/ TYPEDEFS and MEMBER CONSTANTS for the sequence class: // typedef ____ value_type // sequence::value_type is the data type of the items in the sequence. // It may be any of the C++ built-in types (int, char, etc.), or a // class with a default constructor, an assignment operator, and a // copy constructor. // // typedef ____ size_type // sequence::size_type is the data type of any variable that keeps // track of how many items are in a sequence. // // static const size_type DEFAULT_CAPACITY = _____ // sequence::DEFAULT_CAPACITY is the default initial capacity of a // sequence that is created by the default constructor. // // CONSTRUCTOR for the sequence class: // sequence(size_type initial_capacity = DEFAULT_CAPACITY) // Pre: initial_capacity > 0 // Post: The sequence has been initialized as an empty sequence. // The insert/attach functions will work efficiently (without // allocating new memory) until this capacity is reached. // Note: If Pre is not met, initial_capacity will be adjusted to 1. // // MODIFICATION MEMBER FUNCTIONS for the sequence class: // void resize(size_type new_capacity) // Pre: new_capacity > 0 // Post: The sequence's current capacity is changed to new_capacity // (but not less that the number of items already on the sequence). // The insert/attach functions will work efficiently (without // allocating new memory) until this new capacity is reached. // Note: If new_capacity is less than used, it will be made equal to // to used (in order to preserve existing data). Thereafter, if Pre // is not met, new_capacity will be adjusted to 1. // // void start() // Pre: none // Post: The first item on the sequence becomes the current item // (but if the sequence is empty, then there is no current item). // // void advance() // Pre: is_item returns true. // Post: If the current item was already the last item in the // sequence, then there is no longer any current item. Otherwise, // the new current item is the item immediately after the original // current item. // // void insert(const value_type& entry) // Pre: none // Post: A new copy of entry has been inserted in the sequence // before the current item. If there was no current item, then // the new entry has been inserted at the front of the sequence. // In either case, the newly inserted item is now the current item // of the sequence. // // void attach(const value_type& entry) // Pre: none // Post: A new copy of entry has been inserted in the sequence after // the current item. If there was no current item, then the new // entry has been attached to the end of the sequence. In either // case, the newly inserted item is now the current item of the // sequence. // // void remove_current() // Pre: is_item returns true. // Post: The current item has been removed from the sequence, and // the item after this (if there is one) is now the new current // item. If the current item was already the last item in the // sequence, then there is no longer any current item. // // CONSTANT MEMBER FUNCTIONS for the sequence class: // size_type size() const // Pre: none // Post: The return value is the number of items in the sequence. // // bool is_item() const // Pre: none // Post: A true return value indicates that there is a valid // "current" item that may be retrieved by activating the current // member function (listed below). A false return value indicates // that there is no valid current item. // // value_type current() const // Pre: is_item() returns true. // Post: The item returned is the current item in the sequence. // // VALUE SEMANTICS for the sequence class: // Assignments and the copy constructor may be used with sequence // objects. #ifndef SEQUENCE_H #define SEQUENCE_H #include // provides size_t namespace CS3358_SP2023 { class sequence { public: // TYPEDEFS and MEMBER CONSTANTS typedef double value_type; typedef std::size_t size_type; static const size_type DEFAULT_CAPACITY = 30; // CONSTRUCTORS and DESTRUCTOR sequence(size_type initial_capacity = DEFAULT_CAPACITY); sequence(const sequence& source); ~sequence(); // MODIFICATION MEMBER FUNCTIONS void resize(size_type new_capacity); void start(); void advance(); void insert(const value_type& entry); void attach(const value_type& entry); void remove_current(); sequence& operator=(const sequence& source); // CONSTANT MEMBER FUNCTIONS size_type size() const; bool is_item() const; value_type current() const; private: value_type* data; size_type used; size_type current_index; size_type capacity; }; }
// TYPEDEFS and MEMBER CONSTANTS for the sequence class:
// typedef ____ value_type
// sequence::value_type is the data type of the items in the sequence.
