46bd-9932-3ddac22cee03%2F7638d35f-94fd-447d-b836-dd2b8baee01a%2Fffoft7h_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

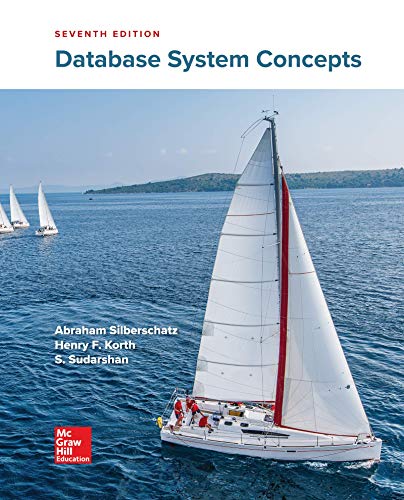
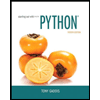
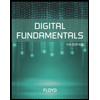
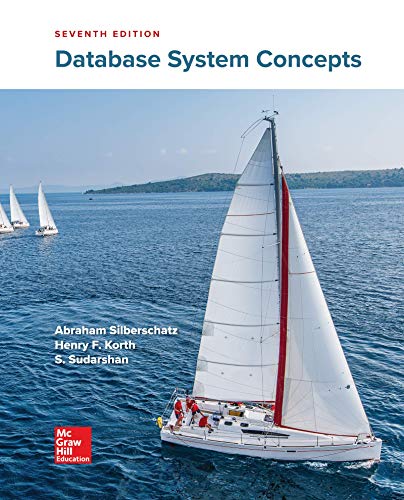
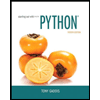
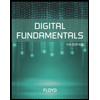
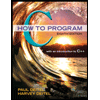
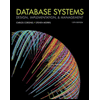
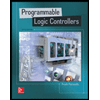