main.cc file #include #include #include #include "cup.h" int main() { std::string drink_name; double amount = 0.0; std::cout << "What kind of drink can I get you?: "; std::getline(std::cin, drink_name); while (std::cout << "How much do you want to fill?: " && !(std::cin >> amount)) { // If the input is invalid, clear it, and ask again std::cin.clear(); std::cin.ignore(); std::cout << "Invalid input; please re-enter.\n"; } //================== YOUR CODE HERE ================== // Instantiate a `Cup` object named `mug`, with // the drink_name and amount given by the user above. //==================================================== while (true) { char menu_input = 'X'; std::cout << "\n=== Your cup currently has " << mug.GetFluidOz() << " oz. of " << mug.GetDrinkType() << " === \n\n"; std::cout << "Please select what you want to do with your drink/cup?:\n"; std::cout << "D: Drink\n"; std::cout << "R: Refill\n"; std::cout << "N: Get a brand new drink\n"; std::cout << "E: Empty\n"; std::cout << "X: Exit\n"; std::cout << "Selection: "; std::cin >> menu_input; std::cin.ignore(); if (menu_input == 'X' || menu_input == 'x') { std::cout << "Thank you for using our cup!\n"; break; } if (menu_input == 'D' || menu_input == 'd') { std::cout << "How much do you want to drink from the cup?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `Drink` function here. //==================================================== } else if (menu_input == 'R' || menu_input == 'r') { std::cout << "How much do you want to refill your cup?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `Refill` function here. //==================================================== } else if (menu_input == 'N' || menu_input == 'n') { std::cout << "What is the new drink you want?: "; std::getline(std::cin, drink_name); std::cout << "What is the amount you want?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `NewDrink` function here. //==================================================== } else if (menu_input == 'E' || menu_input == 'e') { std::cout << "Emptying your cup\n"; //================== YOUR CODE HERE ================== // Call the `Empty` function here. //==================================================== } else { std::cout << "Invalid use of a cup!\n"; } } return0; } cup.cc file #include "cup.h" #include //========================== YOUR CODE HERE ========================== // Implement the member functions for the Cup class in this // file. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Cup class. //==================================================================== cup.h file #include //====================== YOUR CODE HERE ====================== // Declare the Cup class in this file, with the member // variables and member functions as described in the README. //============================================================ class Cup { public: //================== YOUR CODE HERE ================== // Add the member function declarations here. //==================================================== private: //================== YOUR CODE HERE ================== // Add the member variable declarations here. //==================================================== }; Sample output picture link https://imgur.com/a/DtAQurD
main.cc file #include #include #include #include "cup.h" int main() { std::string drink_name; double amount = 0.0; std::cout << "What kind of drink can I get you?: "; std::getline(std::cin, drink_name); while (std::cout << "How much do you want to fill?: " && !(std::cin >> amount)) { // If the input is invalid, clear it, and ask again std::cin.clear(); std::cin.ignore(); std::cout << "Invalid input; please re-enter.\n"; } //================== YOUR CODE HERE ================== // Instantiate a `Cup` object named `mug`, with // the drink_name and amount given by the user above. //==================================================== while (true) { char menu_input = 'X'; std::cout << "\n=== Your cup currently has " << mug.GetFluidOz() << " oz. of " << mug.GetDrinkType() << " === \n\n"; std::cout << "Please select what you want to do with your drink/cup?:\n"; std::cout << "D: Drink\n"; std::cout << "R: Refill\n"; std::cout << "N: Get a brand new drink\n"; std::cout << "E: Empty\n"; std::cout << "X: Exit\n"; std::cout << "Selection: "; std::cin >> menu_input; std::cin.ignore(); if (menu_input == 'X' || menu_input == 'x') { std::cout << "Thank you for using our cup!\n"; break; } if (menu_input == 'D' || menu_input == 'd') { std::cout << "How much do you want to drink from the cup?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `Drink` function here. //==================================================== } else if (menu_input == 'R' || menu_input == 'r') { std::cout << "How much do you want to refill your cup?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `Refill` function here. //==================================================== } else if (menu_input == 'N' || menu_input == 'n') { std::cout << "What is the new drink you want?: "; std::getline(std::cin, drink_name); std::cout << "What is the amount you want?: "; std::cin >> amount; std::cin.ignore(); //================== YOUR CODE HERE ================== // Call the `NewDrink` function here. //==================================================== } else if (menu_input == 'E' || menu_input == 'e') { std::cout << "Emptying your cup\n"; //================== YOUR CODE HERE ================== // Call the `Empty` function here. //==================================================== } else { std::cout << "Invalid use of a cup!