C++ help with this fixing code .cpp file #include #include #include #include"Food.h" using namespace std; class Nutrition{ private: string name; double Calories double Fat double Sugars double Protein double Sodium Nutrition(string n, double c, double f, double s, double p, double S): name(n), Calories(c), Fat(f),Sugars(s), Protein(p),Sodium(S){} food[Calories]= food[Fat]=food[Sugars]=food[Protein]=food[Sodium]=0; name = ""; } Nutrition(string type, double calories, double fat, double sugar,double protein, double sodium){ food[Calories]= calories; food[Fat]=fat; food[Sugars]= sugar; food[Protein]=protein; food[Sodium]=sodium; name= type; } void setName(string type){ name = type; } void setCalories(double calories){ food [Calories]= calories; } void setFat(double fat){ food [Fat]= fat; } void setSugars(double sugar){ food [Sugars]= sugar; } void setProtein(double protein){ food [Protein]= protein; } void setSodium(double sodium){ food [Sodium]= sodium; } string getName(){ return name; } double setCalories(){ return food[Calories]; } double getFat(){ return food[Fat]; } double getSugars(){ return food[Sugars]; } double getProtein(){ return food [Protein]; } double getSodium(){ return food[Sodium]; } .h file #ifndef FOOD_H #define FOOD_H Nutrition operator + (Nutrition & F){ Nutrition temp; temp.food[Calories]= food[Calories + F.getCalories()]; temp.food[Fat] = food[Fat] + f.getFat(); temp.food[Sugars]= food[Sugars] + F.getSugars(); temp.food[Protein]= food[Protein]+ F.getProtein(); temp.food[Sodium] = food[Sodium] + F.getSodium(); return temp; } } void display (Food text){ cout<< "Your total intake is"<Nutrition Items; Nutrition I1("Apple",1 4,5,7); Nutrition I2(" French fries", 2, 5 ,6); Nutrition I3("Burger",3, 50,60, 4,5); Nutrition I4( "Pizza",4,36,43,4,4); Nutrition I5(" Ramen", 5,22,34,5,5); Nutrition I6("Steak", 6,23,27,10,10); Nutrition I7(" Chicken", 7,19,17,7,7); Nutrition I8(" Rice", 8, 16,3,2,2); Nutrition I9(" Pork", 9,17,17,8,8); Nutrition I10(" Carrots",10,17,1,1); Nutrition I11("FInished"); Items.push_back(I1); Items.push_back(I2); Items.push_back(I3); Items.push_back(I4); Items.push_back(I5); Items.push_back(I6); Items.push_back(I7); Items.push_back(I8); Items.push_back(I9); Items.push_back(I10); Items.push_back(I11); void excess(Nutrition text){ if (text.getCalories() > averageFood[Calories]){ cout<<" You exceeded the suggested amount"<< endl; } if (text.getFat() > averageFood[Fat]){ cout<<" You exceeded the suggested amount"<< endl; } if (text.getSugars() > averageFood[Sugars]){ cout<<" You exceeded the suggested amount"<< endl; } if (text.getProtein() > averageFood[Protein]){ cout<<" You exceeded the suggested amount"<< endl; } if (text.getSodium() > averageFood[Sodium]){ cout<<" You exceeded the suggested amount"<< endl; } } int main(){ Nutrition Items[MAX]; int size; int choice =-1; Nutrition selected; int selected Item = 0; while ( choice != size +2){ for (int i = 0; i < size; i++){ cout << i + 1 << ":"<< list[i].get Nmaw()<> choice; if (choice == size +1){ size= addItem(list,size); } else if )choice < size && choice>0){ selected= selected +Items[choice - 1]; selectedItemCount++; } else if (choice<1 || choice>size +2){ cout << "invalid"< 0){ print (selected); cout << endl; checkexcess(selected); } } return 0; } create a class to contain a "Food Item". This food item class should track the name of the food, calories, total fat, total sugars, protein, and sodium. The class's member variables should only be accessible via public accessors or mutators, if necessary. It should contain no public member variables. Override the addition operator to allow food items to be added together. The result of the addition should populate a class containing the combined nutritional value of the two items. Use this operator to sum up the nutritional value of all the food items selected by the user. The program should display at least 10 pre-populated items for the user to choose from. After all food entries have been entered into the console and the total nutritional value has been computed, display to the user if they have exceeded any of the recommended intakes for an average adult: The addition operation is properly overloaded. The resulting class will contain the combined values of each nutrient member variable. Class files are stored in separate files The class is separated into .cpp and .h files properly.
