namespace CS3358_FA2022_A04_sequenceOfChar { template class sequence { public: // TYPEDEFS and MEMBER SP2020 typedef char value_type; typedef std::size_t size_type; static const size_type CAPACITY = 10; // CONSTRUCTOR sequence(); // MODIFICATION MEMBER FUNCTIONS void start(); void end(); void advance(); void move_back(); void add(const value_type& entry); void remove_current(); // CONSTANT MEMBER FUNCTIONS size_type size() const; bool is_item() const; value_type current() const; private: value_type data[CAPACITY]{}; size_type used; size_type current_index; }; } error: invalid use of template-name 'CS3358_FA2022_A04_sequenceOfChar::sequence' without an argument list 197 | sequence::size_type sequence::size() const { return used; } | ^~~~~~~~
namespace CS3358_FA2022_A04_sequenceOfChar
{
template <class Item>
class sequence
{
public:
// TYPEDEFS and MEMBER SP2020
typedef char value_type;
typedef std::size_t size_type;
static const size_type CAPACITY = 10;
// CONSTRUCTOR
sequence();
// MODIFICATION MEMBER FUNCTIONS
void start();
void end();
void advance();
void move_back();
void add(const value_type& entry);
void remove_current();
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is_item() const;
value_type current() const;
private:
value_type data[CAPACITY]{};
size_type used;
size_type current_index;
};
}
error: invalid use of template-name 'CS3358_FA2022_A04_sequenceOfChar::sequence' without an argument list
197 | sequence::size_type sequence::size() const { return used; }
| ^~~~~~~~

Below is the c++ program for above question. The code is explained in comments.
----------------------------------------------------------------------------------------------------------------------------------
#include<bits/stdc++.h>
using namespace std;
typedef double value_type;
typedef std::size_t size_type;
class sequence{
public:
static const size_type CAPACITY = 30;
// default constructor
sequence()
{
used=0;
current_index=CAPACITY;
}
// modification member function.
void start();
void advance();
void insert(const value_type& entry);
void attach(const value_type& entry);
void remove_current(const value_type& entry);
// constant member function
size_type size() const{return used;}
bool is_item(const value_type& entry) const;
// print member function to print the array
void print()
{
for(size_type i=0;i<size();i++)
{
cout<<data[i]<<" ";
}
cout<<endl;
}
value_type current() const;
private:
value_type data[CAPACITY]; // array to store items
size_type used; // how much array is used
size_type current_index; // index of current item
};
// at starting current index will be zero.
void sequence::start()
{
current_index=0;
}
// increase the current_index everytime when value is inserted.
void sequence::advance()
{
current_index++;
}
// insert the element at current_index;
void sequence::insert(const value_type& entry)
{
if(current_index==CAPACITY)
{
start();
data[current_index]=entry;
// increase the index.
advance();
// keep track of the number of array used.
used++;
}
else{
// increase the index after each insertion by calling advance
data[current_index]=entry;
advance();
used++;
}
}
// attach element at end of the array
void sequence::attach(const value_type& entry)
{
if(current_index==CAPACITY)
{
// keep track of the number of array used.
start();
data[current_index]=entry;
advance();
used++;
}
else{
// increase the index after each insertion.
data[current_index]=entry;
advance();
// keep track of the number of array used.
used++;
}
}
// removes current element in array
void sequence::remove_current(const value_type& entry)
{
size_type index;
for(size_type i=0;i<size();i++)
{
if(data[i]==entry)
{
index=i;
break;
}
}
for(size_type j=index;j<size()-1;j++)
{
data[j]=data[j+1];
}
// one element is removed so used will decrease
used--;
}
// checks if item is present in array or not.
bool sequence::is_item(const value_type& entry) const{
for(size_type i=0;i<size();i++)
{
if(data[i]==entry)
{
return true;
}
}
// if not present then return false.
return false;
}
// returns current item form array.
value_type sequence::current() const{
return data[current_index];
}
// driver code.
int main()
{
sequence seq;
seq.insert(5);
seq.insert(2);
seq.insert(200);
seq.insert(-3.0);
seq.insert(45);
seq.insert(-55.5);
seq.print();
seq.remove_current(-3.0);
seq.print();
}
Step by step
Solved in 2 steps with 1 images

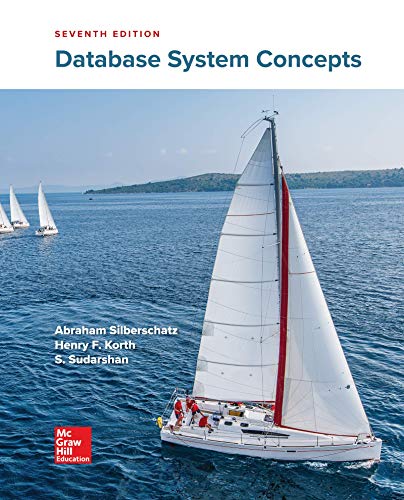
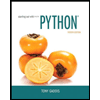
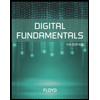
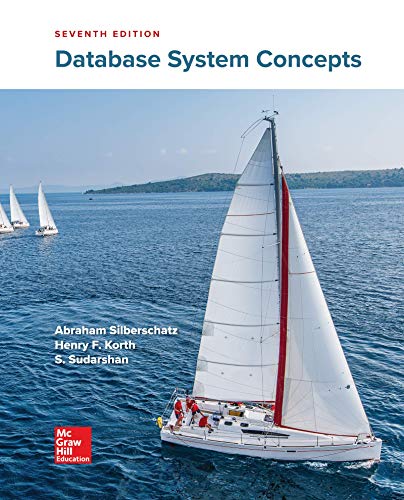
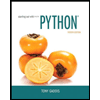
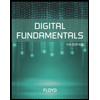
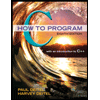
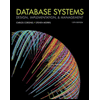
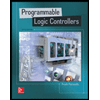