\n"; } } return0; } cup.cc file #include "cup.h" #include //========================== YOUR CODE HERE ========================== // Implement the member functions for the Cup class in this // file. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Cup class. //==================================================================== cup.h file #include //====================== YOUR CODE HERE ====================== // Declare the Cup class in this file, with the member // variables and member functions as described in the README. //============================================================ class Cup { public: //================== YOUR CODE HERE ================== // Add the member function declarations here. //==================================================== private: //================== YOUR CODE HERE ================== // Add the member variable declarations here. //==================================================== }; Sample output picture link https://imgur.com/a/DtAQurD
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
main.cc file
#include <iomanip> | |
#include <iostream> | |
#include <string> | |
#include "cup.h" | |
int main() { | |
std::string drink_name; | |
double amount = 0.0; | |
std::cout << "What kind of drink can I get you?: "; | |
std::getline(std::cin, drink_name); | |
while (std::cout << "How much do you want to fill?: " && | |
!(std::cin >> amount)) { | |
// If the input is invalid, clear it, and ask again | |
std::cin.clear(); | |
std::cin.ignore(); | |
std::cout << "Invalid input; please re-enter.\n"; | |
} | |
//================== YOUR CODE HERE ================== | |
// Instantiate a `Cup` object named `mug`, with | |
// the drink_name and amount given by the user above. | |
//==================================================== | |
while (true) { | |
char menu_input = 'X'; | |
std::cout << "\n=== Your cup currently has " << mug.GetFluidOz() | |
<< " oz. of " << mug.GetDrinkType() << " === \n\n"; | |
std::cout << "Please select what you want to do with your drink/cup?:\n"; | |
std::cout << "D: Drink\n"; | |
std::cout << "R: Refill\n"; | |
std::cout << "N: Get a brand new drink\n"; | |
std::cout << "E: Empty\n"; | |
std::cout << "X: Exit\n"; | |
std::cout << "Selection: "; | |
std::cin >> menu_input; | |
std::cin.ignore(); | |
if (menu_input == 'X' || menu_input == 'x') { | |
std::cout << "Thank you for using our cup!\n"; | |
break; | |
} | |
if (menu_input == 'D' || menu_input == 'd') { | |
std::cout << "How much do you want to drink from the cup?: "; | |
std::cin >> amount; | |
std::cin.ignore(); | |
//================== YOUR CODE HERE ================== | |
// Call the `Drink` function here. | |
//==================================================== | |
} else if (menu_input == 'R' || menu_input == 'r') { | |
std::cout << "How much do you want to refill your cup?: "; | |
std::cin >> amount; | |
std::cin.ignore(); | |
//================== YOUR CODE HERE ================== | |
// Call the `Refill` function here. | |
//==================================================== | |
} else if (menu_input == 'N' || menu_input == 'n') { | |
std::cout << "What is the new drink you want?: "; | |
std::getline(std::cin, drink_name); | |
std::cout << "What is the amount you want?: "; | |
std::cin >> amount; | |
std::cin.ignore(); | |
//================== YOUR CODE HERE ================== | |
// Call the `NewDrink` function here. | |
//==================================================== | |
} else if (menu_input == 'E' || menu_input == 'e') { | |
std::cout << "Emptying your cup\n"; | |
//================== YOUR CODE HERE ================== | |
// Call the `Empty` function here. | |
//==================================================== | |
} else { | |
std::cout << "Invalid use of a cup!\n"; | |
} | |
} | |
return0; | |
} |
cup.cc file
#include "cup.h" | |
#include <iostream> | |
//========================== YOUR CODE HERE ========================== | |
// Implement the member functions for the Cup class in this | |
// file. | |
// | |
// Remember to specify the name of the class with :: in this format: | |
// <return type> MyClassName::MyFunction() { | |
// ... | |
// } | |
// to tell the compiler that each function belongs to the Cup class. | |
//==================================================================== |
cup.h file
#include <iostream> | |
//====================== YOUR CODE HERE ====================== | |
// Declare the Cup class in this file, with the member | |
// variables and member functions as described in the README. | |
//============================================================ | |
class Cup { | |
public: | |
//================== YOUR CODE HERE ================== | |
// Add the member function declarations here. | |
//==================================================== | |
private: | |
//================== YOUR CODE HERE ================== | |
// Add the member variable declarations here. | |
//==================================================== | |
}; |
Sample output picture link
https://imgur.com/a/DtAQurD

Transcribed Image Text:Digicup
This program simulates interactions with a Cup object for getting a drink, refilling a drink, emptying a drink, and drinking from it.