// It may be any of the C++ built-in types (int, char, etc.), or a
// class with a default constructor, an assignment operator, and a
// copy constructor.
//
// typedef ____ size_type
// sequence::size_type is the data type of any variable that keeps
// track of how many items are in a sequence.
//
// static const size_type DEFAULT_CAPACITY = _____
// sequence::DEFAULT_CAPACITY is the default initial capacity of a
// sequence that is created by the default constructor.
//
// CONSTRUCTOR for the sequence class:
// sequence(size_type initial_capacity = DEFAULT_CAPACITY)
// Pre: initial_capacity > 0
// Post: The sequence has been initialized as an empty sequence.
// The insert/attach functions will work efficiently (without
// allocating new memory) until this capacity is reached.
// Note: If Pre is not met, initial_capacity will be adjusted to 1.
//
// MODIFICATION MEMBER FUNCTIONS for the sequence class:
// void resize(size_type new_capacity)
// Pre: new_capacity > 0
// Post: The sequence's current capacity is changed to new_capacity
// (but not less that the number of items already on the sequence).
// The insert/attach functions will work efficiently (without
// allocating new memory) until this new capacity is reached.
// Note: If new_capacity is less than used, it will be made equal to
// to used (in order to preserve existing data). Thereafter, if Pre
// is not met, new_capacity will be adjusted to 1.
//
// void start()
// Pre: none
// Post: The first item on the sequence becomes the current item
// (but if the sequence is empty, then there is no current item).
//
// void advance()
// Pre: is_item returns true.
// Post: If the current item was already the last item in the
// sequence, then there is no longer any current item. Otherwise,
// the new current item is the item immediately after the original
// current item.
//
// void insert(const value_type& entry)
// Pre: none
// Post: A new copy of entry has been inserted in the sequence
// before the current item. If there was no current item, then
// the new entry has been inserted at the front of the sequence.
// In either case, the newly inserted item is now the current item
// of the sequence.
//
// void attach(const value_type& entry)
// Pre: none
// Post: A new copy of entry has been inserted in the sequence after
// the current item. If there was no current item, then the new
// entry has been attached to the end of the sequence. In either
// case, the newly inserted item is now the current item of the
// sequence.
//
// void remove_current()
// Pre: is_item returns true.
// Post: The current item has been removed from the sequence, and
// the item after this (if there is one) is now the new current
// item. If the current item was already the last item in the
// sequence, then there is no longer any current item.
//
// CONSTANT MEMBER FUNCTIONS for the sequence class:
// size_type size() const
// Pre: none
// Post: The return value is the number of items in the sequence.
//
// bool is_item() const
// Pre: none
// Post: A true return value indicates that there is a valid
// "current" item that may be retrieved by activating the current
// member function (listed below). A false return value indicates
// that there is no valid current item.
//
// value_type current() const
// Pre: is_item() returns true.
// Post: The item returned is the current item in the sequence.
//
// VALUE SEMANTICS for the sequence class:
// Assignments and the copy constructor may be used with sequence
// objects.
