. Complete the function evaluate_postfix(String exp): Input: "10 23 - 5 15 + +" Retur: 7 Node.java public class Node { Object info; Node next; Node(Object info, Node next){ this.info=info; this.next=next; } } // "((1+2)" // ['(','(','1','+','2',')'] // stack : ')' -> '2' -> '1' -> '(' -> '(' -> null Stack.java public class Stack { private Node top; public Stack() { top = null; } public boolean isEmpty() { return (top == null); } public void push(Object newItem) { top = new Node(newItem, top); } public Object pop() { if (isEmpty()) { System.out.println( "Trying to pop when stack is empty"); return null; } else { Node temp = top; top = top.next; return temp.info; } } void popAll() { top = null; } public Object peek() { if (isEmpty()) { System.out.println( "Trying to peek when stack is empty"); return null; } else { return top.info; } } } Runner.java public class Runner { public static void main(String[] args) { String expression1 = "((1+2)))))+(2+2))(1/2)"; boolean res = isBalanced(expression1); System.out.println(res); } public static boolean isBalanced(String exp) { System.out.println("The string expression is " + exp); Stack st = new Stack(); char[] exp_char = exp.toCharArray(); System.out.println("The char array expression is "); for (char c : exp_char) { // if the exp_char[i] == '(' // push it on stack if (c == '(') st.push(c); // if the exp_char[i] == ')' // check if stack is empty // if stack empty: return false // else: pop from stack else if (c == ')') { if (st.isEmpty()) return false; st.pop(); } } // after loop check if stack is null // if stack is null return True; //else return false return st.isEmpty(); } }
. Complete the function evaluate_postfix(String exp): Input: "10 23 - 5 15 + +" Retur: 7 Node.java public class Node { Object info; Node next; Node(Object info, Node next){ this.info=info; this.next=next; } } // "((1+2)" // ['(','(','1','+','2',')'] // stack : ')' -> '2' -> '1' -> '(' -> '(' -> null Stack.java public class Stack { private Node top; public Stack() { top = null; } public boolean isEmpty() { return (top == null); } public void push(Object newItem) { top = new Node(newItem, top); } public Object pop() { if (isEmpty()) { System.out.println( "Trying to pop when stack is empty"); return null; } else { Node temp = top; top = top.next; return temp.info; } } void popAll() { top = null; } public Object peek() { if (isEmpty()) { System.out.println( "Trying to peek when stack is empty"); return null; } else { return top.info; } } } Runner.java public class Runner { public static void main(String[] args) { String expression1 = "((1+2)))))+(2+2))(1/2)"; boolean res = isBalanced(expression1); System.out.println(res); } public static boolean isBalanced(String exp) { System.out.println("The string expression is " + exp); Stack st = new Stack(); char[] exp_char = exp.toCharArray(); System.out.println("The char array expression is "); for (char c : exp_char) { // if the exp_char[i] == '(' // push it on stack if (c == '(') st.push(c); // if the exp_char[i] == ')' // check if stack is empty // if stack empty: return false // else: pop from stack else if (c == ')') { if (st.isEmpty()) return false; st.pop(); } } // after loop check if stack is null // if stack is null return True; //else return false return st.isEmpty(); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
1. Complete the function evaluate_postfix(String exp):
Input: "10 23 - 5 15 + +"
Retur: 7
Node.java
public class Node {
Object info;
Node next;
Node(Object info, Node next){
this.info=info;
this.next=next;
}
}
// "((1+2)"
// ['(','(','1','+','2',')']
// stack : ')' -> '2' -> '1' -> '(' -> '(' -> null
Object info;
Node next;
