C++ Using the code provided below Do the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SC
In C++
Using the code provided below
Do the Following:
- Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools
- Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array)
USE THE FOLLOWING CODE and MODIFY IT:
#define _SECURE_SCL_DEPRECATE 0
#include <iostream>
#include <string>
#include <cstdlib>
using namespace std;
class GChar
{
public:
static const int DEFAULT_CAPACITY = 5;
//constructor
GChar(string name = "john", int capacity = DEFAULT_CAPACITY);
//copy constructor
GChar(const GChar& source);
//Overload Assignment
GChar& operator=(const GChar& source);
//Destructor
~GChar();
//Insert a New Tool
void insert(const std::string& toolName);
private:
//data members
string name;
int capacity;
int used;
string* toolHolder;
};
//constructor
GChar::GChar(string n, int cap)
{
name = n;
capacity = cap;
used = 0;
toolHolder = new string[cap];
}
//copy constructor
GChar::GChar(const GChar& source)
{
cout << "Copy Constructor Called." << endl;
name = source.name;
capacity = source.capacity;
used = source.used;
toolHolder = new string[source.capacity];
copy(source.toolHolder, source.toolHolder + used, toolHolder);
}
//Overload Assignment
GChar& GChar::operator=(const GChar& source)
{
cout << "Overload Assignment Called." << endl;
//Check for self assignment
//c1=c1
if (this == &source)
{
return *this;
}
this->name = source.name;
this->capacity = source.capacity;
used = source.used;
copy(source.toolHolder, source.toolHolder + used, this->toolHolder);
}
//Destructor
GChar::~GChar()
{
cout << "Destructor called for " << this->name << " @ this memory location " << this << endl;
delete[] toolHolder;
}
void GChar::insert(const string& toolName)
{
if (used == capacity)
{
cout << " The tool holder is full, Cannot add any additional tools" << endl;
}
else
{
toolHolder[used] = toolName;
used++;
}
}
int main()
{
GChar gc1;
gc1.insert("potion");
gc1.insert("crossbow");
gc1.insert("candle");
gc1.insert("cloak");
gc1.insert("sword");
gc1.insert("book of spells");
GChar gc2("Bob", 5);
GChar gc3 = gc2;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

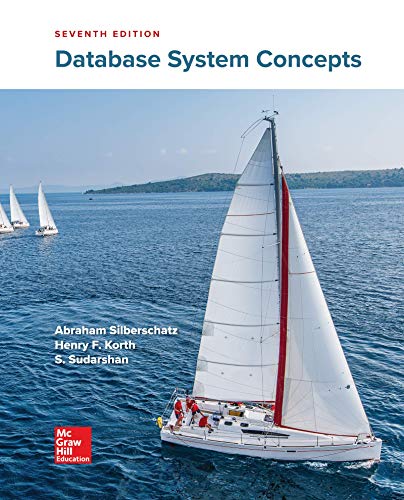
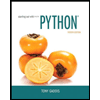
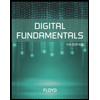
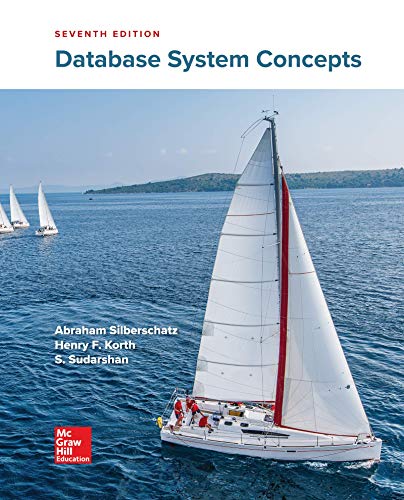
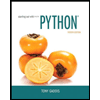
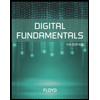
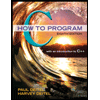
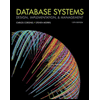
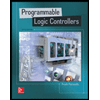