add member function Signature: Complex add(const Complex &addComplex) const Returns a new Complex object that is this object added by the specified object. Formula: (a + bi) + (c + di) = (a + c) + (b + d)i New Real: (a + c) New Imaginary: (b + d) subtract member function Signature: Complex subtract(const Complex &subtractComplex) const Returns a new Complex object that is this object subtracted by the specified object. Formula: (a + bi) - (c + di) = (a - c) + (b - d)i New Real: (a - c) New Imaginary: (b - d) multiply member function Signature: Complex multiply(const Complex &multiplyComplex) const Returns a new Complex object that is this object multiplied by the specified object. Formula: (a + bi) * (c + di) = (a*c - b*d) + (b*c + a*d)i New Real: (a*c - b*d) New Imaginary: (b*c + a*d) divide member function Signature: Complex divide(const Complex ÷Complex) const Returns a new Complex object that is this object divided by the specified object. Formula: (a + bi) / (c + di) = ((a*c + b*d)/(c*c + d*d)) + ((b*c - a*d)/(c*c + d*d))i New Real: ((a*c + b*d) / (c*c + d*d)) New Imaginary: ((b*c - a*d) / (c*c + d*d)) Be sure to have a program description at the top and in-line comments. Be clear with your comments and output to the user, so I can understand what the program is doing. Code to edit C++ CODE #include using namespace std; class Complex { double real,imaginary; public: Complex() { real=0; imaginary=0; } void set(double r,double i) { real=r; imaginary=i; } void print() { cout<<"("<
-
-
- add member function
- Signature: Complex add(const Complex &addComplex) const
- Returns a new Complex object that is this object added by the specified object.
- Formula: (a + bi) + (c + di) = (a + c) + (b + d)i
- New Real: (a + c)
- New Imaginary: (b + d)
- subtract member function
- Signature: Complex subtract(const Complex &subtractComplex) const
- Returns a new Complex object that is this object subtracted by the specified object.
- Formula: (a + bi) - (c + di) = (a - c) + (b - d)i
- New Real: (a - c)
- New Imaginary: (b - d)
- multiply member function
- Signature: Complex multiply(const Complex &multiplyComplex) const
- Returns a new Complex object that is this object multiplied by the specified object.
- Formula: (a + bi) * (c + di) = (a*c - b*d) + (b*c + a*d)i
- New Real: (a*c - b*d)
- New Imaginary: (b*c + a*d)
- divide member function
- Signature: Complex divide(const Complex ÷Complex) const
- Returns a new Complex object that is this object divided by the specified object.
- Formula: (a + bi) / (c + di) = ((a*c + b*d)/(c*c + d*d)) + ((b*c - a*d)/(c*c + d*d))i
- New Real: ((a*c + b*d) / (c*c + d*d))
- New Imaginary: ((b*c - a*d) / (c*c + d*d))
- Be sure to have a program description at the top and in-line comments.
- Be clear with your comments and output to the user, so I can understand what the program is doing.
- add member function
-
Code to edit
C++ CODE
#include<iostream>
using namespace std;
class Complex
{
double real,imaginary;
public:
Complex()
{
real=0;
imaginary=0;
}
void set(double r,double i)
{
real=r;
imaginary=i;
}
void print()
{
cout<<"("<<real<<"+("<<imaginary<<"i))";
}
double getReal()
{
return real;
}
double getImaginary()
{
return imaginary;
}
~Complex()
{
cout<<"Destrcutor\n";
}
};
int main()
{
Complex c1;
Complex c2;
Complex c3;
c2.set(3.3,-4.4);
c3.set(5.5,6.6);
cout<<"Test the constructor."<<endl;
cout<<"Complex number complex1 is: ";
c1.print();
cout<<"\n";
cout<<"Test the one set() function."<<endl;
cout<<"Complex number complex2 is: ";
c2.print();
cout<<"\n";
cout<<"Complex number complex3 is: ";
c3.print();
cout<<endl;
cout<<"Test the two get() functions."<<endl;
cout<<"Complex number complex3's real part is: "<<c3.getReal()<<endl;
cout<<"Complex number complex3's imaginary part is: "<<c3.getImaginary()<<endl;
return 0;
}


Step by step
Solved in 2 steps

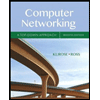
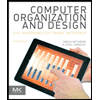
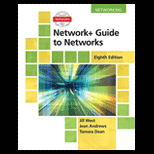
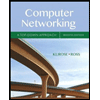
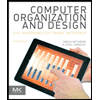
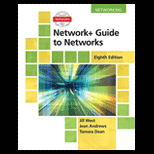
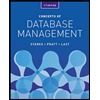
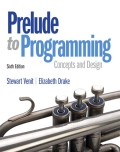
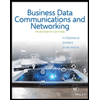