class TenNums { private: int *p; public: TenNums() { p = new int[10]; for (
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
class TenNums {
private:
int *p;
public:
TenNums() {
p = new int[10];
for (int i = 0; i < 10; i++) p[i] = i;
}
void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }
};
int main() {
TenNums a;
a.display();
TenNums b = a;
b.display();
return 0;
}
Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method?
TenNums TenNums::operator+(const TenNums& b) {
TenNums o;
for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i];
return o;
}

Step by step
Solved in 2 steps

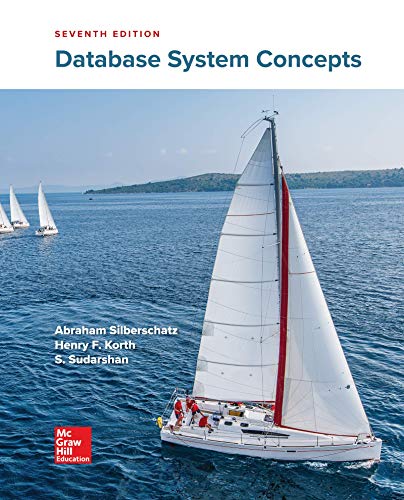
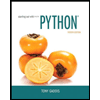
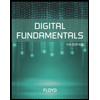
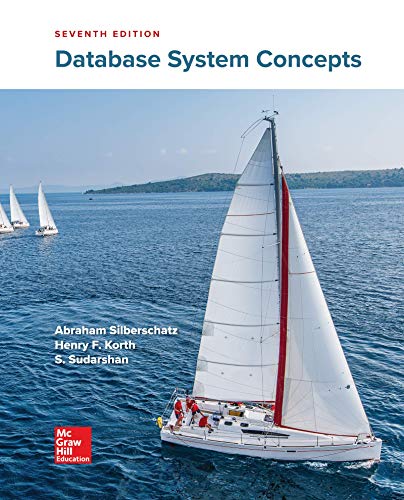
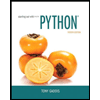
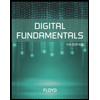
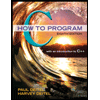
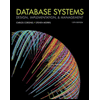
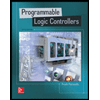