public class Student { private String name; private String major; privatedoublegpa; privateinthoursCompleted; /**Constructor * @param name * The student's name * @param major * The student's major */ public Student(String name, String major) { this.name = name; this.major = major; this.gpa = 0.0; hoursCompleted = 0; } /**Constructor * @param name * The student's name * @param major * The student's major * @param gpa * The student's cumulative gpa * @param hoursCompleted * Number of credit hours the student has completed */ public Student(String name, String major, doublegpa, inthoursCompleted) { this.name = name; this.major = major; this.gpa = gpa; this.hoursCompleted = hoursCompleted; } /** * @return The student's major. */ public String getMajor() { returnmajor; } /** * @param major The major to set. */ publicvoid setMajor(String major) { this.major = major; } /** * @return The student's name. */ public String getName() { returnname; } /** * @param name The name to set. */ publicvoid setName(String name) { this.name = name; } /** * @return The student's gpa. */ publicdouble getGpa() { returngpa; } /** * @return The number of credit hours the student has completed. */ publicint getHoursCompleted() { returnhoursCompleted; } /** * @return The student's class (Freshman, Sophomore, Junior, Senior) */ publicString getClassLevel() { String classLevel; if (hoursCompleted < 30) { classLevel = "Freshman"; } elseif (hoursCompleted < 60) { classLevel = "Sophomore"; } elseif (hoursCompleted < 90) { classLevel = "Junior"; } else { classLevel = "Senior"; } returnclassLevel; } /**Update student's information to reflect completion of a semester's work * @param semHours * Number of credit hours the student has just completed * @param semGPA * GPA for the semester just completed */ publicvoid updateStudent(intsemHours, doublesemGPA) { intoldHours = hoursCompleted; hoursCompleted = oldHours + semHours; gpa = (oldHours *gpa + semHours * semGPA) / hoursCompleted; } /** Print the student's name and class */ publicvoid printClassLevel() { System.out.println(name + " is a " + getClassLevel()); } /**Prints the information about a student in a nice format with * appropriate labeling */ publicvoid print() { DecimalFormat fmt = new DecimalFormat("0.000"); System.out.println(name + " is a " + getClassLevel() + " " + major); System.out.print("who has completed " + hoursCompleted + " hours "); System.out.println("with a " + fmt.format(gpa) + " gpa."); } } public class StudentPlay { /** * @param args */ publicstaticvoid main(String[] args) { Student student1 = new Student("Reagan Wilson", "Music Education", 3.8, 16); Student student2 = new Student("Hillary Adams", "Marketing"); student1.printClassLevel(); student2.setMajor("Agriculture"); student1.print(); System.out.print(student1); } } How would I fix main method so it is not printing out the memory location. I cannot change the code in the Student class.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
public class Student
{
private String name;
private String major;
privatedoublegpa;
privateinthoursCompleted;
/**Constructor
* @param name
* The student's name
* @param major
* The student's major
*/
public Student(String name, String major)
{
this.name = name;
this.major = major;
this.gpa = 0.0;
hoursCompleted = 0;
}
/**Constructor
* @param name
* The student's name
* @param major
* The student's major
* @param gpa
* The student's cumulative gpa
* @param hoursCompleted
* Number of credit hours the student has completed
*/
public Student(String name, String major, doublegpa, inthoursCompleted)
{
this.name = name;
this.major = major;
this.gpa = gpa;
this.hoursCompleted = hoursCompleted;
}
/**
* @return The student's major.
*/
public String getMajor()
{
returnmajor;
}
/**
* @param major The major to set.
*/
publicvoid setMajor(String major)
{
this.major = major;
}
/**
* @return The student's name.
*/
public String getName()
{
returnname;
}
/**
* @param name The name to set.
*/
publicvoid setName(String name)
{
this.name = name;
}
/**
* @return The student's gpa.
*/
publicdouble getGpa()
{
returngpa;
}
/**
* @return The number of credit hours the student has completed.
*/
publicint getHoursCompleted()
{
returnhoursCompleted;
}
/**
* @return The student's class (Freshman, Sophomore, Junior, Senior)
*/
publicString getClassLevel()
{
String classLevel;
if (hoursCompleted < 30)
{
classLevel = "Freshman";
}
elseif (hoursCompleted < 60)
{
classLevel = "Sophomore";
}
elseif (hoursCompleted < 90)
{
classLevel = "Junior";
}
else
{
classLevel = "Senior";
}
returnclassLevel;
}
/**Update student's information to reflect completion of a semester's work
* @param semHours
* Number of credit hours the student has just completed
* @param semGPA
* GPA for the semester just completed
*/
publicvoid updateStudent(intsemHours, doublesemGPA)
{
intoldHours = hoursCompleted;
hoursCompleted = oldHours + semHours;
gpa = (oldHours *gpa + semHours * semGPA) / hoursCompleted;
}
/** Print the student's name and class */
publicvoid printClassLevel()
{
System.out.println(name + " is a " + getClassLevel());
}
/**Prints the information about a student in a nice format with
* appropriate labeling
*/
publicvoid print()
{
DecimalFormat fmt = new DecimalFormat("0.000");
System.out.println(name + " is a " + getClassLevel() + " " + major);
System.out.print("who has completed " + hoursCompleted + " hours ");
System.out.println("with a " + fmt.format(gpa) + " gpa.");
}
}
public class StudentPlay
{
/**
* @param args
*/
publicstaticvoid main(String[] args)
{
Student student1 = new Student("Reagan Wilson", "Music Education", 3.8, 16);
Student student2 = new Student("Hillary Adams", "Marketing");
student1.printClassLevel();
student2.setMajor("Agriculture");
student1.print();
System.out.print(student1);
}
}
How would I fix main method so it is not printing out the memory location. I cannot change the code in the Student class.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

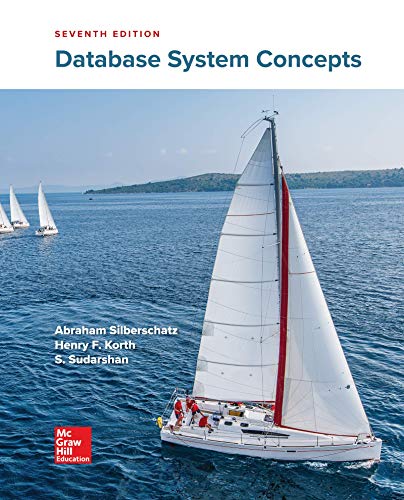
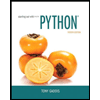
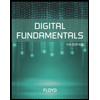
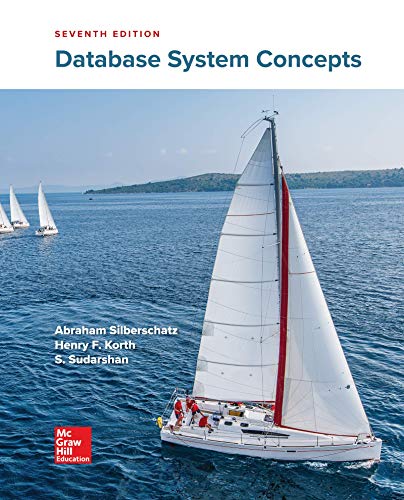
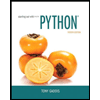
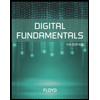
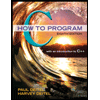
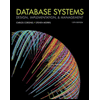
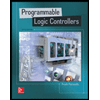