Output
All of the output statements (std::cout) should be in main and are mostly provided for you. You will only need to complete missing logic
to construct a Cup object and call the appropriate member functions in the Cup class.
The Cup object will be used to ask the user for the type of drink they prefer and the amount they want to drink.
The menu options are shown below for your reference:
D: Drink
R: Refill
N: Get a brand new drink
E: Empty
X: Exit
The Cup class
State (member variables)
Create a class called Cup with the following member variables:
1. drink_type_ which is a std::string that will be the name of the drink.
2. fluid_oz_ which is a double that will be the amount of fluid in the cup.
Constructors
Default constructor
The default constructor should initialize drink_type_ to "Water" and initialize fluid_oz_ to 16.0
Non-default constructor

Transcribed Image Text:Non-default constructor
The non-default constructor should take in a std::string parameter that will be the name of the drink type and a double parameter that
will be the amount of drink in the cup in fluid ounces. It should set the passed parameter values to the corresponding data members.
Member functions
Drink
Create a function called Drink which accepts one double as a parameter. Drinking reduces the amount of liquid in the Cup based on a
given double that is passed as input. Take note that fluid_oz_ should never be negative, so if you drink an amount that is greater than
fluid_oz_, then fluid_oz_ should be set to 0.
Refill
Create a function called Refill which accepts one double as a parameter. Refilling the cup increases the amount of liquid in the Cup
based on the given input. Assume the cup is bottomless.
NewDrink
Create a function called NewDrink which accepts two parameters. The first is the name of the new drink type and the second is the
amount of the new drink type. NewDrink should throw out your current drink and replace it with a new drink. Make sure to replace the
value of the member variables in this function.
Empty
Empties out the contents of the cup in its entirety. fluid_oz_ should be set to 0, and _drink_type should be set to "nothing"
Accessors
Create the accessor for fluid_oz_, and name it GetFluidoz. Create the accessor for drink_type_, and name it GetDrinkType. Don't
forget, accessors/getters should be marked const since they don't make modifications to the member variables.
Other information
Declare the Cup class in cup.h, implement the member functions in cup.cc, and complete the code in main.cc.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
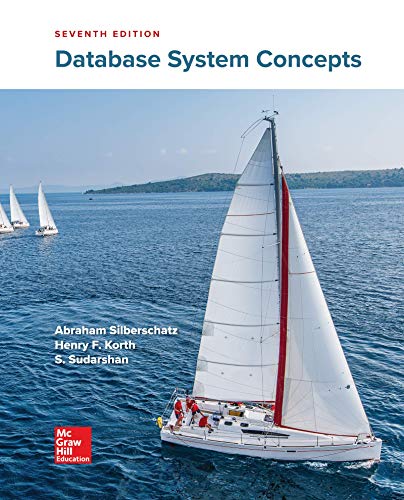
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
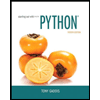
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
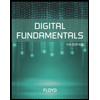
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
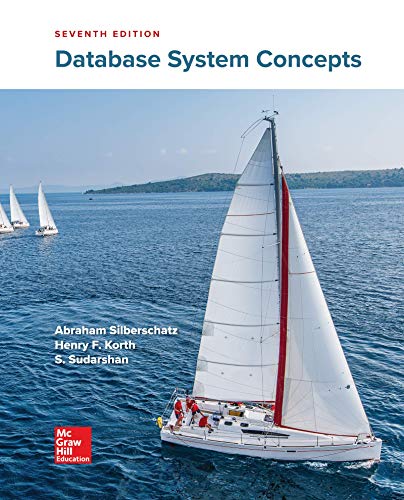
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
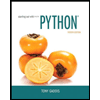
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
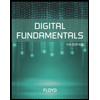
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
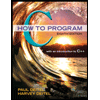
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
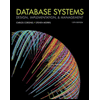
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
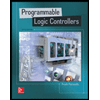
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education