class sequence { public: // TYPEDEFS and MEMBER SP2020 typedef Item value_type; typedef std::size_t size_type; static const size_type CAPACITY = 10; // CONSTRUCTOR sequence(); // MODIFICATION MEMBER FUNCTIONS void start(); void end(); void advance(); void move_back(); void add(const value_type& entry); void remove_current(); // CONSTANT MEMBER FUNCTIONS size_type size() const; bool is_item() const; value_type current() const; error: expected initializer before 'sequence' 125 | sequencevalue_type sequence ::current() const { | ^~~~~~~~
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
class sequence
{
public:
// TYPEDEFS and MEMBER SP2020
typedef Item value_type;
typedef std::size_t size_type;
static const size_type CAPACITY = 10;
// CONSTRUCTOR
sequence();
// MODIFICATION MEMBER FUNCTIONS
void start();
void end();
void advance();
void move_back();
void add(const value_type& entry);
void remove_current();
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is_item() const;
value_type current() const;
error: expected initializer before 'sequence'
125 | sequence<Item>value_type sequence ::current() const {
| ^~~~~~~~

Solution:
Given,
TYPEDEFS and MEMBER CONSTANTS typedef double value_type;
typedef
std::size_t
size_type;
static const
size_type
CAPACITY = 30;
CONSTRUCTOR
sequence (
{
used = 0;
current_index = CAPACITY:}
// MODIFICATION MEMBER FUNCTIONS
void start ();
void advance ();
void insert (const value_type & entry);
void attach (const value_type & entry); void remove_current ();
// CONSTANT MEMBER FUNCTIONS s
ize_type size ()
const
{
return used;
}
bool is_item ()
const;
value_type current ()
const;
private: value_type data[CAPACITY); // The array to store items size_type used; // How much of array is used size_type current_index; // Index of current item Write your main()
Step by step
Solved in 3 steps with 3 images

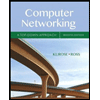
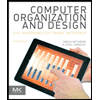
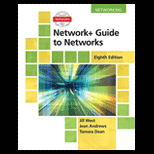
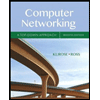
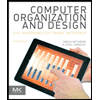
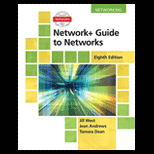
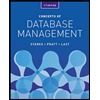
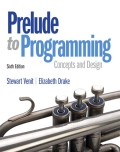
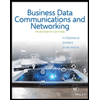