C++ help with this fixing code
.cpp file
#include<iostream>
#include<string>
#include<
#include"Food.h"
using namespace std;
class Nutrition{
private:
string name;
double Calories
double Fat
double Sugars
double Protein
double Sodium
Nutrition(string n, double c, double f, double s, double p, double S): name(n), Calories(c), Fat(f),Sugars(s), Protein(p),Sodium(S){}
food[Calories]= food[Fat]=food[Sugars]=food[Protein]=food[Sodium]=0;
name = "";
}
Nutrition(string type, double calories, double fat, double sugar,double protein, double sodium){
food[Calories]= calories; food[Fat]=fat; food[Sugars]= sugar; food[Protein]=protein; food[Sodium]=sodium; name= type;
}
void setName(string type){
name = type;
}
void setCalories(double calories){
food [Calories]= calories;
}
void setFat(double fat){
food [Fat]= fat;
}
void setSugars(double sugar){
food [Sugars]= sugar;
}
void setProtein(double protein){
food [Protein]= protein;
}
void setSodium(double sodium){
food [Sodium]= sodium;
}
string getName(){
return name;
}
double setCalories(){
return food[Calories];
}
double getFat(){
return food[Fat];
}
double getSugars(){
return food[Sugars];
}
double getProtein(){
return food [Protein];
}
double getSodium(){
return food[Sodium];
}
.h file
#ifndef FOOD_H
#define FOOD_H
Nutrition operator + (Nutrition & F){
Nutrition temp;
temp.food[Calories]= food[Calories + F.getCalories()];
temp.food[Fat] = food[Fat] + f.getFat();
temp.food[Sugars]= food[Sugars] + F.getSugars();
temp.food[Protein]= food[Protein]+ F.getProtein();
temp.food[Sodium] = food[Sodium] + F.getSodium();
return temp;
}
}
void display (Food text){
cout<< "Your total intake is"<<endl;
cout<< " Calories consumed:"<< text.getCalories()<<endl;
cout<< " Total Sugar consumed:"<< text.getSugars()<<endl;
cout<< " Protein consumed:"<< text.getProtein()<<endl;
cout<< " Sodium consumed" << text.getSodium<<endl;
cout<< " Fat consumed:" << text.getFat()<<endl;
}
vector<Nutrirtion>Nutrition Items;
Nutrition I1("Apple",1 4,5,7);
Nutrition I2(" French fries", 2, 5 ,6);
Nutrition I3("Burger",3, 50,60, 4,5);
Nutrition I4( "Pizza",4,36,43,4,4);
Nutrition I5(" Ramen", 5,22,34,5,5);
Nutrition I6("Steak", 6,23,27,10,10);
Nutrition I7(" Chicken", 7,19,17,7,7);
Nutrition I8(" Rice", 8, 16,3,2,2);
Nutrition I9(" Pork", 9,17,17,8,8);
Nutrition I10(" Carrots",10,17,1,1);
Nutrition I11("FInished");
Items.push_back(I1);
Items.push_back(I2);
Items.push_back(I3);
Items.push_back(I4);
Items.push_back(I5);
Items.push_back(I6);
Items.push_back(I7);
Items.push_back(I8);
Items.push_back(I9);
Items.push_back(I10);
Items.push_back(I11);
void excess(Nutrition text){
if (text.getCalories() > averageFood[Calories]){
cout<<" You exceeded the suggested amount"<< endl;
}
if (text.getFat() > averageFood[Fat]){
cout<<" You exceeded the suggested amount"<< endl;
}
if (text.getSugars() > averageFood[Sugars]){
cout<<" You exceeded the suggested amount"<< endl;
}
if (text.getProtein() > averageFood[Protein]){
cout<<" You exceeded the suggested amount"<< endl;
}
if (text.getSodium() > averageFood[Sodium]){
cout<<" You exceeded the suggested amount"<< endl;
}
}
int main(){
Nutrition Items[MAX];
int size;
int choice =-1;
Nutrition selected;
int selected Item = 0;
while ( choice != size +2){
for (int i = 0; i < size; i++){
cout << i + 1 << ":"<< list[i].get Nmaw()<<endl;
}
cout << size +2 << ": Finished"<< endl;
cin>> choice;
if (choice == size +1){
size= addItem(list,size);
}
else if )choice < size && choice>0){
selected= selected +Items[choice - 1];
selectedItemCount++;
}
else if (choice<1 || choice>size +2){
cout << "invalid"<<endl;
}
if ( selectedItemCount > 0){
print (selected);
cout << endl;
checkexcess(selected);
}
}
return 0;
}
create a class to contain a "Food Item". This food item class should track the name of the food, calories, total fat, total sugars, protein, and sodium. The class's member variables should only be accessible via public accessors or mutators, if necessary. It should contain no public member variables.
Override the addition operator to allow food items to be added together. The result of the addition should populate a class containing the combined nutritional value of the two items. Use this operator to sum up the nutritional value of all the food items selected by the user.
The program should display at least 10 pre-populated items for the user to choose from.
After all food entries have been entered into the console and the total nutritional value has been computed, display to the user if they have exceeded any of the recommended intakes for an average adult:
Class files are stored in separate files

Step by step
Solved in 2 steps

Where are the list of food items that were made using the
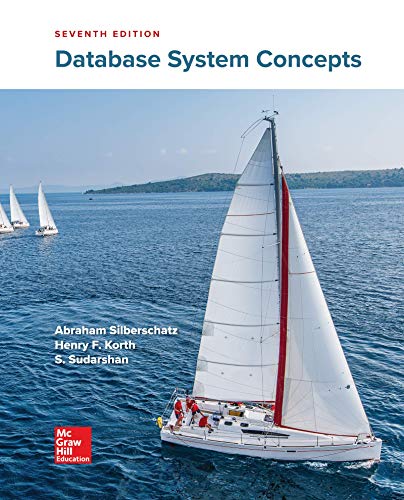
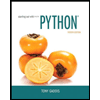
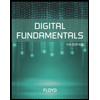
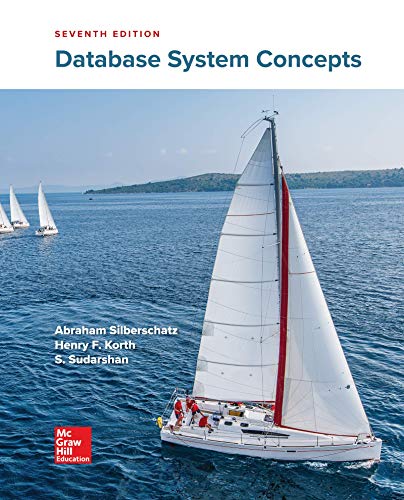
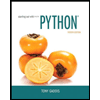
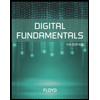
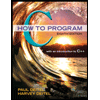
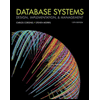
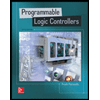