#ifndef SEQUENCE_H
#define SEQUENCE_H
#include <cstdlib> // provides size_t
namespace CS3358_SP2023
{
class sequence
{
public:
// TYPEDEFS and MEMBER CONSTANTS
typedef double value_type;
typedef std::size_t size_type;
static const size_type DEFAULT_CAPACITY = 30;
// CONSTRUCTORS and DESTRUCTOR
sequence(size_type initial_capacity = DEFAULT_CAPACITY);
sequence(const sequence& source);
~sequence();
// MODIFICATION MEMBER FUNCTIONS
void resize(size_type new_capacity);
void start();
void advance();
void insert(const value_type& entry);
void attach(const value_type& entry);
void remove_current();
sequence& operator=(const sequence& source);
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is_item() const;
value_type current() const;
private:
value_type* data;
size_type used;
size_type current_index;
size_type capacity;
};
}
![139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
void sequence::remove_current ()
}
sequence& sequence::operator= (const sequence& source)
cout << "operator= (const sequence& source) not implemented yet" << endl;
return this;
{
if(is_item())
for (int i=current_index;i<(used-1);i++)
{
// CONSTANT MEMBER FUNCTIONS
sequence::size_type sequence::size() const
return used;
data[i] = data[i+1];
}
used--;
}
}
bool sequence::is_item() const
{
{
if (current_index < used)
{
return true;
}
else
return false;
sequence::value_type sequence::current() const
assert (is_item());
return data[current_index];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3dbb91f-777d-47b2-aa94-40b3e17142a5%2Fb9721450-3899-454f-8979-2b0b6e165a08%2Fsqdn3z_processed.png&w=3840&q=75)
![41 #include <cassert>
42 #include "Sequence.h"
43 #include <iostream>
using namespace std;
44
45
46 namespace CS3358_SP2023
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
93
94
95
{
96
97
// CONSTRUCTORS and DESTRUCTOR
sequence::sequence (size_type initial capacity) : capacity (initial_capacity), used(0), current_index(0)
if(capacity <1)
capacity = DEFAULT_CAPACITY;
data = new value_type [capacity] ;
sequence::sequence (const sequence& source): capacity (source.capacity), used (source.used), current_index(source.current_index)
data = new value_type [capacity] ;
copy (source.data, source.data + used, data);
}
sequence::~sequence()
{
}
// MODIFICATION MEMBER FUNCTIONS
void sequence: :resize(size_type new_capacity)
{
}
value_type* arr = new value_type [new_capacity]; // create a new arrays
for(int i = 0; i < new_capacity; i++) // copying the old array
{
arr[i] = data[i]; // copy each arry of elements
delete[] data;
}
void sequence::start()
delete[] data; // successfully delete old remove
data = arr;
87
88 // sequence, then there is no longer any current item. Otherwise, the new
89 // current item is the item immediately after the original current item.
90
void sequence:: advance()
91
92
}
current_index = 0;
{
// ensure that current_index is valid
if(is_item())
current_index++;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3dbb91f-777d-47b2-aa94-40b3e17142a5%2Fb9721450-3899-454f-8979-2b0b6e165a08%2Fmsinxnj_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

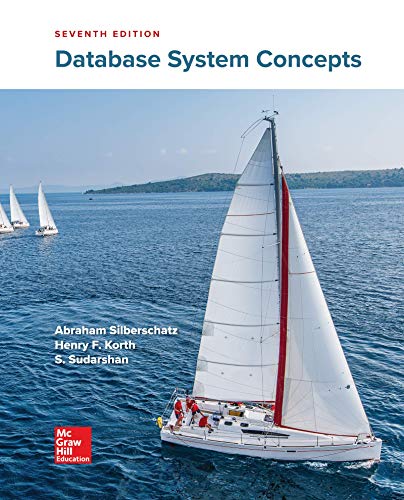
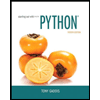
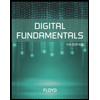
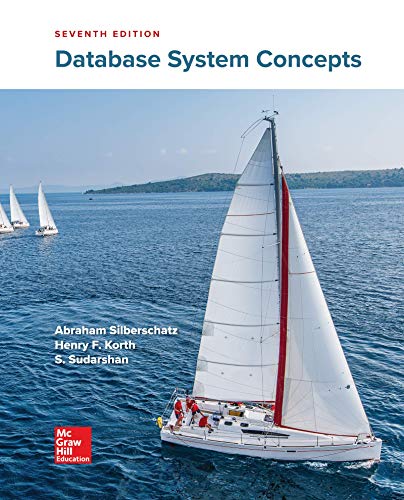
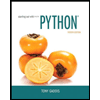
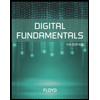
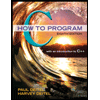
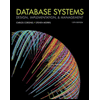
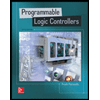