In C++ class film { public: void setFilmDirector(string s); //sets the filmTitle to s void setFilmYear(string year); //sets the private member filmYear to year void setFilmProdCost(double cost); //sets the private member filmProdCost to cost void setFilmGenre (string type); //sets the private member filmGenre to type void printAllFilmInfo() const; //prints the filmDirector, filmYear, the filmProdCost and the filmGenre string geFilmGenre() const; //returns the filmGenre string getFilmYear() const; //returns the filmYear double getFilmProdCost() const; //returns the filmProdCost void updateFilmProdCost(double cost); //adds cost to the filmProdCost, so that the filmProdCost now has it original value plus the //parameter sent to this function private: string filmDirector; string filmYear; double filmProdCost; string filmGenre; }; Assume the following in your main program (the constructors above have been written and added): film Joker(“Todd Phillips”, “2019”,43550698, “drama”); Write the code in the main program that will print out the information about Joker. Write the code in the main program that will add 12 Million to the filmProdCost for Joker.
In C++
class film
{
public:
void setFilmDirector(string s);
//sets the filmTitle to s
void setFilmYear(string year);
//sets the private member filmYear to year
void setFilmProdCost(double cost);
//sets the private member filmProdCost to cost
void setFilmGenre (string type);
//sets the private member filmGenre to type
void printAllFilmInfo() const;
//prints the filmDirector, filmYear, the filmProdCost and the filmGenre
string geFilmGenre() const;
//returns the filmGenre
string getFilmYear() const;
//returns the filmYear
double getFilmProdCost() const;
//returns the filmProdCost
void updateFilmProdCost(double cost);
//adds cost to the filmProdCost, so that the filmProdCost now has it original value plus the //parameter sent to this function
private:
string filmDirector;
string filmYear;
double filmProdCost;
string filmGenre;
};
Assume the following in your main program (the constructors above have been written and added):
film Joker(“Todd Phillips”, “2019”,43550698, “drama”);
- Write the code in the main program that will print out the information about Joker.
- Write the code in the main program that will add 12 Million to the filmProdCost for Joker.

Step by step
Solved in 2 steps

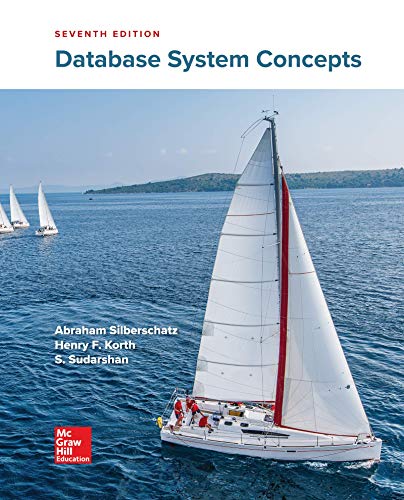
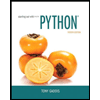
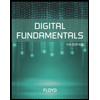
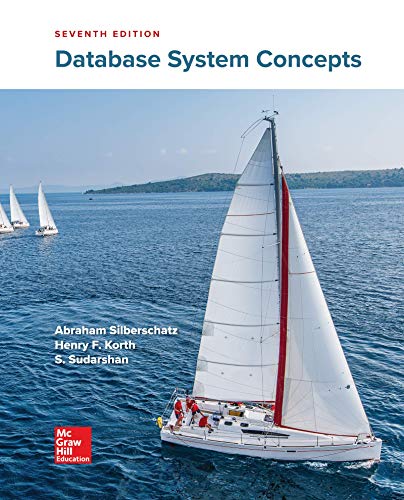
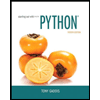
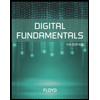
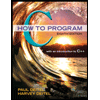
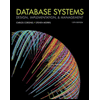
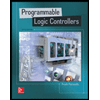