Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList(), that uses the printInfo() methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printInfo() method. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac. flower Rose 6 false white plant Mint 4 -1 the output is: Plant 1 Information: Plant name: Spirea Cost: 10 Plant 2 Information: Plant name: Hydrangea Cost: 30 Annual: false Color of flowers: lilac Plant 3 Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant 4 Information: Plant name: Mint Cost: 4
public class Plant {
protected String plantName;
protected String plantCost;
public void setPlantName(String userPlantName) {
plantName = userPlantName;
}
public String getPlantName() {
return plantName;
}
public void setPlantCost(String userPlantCost) {
plantCost = userPlantCost;
}
public String getPlantCost() {
return plantCost;
}
public void printInfo() {
System.out.println(" Plant name: " + plantName);
System.out.println(" Cost: " + plantCost);
}
}
public class Flower extends Plant {
private boolean isAnnual;
private String colorOfFlowers;
public void setPlantType(boolean userIsAnnual) {
isAnnual = userIsAnnual;
}
public boolean getPlantType(){
return isAnnual;
}
public void setColorOfFlowers(String userColorOfFlowers) {
colorOfFlowers = userColorOfFlowers;
}
public String getColorOfFlowers(){
return colorOfFlowers;
}
@Override
public void printInfo(){
System.out.println(" Plant name: " + plantName);
System.out.println(" Cost: " + plantCost);
System.out.println(" Annual: " + isAnnual);
System.out.println(" Color of flowers: " + colorOfFlowers);
}
}
![**Java Code: PlantArrayListExample**
This code snippet is an example of a Java program that aims to create an `ArrayList` to store objects representing plants or flowers. Below is the transcribed code with comments explaining the intended actions:
```java
import java.util.StringTokenizer;
public class PlantArrayListExample {
// TODO: Define a printArrayList method that prints an ArrayList of plant (or flower) objects
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String input;
// TODO: Declare an ArrayList called myGarden that can hold objects of type plant
// TODO: Declare variables - plantName, plantCost, flowerName, flowerCost, colorOfFlowers, isAnnual
input = scnr.next();
while (!input.equals("-1")) {
// TODO: Check if input is a plant or flower
// Store as a plant object or flower object
// Add to the ArrayList myGarden
input = scnr.next();
}
// TODO: Call the method printArrayList to print myGarden
}
}
```
**Explanation**
- **Import Statement (Line 1):** The code imports the `StringTokenizer` class from Java's utility package, although not used in this snippet, it might be planned for parsing strings efficiently.
- **Class Declaration (Line 3):** The class `PlantArrayListExample` is defined. It likely includes methods and data related to plant objects.
- **Main Method (Lines 5-19):**
- The main method begins by creating a `Scanner` object `scnr` to read user input.
- A `String` variable `input` is declared to receive input values.
- A placeholder is present to declare an `ArrayList` called `myGarden`. This list will potentially store plant or flower objects.
- Additional placeholder comments suggest the need to declare several variables for storing attributes like `plantName`, `plantCost`, `flowerName`, `flowerCost`, `colorOfFlowers`, and `isAnnual`.
- A loop is set to continue taking inputs until the user enters `-1`. Inside this loop, checks will be made to determine if the input corresponds to a plant or a flower, then store it in the `myGarden` ArrayList.
- A final comment hints at a method `printArrayList](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Fae9383bf-83e5-41aa-ab5e-c4df5309ea10%2Fc0v9tql_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

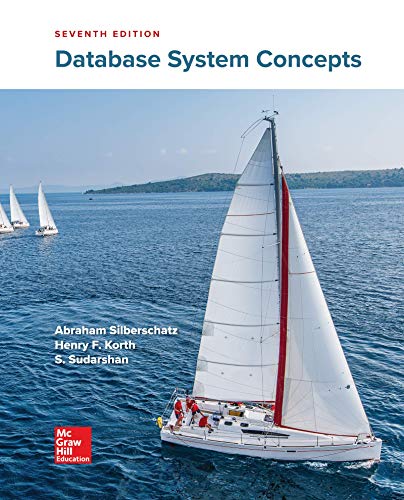
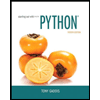
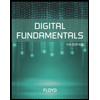
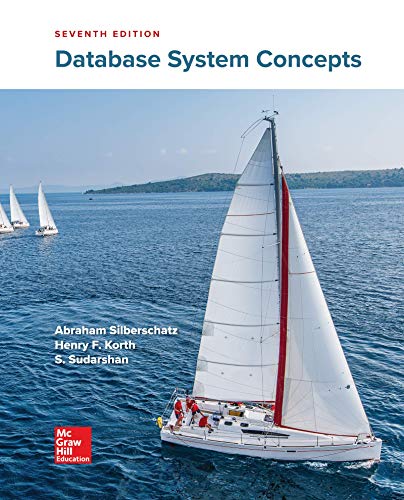
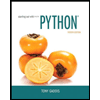
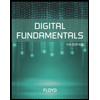
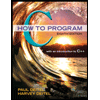
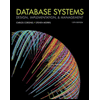
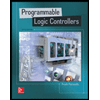