C++ Program: #include #include using namespace std; const int AIRPORT_COUNT = 12; string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main() { // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl; // Retrieve data from the STACK/QUEUE here }
C++ Program:
#include <iostream>
#include <string>
using namespace std;
const int AIRPORT_COUNT = 12;
string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"};
int main()
{
// define stack (or queue ) here
string origin;
string dest;
string citypair;
cout << "Loading the CONTAINER ..." << endl;
// LOAD THE STACK ( or queue) HERE
// Create all the possible Airport combinations that could exist from the list provided.
// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...
// DO NOT Load SameSame - DALDAL, ABQABQ, etc ..
cout << "Getting data from the CONTAINER ..." << endl;
// Retrieve data from the STACK/QUEUE here
}
Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic.
Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible origin/destinations possible combinations from the airport codes provided. Load the origin/destination string onto a stack for processing. Do not load same/same values, such as DALDAL, or LAXLAX. See comments in the program from more details.
After loading the values, Create a loop to display all the values in the container. This loop should inherently remove items as they are displayed.
Deliverable is a working CPP program.

Step by step
Solved in 2 steps

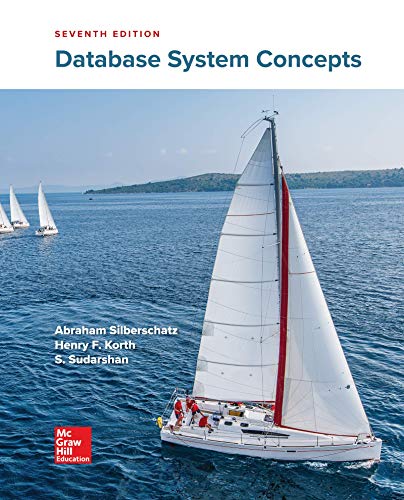
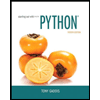
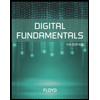
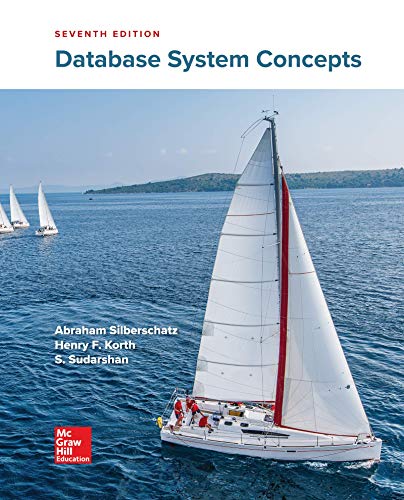
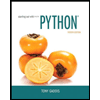
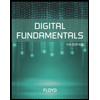
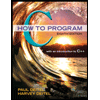
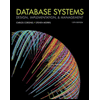
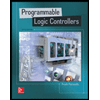