Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) • Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex: If the program has 4 students enrolled in the course, the output looks like: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4
Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) • Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex: If the program has 4 students enrolled in the course, the output looks like: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
public class LabProgram {
public static void main(String args[]) {
Course course = new Course();
String first; // first name
String last; // last name
double gpa; // grade point average
first = "Henry";
last = "Cabot";
gpa = 3.5;
course.addStudent(new Student(first, last, gpa)); // Add 1st student
first = "Brenda";
last = "Stern";
gpa = 2.0;
course.addStudent(new Student(first, last, gpa)); // Add 2nd student
first = "Jane";
last = "Flynn";
gpa = 3.9;
course.addStudent(new Student(first, last, gpa)); // Add 3rd student
first = "Lynda";
last = "Robison";
gpa = 3.2;
course.addStudent(new Student(first, last, gpa)); // Add 4th student
course.printRoster();
}
}
// Class representing a student
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average
// Student class constructor
public Student(String first, String last, double gpa) {
this.first = first; // first name
this.last = last; // last name
this.gpa = gpa; // grade point average
}
public double getGPA() {
return gpa;
}
public String getLast() {
return last;
}
// Returns a String representation of the Student object, with the GPA formatted to one decimal
public String toString() {
DecimalFormat fmt = new DecimalFormat("#.0");
return first + " " + last + " " + "(GPA: " + fmt.format(gpa) + ")";
}
}

Transcribed Image Text:**Objective**: Implement the `printRoster()` method in the `Course` class to display a list of all students enrolled in a course, along with the total number of students.
### Given Classes:
- **Class LabProgram**: Contains the main method for testing the program.
- **Class Course**: Represents a course containing an `ArrayList` of `Student` objects, which serves as the course roster. (Type your code in this section)
- **Class Student**: Represents a student with three fields: first name, last name, and GPA.
### Example:
If the program has 4 students enrolled, the output will be formatted as follows:
```
Henry Cabot (GPA: 3.5)
Brenda Stern (GPA: 2.0)
Jane Flynn (GPA: 3.9)
Lynda Robison (GPA: 3.2)
Students: 4
```

Transcribed Image Text:### Java Class: Course
This Java code snippet defines a class `Course`, which manages a collection of `Student` objects using an `ArrayList`. Below is a detailed explanation of the code structure:
```java
public class Course {
private ArrayList<Student> roster; // Collection of Student objects
public Course() {
roster = new ArrayList<Student>();
}
public void printRoster() {
/* Type your code here */
}
public void addStudent(Student s) {
roster.add(s);
}
}
```
#### Explanation:
1. **Class Declaration:**
- `public class Course`: This declares a public class named `Course`.
2. **Private Field:**
- `private ArrayList<Student> roster;`: This is a private field named `roster` that holds a collection of `Student` objects using `ArrayList`.
3. **Constructor:**
- `public Course()`: This is the constructor for the `Course` class. It initializes the `roster` as a new `ArrayList` of `Student`.
4. **Method - printRoster:**
- `public void printRoster()`: A method intended to print the roster of students. The method body is currently empty and marked with a comment `/* Type your code here */`, indicating a place where code for printing the roster can be added.
5. **Method - addStudent:**
- `public void addStudent(Student s)`: A method to add a `Student` object to the `roster`. It takes a parameter `s` of type `Student` and adds it to the `roster` list using the `add` method.
The `Course` class provides a basic template to manage students in a course by allowing students to be added and potentially printed. Students and additional functionality can be implemented by expanding the methods accordingly.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
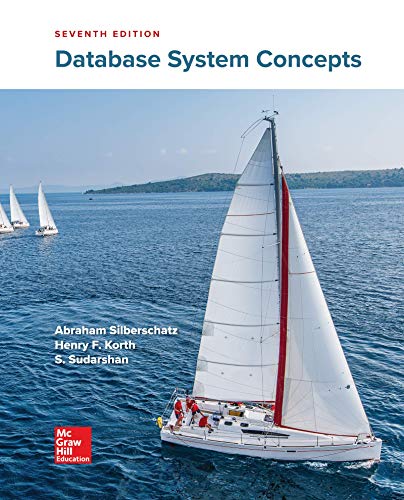
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
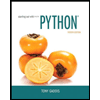
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
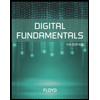
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
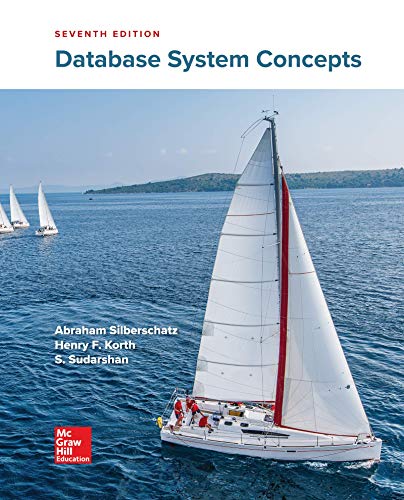
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
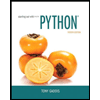
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
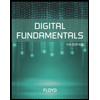
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
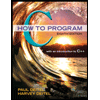
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
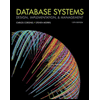
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
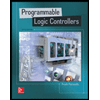
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education