C++ programming task. main.cc file: #include #include #include #include "plane.h" int main() { std::vector weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3}; std::cout << "Printing out all the weights: " << std::endl; // ======= YOUR CODE HERE ======== // 1. Using an iterator, print out all the elements in // the weights vector on one line, separated by spaces. // Hint: see the README for the for loop syntax using // an iterator, .begin(), and .end() // ========================== std::cout << std::endl; std::map abbrevs{{"AL", "Alabama"}, {"CA", "California"}, {"GA", "Georgia"}, {"TX", "Texas"}}; std::map populations{ {"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}}; std::cout << "\nPrinting out the state populations: " << std::endl; // ========= YOUR CODE HERE ========= // 2. Using an iterator, print out each state's population // on a new line, in the format: // "Population of Alabama: 5.1 million" // "Population of California: 39.2 million" // ... and so on // // * abbrevs maps from the state abbreviation to the // full state name. // * populations maps from the state abbreviation to // to the population (in millions) // Use the abbrevs map to retrieve the full state name // to print out, while iterating over the populations // map. // ======================= std::cout << std::endl; // 3. Implement the constructors of the Plane class, in // "plane.h". Refer to the README or the comments in // "plane.h" for instructions. // ======= YOUR CODE HERE ======== // 4. Create an empty vector of Plane objects called `fleet`. // ========================== // =========== YOUR CODE HERE ======= // 5. Create a Plane `p1` instantiated with the default // constructor. Add `p1` to the `fleet` vector. // ========================= // ======= YOUR CODE HERE ==== // 6. Use the constructor overload to create a Plane `p2` // with 150 seats, 75 passengers, and destination // "New York City". Add `p2` to the `fleet` vector. // ================== // Uncomment these lines of code after completing #3-6. // Plane p3(220, 220, "Atlanta"); // Plane p4(75, 75, "Guatemala City"); // Plane p5(125, 94, "Medellin"); // ====== YOUR CODE HERE ==== // 7. Add `p3`, `p4`, and `p5` to the `fleet` vector. // ========================== // ======= YOUR CODE HERE ======= // 8. Using an iterator, print out all the flights in // the `fleet` vector, only if the flights are full. // Hint: see the README for the expected format. // ========================= plane.cc file #include "plane.h" // ============ YOUR CODE HERE ========== // This implementation file (plane.cc) is where you should implement // the member functions declared in the header (plane.h), only // if you didn't implement them inline within plane.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Plane class. // ============================================= plane.h file #include class Plane { public: // ========== YOUR CODE HERE ========== // Define the following constructors using member initializer // list syntax: // // 1. A default constructor, which initializes seat count // to 121, passenger count to 121, and the flight // destination to "Fullerton". // 2. A constructor overload which accepts the seat count, // passenger count, and flight destination and initializes // each corresponding member variable appropriately. // =========================== int GetPassengerCount() const { return passenger_count_; } void SetPassengerCount(int passengers) { passenger_count_ = passengers; } int GetSeatCount() const { return seat_count_; } void SetSeatCount(int seats) { seat_count_ = seats; } const std::string &GetDestination() const { return destination_; } void SetDestination(const std::string &dest) { destination_ = dest; } private: int seat_count_; int passenger_count_; std::string destination_; }; Expected Output: 121 passengers flying to Fullerton 220 passengers flying to Atlanta 75 passengers flying to Guatemala City
C++ programming task.
main.cc file:
#include <iostream>
#include <map>
#include <
#include "plane.h"
int main() {
std::vector<double> weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3};
std::cout << "Printing out all the weights: " << std::endl;
// ======= YOUR CODE HERE ========
// 1. Using an iterator, print out all the elements in
// the weights vector on one line, separated by spaces.
// Hint: see the README for the for loop syntax using
// an iterator, .begin(), and .end()
// ==========================
std::cout << std::endl;
std::map<std::string, std::string> abbrevs{{"AL", "Alabama"},
{"CA", "California"},
{"GA", "Georgia"},
{"TX", "Texas"}};
std::map<std::string, double> populations{
{"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}};
std::cout << "\nPrinting out the state populations: " << std::endl;
// ========= YOUR CODE HERE =========
// 2. Using an iterator, print out each state's population
// on a new line, in the format:
// "Population of Alabama: 5.1 million"
// "Population of California: 39.2 million"
// ... and so on
//
// * abbrevs maps from the state abbreviation to the
// full state name.
