when printing Weigh no value shows up code public class HealthProfile { private String _FirstName; private String _LastName; private int _BirthYear; private double _Height; private double _Weigth; private int _CurrentYear; public HealthProf
C# problem
when printing Weigh no value shows up
code
public class HealthProfile
{
private String _FirstName;
private String _LastName;
private int _BirthYear;
private double _Height;
private double _Weigth;
private int _CurrentYear;
public HealthProfile(string firstName, string lastName, int birthYear, double height, double weigth, int currentYear)
{
_FirstName = firstName;
_LastName = lastName;
_BirthYear = birthYear;
_Height = height;
_Weigth = weight;
_CurrentYear = currentYear;
}
public string firstName { get; set; }
public string lastName { get; set; }
public int birthYear { get; set; }
public int height { get; set; }
public double weight { get; set; }
public int currentYear { get; set; }
public int Person_Age
{
get { return _CurrentYear - _BirthYear; }
}
public double Height
{
get { return _Height; }
}
public double Weight
{
get { return Weight; }
}
public int Max_Heart_Rate
{
get { return 220 - Person_Age; }
}
public double Min_Target_Heart_Rate
{
get { return Max_Heart_Rate * 0.65; }
}
public double Max_Target_Heart_Rate
{
get { return Max_Heart_Rate * 0.75; }
}
public double BMI
{
get {return (_Height / (Math.Pow(_Height, 2))) *703;}
}
public string BMIEvaluation()
{
if (BMI < 18.5)
return "Underweight";
else if (BMI < 25)
return "Normal";
else if (BMI < 30)
return "Overweight";
else
return "Obese";
}
public void DisplayPatientRecord()
{
Console.WriteLine($"|--------------------------------------------------------|");
Console.WriteLine($"| Patient Health Record |");
Console.WriteLine($"|--------------------------------------------------------|");
Console.WriteLine($"| {"Employee Name",-21} | {_LastName + ", " + _FirstName,-30} |");
Console.WriteLine($"| {"Patient Birth Year",-21} | {_BirthYear,30:D} |");
Console.WriteLine($"| {"Patient Age:",-21} | {Person_Age,30:D} |");
Console.WriteLine($"| {"Height",-21} | {_Height,30:F} |");
Console.WriteLine($"| {"Weight",-21} | {_Weigth,30:F} |");
Console.WriteLine($"| {"Maximum Heart Rate",-21} | {Max_Heart_Rate,30:F} |");
Console.WriteLine($"| {"Target Heart Rate",-21} | {(Min_Target_Heart_Rate + " - " + Max_Target_Heart_Rate),31:F}|");
Console.WriteLine($"| {"BMI",-21} | {BMI,30:F} |");
Console.WriteLine($"| {"BMI Text Value",-21} | {BMIEvaluation(),30} |");
Console.WriteLine($"|--------------------------------------------------------|");
}
}
}
main
static void Main(string[] args)
{
HealthProfile heartRate = new HealthProfile("Jessie","May",1977, 66.5 ,165.5, 2021 );
heartRate.DisplayPatientRecord();
}


Step by step
Solved in 3 steps with 1 images

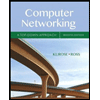
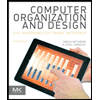
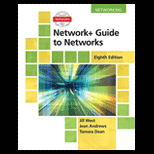
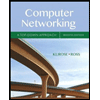
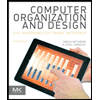
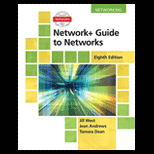
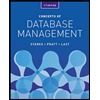
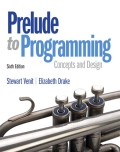
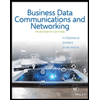