Node(Object info, Node next){
this.info=info;
this.next=next;
}
}
// "((1+2)"
// ['(','(','1','+','2',')']
// stack : ')' -> '2' -> '1' -> '(' -> '(' -> null
Stack.java
public class Stack {
private Node top;
public Stack() {
top = null;
}
public boolean isEmpty() {
return (top == null);
}
public void push(Object newItem) {
top = new Node(newItem, top);
}
public Object pop() {
if (isEmpty()) {
System.out.println(
"Trying to pop when stack is empty");
return null;
} else {
Node temp = top;
top = top.next;
return temp.info;
}
}
void popAll() {
top = null;
}
public Object peek() {
if (isEmpty()) {
System.out.println(
"Trying to peek when stack is empty");
return null;
} else {
return top.info;
}
}
}
private Node top;
public Stack() {
top = null;
}
public boolean isEmpty() {
return (top == null);
}
public void push(Object newItem) {
top = new Node(newItem, top);
}
public Object pop() {
if (isEmpty()) {
System.out.println(
"Trying to pop when stack is empty");
return null;
} else {
Node temp = top;
top = top.next;
return temp.info;
}
}
void popAll() {
top = null;
}
public Object peek() {
if (isEmpty()) {
System.out.println(
"Trying to peek when stack is empty");
return null;
} else {
return top.info;
}
}
}
Runner.java
public class Runner {
public static void main(String[] args) {
String expression1 = "((1+2)))))+(2+2))(1/2)";
boolean res = isBalanced(expression1);
System.out.println(res);
}
public static boolean isBalanced(String exp) {
System.out.println("The string expression is " + exp);
Stack st = new Stack();
char[] exp_char = exp.toCharArray();
System.out.println("The char array expression is ");
for (char c : exp_char) {
// if the exp_char[i] == '('
// push it on stack
if (c == '(')
st.push(c);
// if the exp_char[i] == ')'
// check if stack is empty
// if stack empty: return false
// else: pop from stack
else if (c == ')') {
if (st.isEmpty())
return false;
st.pop();
}
}
// after loop check if stack is null
// if stack is null return True;
//else return false
return st.isEmpty();
}
}
public static void main(String[] args) {
String expression1 = "((1+2)))))+(2+2))(1/2)";
boolean res = isBalanced(expression1);
System.out.println(res);
}
public static boolean isBalanced(String exp) {
System.out.println("The string expression is " + exp);
Stack st = new Stack();
char[] exp_char = exp.toCharArray();
System.out.println("The char array expression is ");
for (char c : exp_char) {
// if the exp_char[i] == '('
// push it on stack
if (c == '(')
st.push(c);
// if the exp_char[i] == ')'
// check if stack is empty
// if stack empty: return false
// else: pop from stack
else if (c == ')') {
if (st.isEmpty())
return false;
st.pop();
}
}
// after loop check if stack is null
// if stack is null return True;
//else return false
return st.isEmpty();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
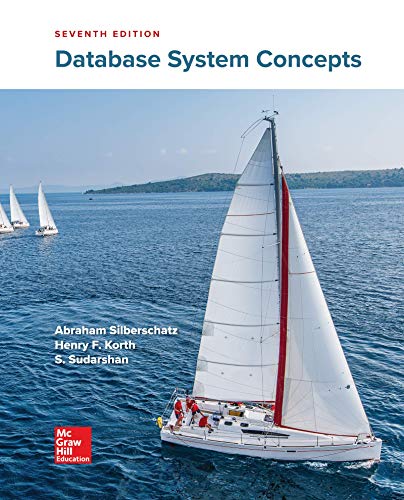
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
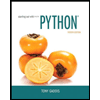
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
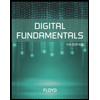
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
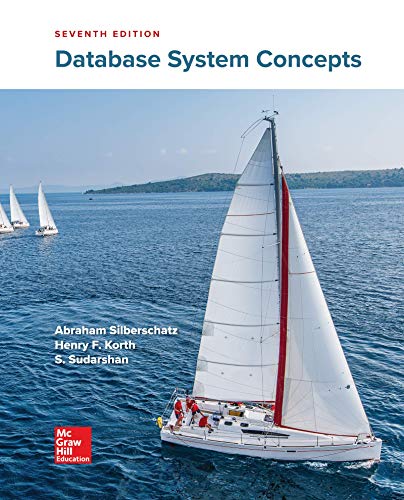
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
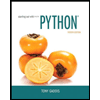
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
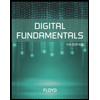
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
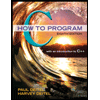
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
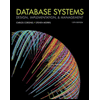
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
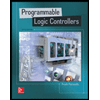
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education