// * populations maps from the state abbreviation to
// to the population (in millions)
// Use the abbrevs map to retrieve the full state name
// to print out, while iterating over the populations
// map.
// =======================
std::cout << std::endl;
// 3. Implement the constructors of the Plane class, in
// "plane.h". Refer to the README or the comments in
// "plane.h" for instructions.
// ======= YOUR CODE HERE ========
// 4. Create an empty vector of Plane objects called `fleet`.
// ==========================
// =========== YOUR CODE HERE =======
// 5. Create a Plane `p1` instantiated with the default
// constructor. Add `p1` to the `fleet` vector.
// =========================
// ======= YOUR CODE HERE ====
// 6. Use the constructor overload to create a Plane `p2`
// with 150 seats, 75 passengers, and destination
// "New York City". Add `p2` to the `fleet` vector.
// ==================
// Uncomment these lines of code after completing #3-6.
// Plane p3(220, 220, "Atlanta");
// Plane p4(75, 75, "Guatemala City");
// Plane p5(125, 94, "Medellin");
// ====== YOUR CODE HERE ====
// 7. Add `p3`, `p4`, and `p5` to the `fleet` vector.
// ==========================
// ======= YOUR CODE HERE =======
// 8. Using an iterator, print out all the flights in
// the `fleet` vector, only if the flights are full.
// Hint: see the README for the expected format.
// =========================
plane.cc file
#include "plane.h"
// ============ YOUR CODE HERE ==========
// This implementation file (plane.cc) is where you should implement
// the member functions declared in the header (plane.h), only
// if you didn't implement them inline within plane.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Plane class.
// =============================================
plane.h file
#include <string>
class Plane {
public:
// ========== YOUR CODE HERE ==========
// Define the following constructors using member initializer
// list syntax:
//
// 1. A default constructor, which initializes seat count
// to 121, passenger count to 121, and the flight
// destination to "Fullerton".
// 2. A constructor overload which accepts the seat count,
// passenger count, and flight destination and initializes
// each corresponding member variable appropriately.
// ===========================
int GetPassengerCount() const {
return passenger_count_;
}
void SetPassengerCount(int passengers) {
passenger_count_ = passengers;
}
int GetSeatCount() const {
return seat_count_;
}
void SetSeatCount(int seats) {
seat_count_ = seats;
}
const std::string &GetDestination() const {
return destination_;
}
void SetDestination(const std::string &dest) {
destination_ = dest;
}
private:
int seat_count_;
int passenger_count_;
std::string destination_;
};
Expected Output:
121 passengers flying to Fullerton
220 passengers flying to Atlanta
75 passengers flying to Guatemala City



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

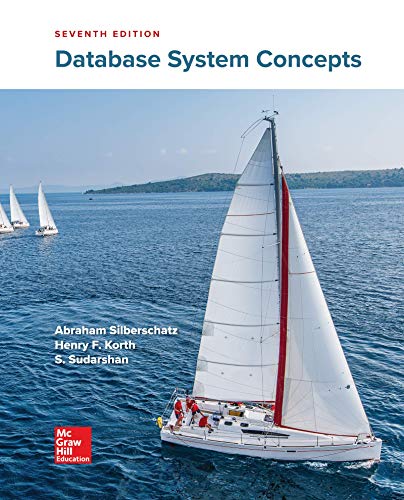
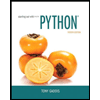
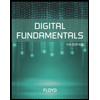
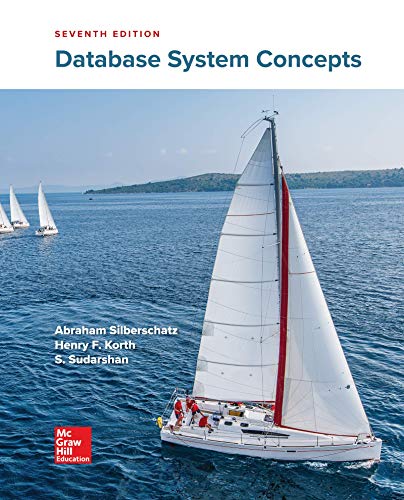
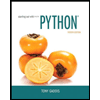
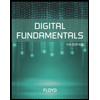
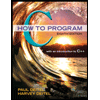
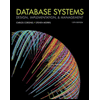
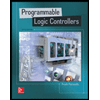