Computer Science c++ help comment code please. Complex has multiple parameters to overload i think. class Complex { public: Complex(); //There are no parameters because... Complex(double); //There is one parameter because... Complex(double, double);//There are two parameters because... double get_Real(); void set_Real(double); double get_Imaginary(); void set_Imaginary(double); friend ostream& operator << (ostream& out, Complex& r); //explain friend istream& operator >> (istream& in, Complex& r);//explain private: double real; double imaginary; }; ostream& operator << (ostream& out, Complex& r) // { double a = r.get_Real(); double b = r.get_Imaginary(); if (a != 0)out << a; if (b < 0) out << "-" << abs(b) << "i"; if (b > 0 && b != 1) out << "+" << b << "i"; if (b == 0) out << "0"; if (b == 1 && a != 0) out << "+" << "i"; if (b == 1 && a == 0) out << "i"; return out; } istream& operator >> (istream& in, Complex& r) // { double a, b; char plus, i; in >> a; in >> plus; in >> b; in >> i; if (plus != '+' && plus != '-') { cout << "No plus or minus found " << endl; } if (i != 'i') { cout << "no i found " << endl; } r.set_Real(a); r.set_Imaginary(b); return in; }
Computer Science
c++ help comment code please.
Complex has multiple parameters to overload i think.
class Complex
{
public:
Complex(); //There are no parameters because...
Complex(double); //There is one parameter because...
Complex(double, double);//There are two parameters because...
double get_Real();
void set_Real(double);
double get_Imaginary();
void set_Imaginary(double);
friend ostream& operator << (ostream& out, Complex& r); //explain
friend istream& operator >> (istream& in, Complex& r);//explain
private:
double real;
double imaginary;
};
ostream& operator << (ostream& out, Complex& r) //
{
double a = r.get_Real();
double b = r.get_Imaginary();
if (a != 0)out << a;
if (b < 0) out << "-" << abs(b) << "i";
if (b > 0 && b != 1) out << "+" << b << "i";
if (b == 0) out << "0";
if (b == 1 && a != 0) out << "+" << "i";
if (b == 1 && a == 0) out << "i";
return out;
}
istream& operator >> (istream& in, Complex& r) //
{
double a, b;
char plus, i;
in >> a;
in >> plus;
in >> b;
in >> i;
if (plus != '+' && plus != '-')
{
cout << "No plus or minus found " << endl;
}
if (i != 'i')
{
cout << "no i found " << endl;
}
r.set_Real(a);
r.set_Imaginary(b);
return in;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

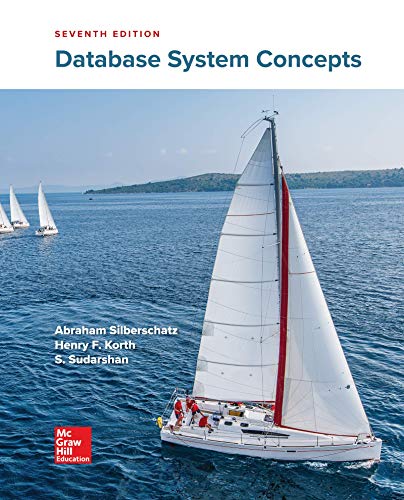
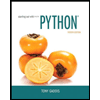
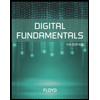
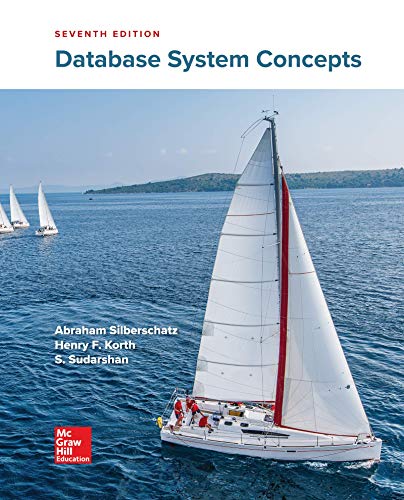
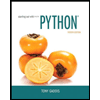
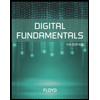
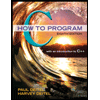
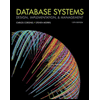
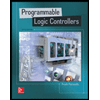