Draw an UML class diagram for the following code: import java.util.EmptyStackException; class Node { int data; Node next; public Node(int data) { this.data = data; this.next = null; } } class List { private Node head; private int size; public List() { head = null; size = 0; } public void add(int data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { Node current = head; while (current.next != null) { current = current.next; } current.next = newNode; } size++; } public int get(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException("Index out of range"); } Node current = head; for (int i = 0; i < index; i++) { current = current.next; } return current.data; } public void remove(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException("Index out of range"); } if (index == 0) { head = head.next; } else { Node prev = null; Node current = head; for (int i = 0; i < index; i++) { prev = current; current = current.next; } prev.next = current.next; } size--; } public boolean isEmpty() { return size == 0; } public int size() { return size; } public void removeAll() { head = null; size = 0; } public void printList() { Node current = head; while (current != null) { System.out.print(current.data + " "); current = current.next; } System.out.println(); } public int indexOf(int item) { Node current = head; int index = 0; while (current != null) { if (current.data == item) { return index; } current = current.next; index++; } return -1; } } class Stack { private List list; public Stack() { list = new List(); } public boolean empty() { return list.isEmpty(); } public int peek() throws EmptyStackException { if (empty()) { throw new EmptyStackException(); } return list.get(list.size() - 1); } public int pop() throws EmptyStackException { if (empty()) { throw new EmptyStackException(); } int poppedItem = list.get(list.size() - 1); list.remove(list.size() - 1); return poppedItem; } public void push(int item) { list.add(item); } public int search(int item) { return list.indexOf(item); } } public class Main { public static void main(String[] args) { Stack stack = new Stack(); if (stack.empty()) { System.out.println("The stack is empty"); } for (int i = 0; i < 5; i++) { stack.push(i); } System.out.println("Element at the top: " + stack.peek()); while (!stack.empty()) { System.out.print(stack.pop() + " "); } System.out.println(); List list = new List(); list.add(10); list.add(20); list.add(30); list.add(40); list.add(50); System.out.println("Elements after adding: "); list.printList(); System.out.println("Element at index 2: " + list.get(2)); list.remove(1); System.out.println("Elements after removing: "); list.printList(); System.out.println("Is the list empty? " + list.isEmpty()); System.out.println("Size of the list: " + list.size()); list.removeAll(); System.out.println("Is the list empty after removeAll? " + list.isEmpty()); } }
Draw an UML class diagram for the following code:
import java.util.EmptyStackException;
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
class List {
private Node head;
private int size;
public List() {
head = null;
size = 0;
}
public void add(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
size++;
}
public int get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of range");
}
Node current = head;
for (int i = 0; i < index; i++) {
current = current.next;
}
return current.data;
}
public void remove(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of range");
}
if (index == 0) {
head = head.next;
} else {
Node prev = null;
Node current = head;
for (int i = 0; i < index; i++) {
prev = current;
current = current.next;
}
prev.next = current.next;
}
size--;
}
public boolean isEmpty() {
return size == 0;
}
public int size() {
return size;
}
public void removeAll() {
head = null;
size = 0;
}
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
public int indexOf(int item) {
Node current = head;
int index = 0;
while (current != null) {
if (current.data == item) {
return index;
}
current = current.next;
index++;
}
return -1;
}
}
class Stack {
private List list;
public Stack() {
list = new List();
}
public boolean empty() {
return list.isEmpty();
}
public int peek() throws EmptyStackException {
if (empty()) {
throw new EmptyStackException();
}
return list.get(list.size() - 1);
}
public int pop() throws EmptyStackException {
if (empty()) {
throw new EmptyStackException();
}
int poppedItem = list.get(list.size() - 1);
list.remove(list.size() - 1);
return poppedItem;
}
public void push(int item) {
list.add(item);
}
public int search(int item) {
return list.indexOf(item);
}
}
public class Main {
public static void main(String[] args) {
Stack stack = new Stack();
if (stack.empty()) {
System.out.println("The stack is empty");
}
for (int i = 0; i < 5; i++) {
stack.push(i);
}
System.out.println("Element at the top: " + stack.peek());
while (!stack.empty()) {
System.out.print(stack.pop() + " ");
}
System.out.println();
List list = new List();
list.add(10);
list.add(20);
list.add(30);
list.add(40);
list.add(50);
System.out.println("Elements after adding: ");
list.printList();
System.out.println("Element at index 2: " + list.get(2));
list.remove(1);
System.out.println("Elements after removing: ");
list.printList();
System.out.println("Is the list empty? " + list.isEmpty());
System.out.println("Size of the list: " + list.size());
list.removeAll();
System.out.println("Is the list empty after removeAll? " + list.isEmpty());
}
}

Step by step
Solved in 4 steps with 1 images

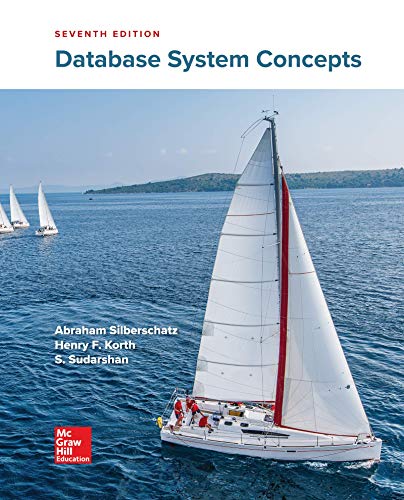
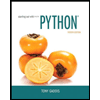
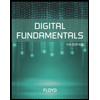
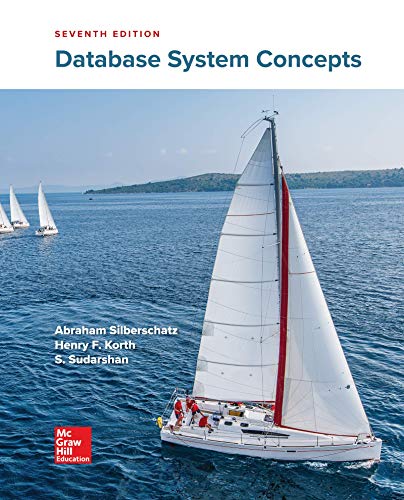
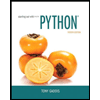
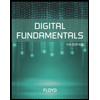
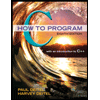
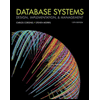
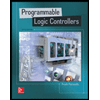