C++ Programming main.cc file #include #include #include #include "bank.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Bank object, name it anything you'd like :) // ======================================================= // =================== YOUR CODE HERE =================== // 2. Create 3 new accounts in your bank. // * The 1st account should belong to "Tuffy", with // a balance of $121.00 // * The 2nd account should belong to "Frank", with // a balance of $1234.56 // * The 3nd account should belong to "Oreo", with // a balance of $140.12 // ======================================================= // =================== YOUR CODE HERE =================== // 3. Deactivate Tuffy's account. // ======================================================= // =================== YOUR CODE HERE =================== // 4. Call DisplayBalances to print out all *active* // account balances. // ======================================================= } bank.h file #include #include #include #include #include #include #include "account.h" class Bank { public: // ======================= YOUR CODE HERE ======================= // Write the Bank class here. Refer to the README for the member // variables, constructors, and member functions needed. // // Note: mark functions that do not modify the member variables // as const, by writing `const` after the parameter list. // Pass objects by const reference when appropriate. // Remember that std::string is an object! // =============================================================== private: // We provided this helper function to you to randomly generate // a new Bank Account ID to be used in CreateAccount. intGenerateAccountId() const { returnstd::rand() % 9000 + 1000; // [1000, 9999] } }; bank.cc file #include "bank.h" // ========================= YOUR CODE HERE ========================= // This implementation file (bank.cc) is where you should implement // the member functions declared in the header (bank.h), only // if you didn't implement them inline within bank.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Bank class. // =================================================================== account.h file #include class Account { public: Account(const std::string& name, double balance) : account_holder_(name), balance_(balance) {} const std::string& GetAccountHolder() const { return account_holder_; } double GetBalance() const { return balance_; } private:
C++ Programming main.cc file #include #include #include #include "bank.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Bank object, name it anything you'd like :) // ======================================================= // =================== YOUR CODE HERE =================== // 2. Create 3 new accounts in your bank. // * The 1st account should belong to "Tuffy", with // a balance of $121.00 // * The 2nd account should belong to "Frank", with // a balance of $1234.56 // * The 3nd account should belong to "Oreo", with // a balance of $140.12 // ======================================================= // =================== YOUR CODE HERE =================== // 3. Deactivate Tuffy's account. // ======================================================= // =================== YOUR CODE HERE =================== // 4. Call DisplayBalances to print out all *active* // account balances. // ======================================================= } bank.h file #include #include #include #include #include #include #include "account.h" class Bank { public: // ======================= YOUR CODE HERE ======================= // Write the Bank class here. Refer to the README for the member // variables, constructors, and member functions needed. // // Note: mark functions that do not modify the member variables // as const, by writing `const` after the parameter list. // Pass objects by const reference when appropriate. // Remember that std::string is an object! // =============================================================== private: // We provided this helper function to you to randomly generate // a new Bank Account ID to be used in CreateAccount. intGenerateAccountId() const { returnstd::rand() % 9000 + 1000; // [1000, 9999] } }; bank.cc file #include "bank.h" // ========================= YOUR CODE HERE ========================= // This implementation file (bank.cc) is where you should implement // the member functions declared in the header (bank.h), only // if you didn't implement them inline within bank.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Bank class. // =================================================================== account.h file #include class Account { public: Account(const std::string& name, double balance) : account_holder_(name), balance_(balance) {} const std::string& GetAccountHolder() const { return account_holder_; } double GetBalance() const { return balance_; } private:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
C++ Programming
main.cc file
#include <iostream> | |
#include <map> | |
#include <vector> | |
#include "bank.h" | |
int main() { | |
// =================== YOUR CODE HERE =================== | |
// 1. Create a Bank object, name it anything you'd like :) | |
// ======================================================= | |
// =================== YOUR CODE HERE =================== | |
// 2. Create 3 new accounts in your bank. | |
// * The 1st account should belong to "Tuffy", with | |
// a balance of $121.00 | |
// * The 2nd account should belong to "Frank", with | |
// a balance of $1234.56 | |
// * The 3nd account should belong to "Oreo", with | |
// a balance of $140.12 | |
// ======================================================= | |
// =================== YOUR CODE HERE =================== | |
// 3. Deactivate Tuffy's account. | |
// ======================================================= | |
// =================== YOUR CODE HERE =================== | |
// 4. Call DisplayBalances to print out all *active* | |
// account balances. | |
// ======================================================= | |
} |
bank.h file
#include <algorithm> | |
#include <cstdlib> | |
#include <iostream> | |
#include <map> | |
#include <memory> | |
#include <string> | |
#include "account.h" | |
class Bank { | |
public: | |
// ======================= YOUR CODE HERE ======================= | |
// Write the Bank class here. Refer to the README for the member | |
// variables, constructors, and member functions needed. | |
// | |
// Note: mark functions that do not modify the member variables | |
// as const, by writing `const` after the parameter list. | |
// Pass objects by const reference when appropriate. | |
// Remember that std::string is an object! | |
// =============================================================== | |
private: | |
// We provided this helper function to you to randomly generate | |
// a new Bank Account ID to be used in CreateAccount. | |
intGenerateAccountId() const { | |
returnstd::rand() % 9000 + 1000; // [1000, 9999] | |
} | |
}; |
bank.cc file
#include "bank.h" | |
// ========================= YOUR CODE HERE ========================= | |
// This implementation file (bank.cc) is where you should implement | |
// the member functions declared in the header (bank.h), only | |
// if you didn't implement them inline within bank.h. | |
// | |
// Remember to specify the name of the class with :: in this format: | |
// <return type> MyClassName::MyFunction() { | |
// ... | |
// } | |
// to tell the compiler that each function belongs to the Bank class. | |
// =================================================================== |
account.h file
#include <string>
class Account {
public:
Account(const std::string& name, double balance)
: account_holder_(name), balance_(balance) {}
const std::string& GetAccountHolder() const {
return account_holder_;
}
double GetBalance() const {
return balance_;
}
private:
std::string account_holder_;
double balance_;
};

Transcribed Image Text:DisplayBalances
Create a member function Display Balances, which accepts no input and returns nothing. This function should use an iterator object (please
use .begin() and .end()) to iterate through all key, value pairs in accounts, and print the details of each account to the console - the
account holder's name and the current balance in the following format:
Alice: $100.21
Bob: $2250.62
Carl: $50.25
Deactivate Account
Create a member function Deactivate Account, which accepts an int representing the ID of the account to be deactivated. This function should
remove the account from the map, if that account ID exists. You can use the std::map function find, which takes in a key and returns an
iterator pointing to that key if that key exists in the map, e.g.:
std::map<std::string, int> grades {{"JC", 98}, {"Paul", 97}, {"Carl", 78}};
grades.find("JC"); // returns an iterator pointing to {"JC", 98}
grades.find("Bob"); // returns grades.end(), since "Bob" is not in the map.
You can use the erase function we learned in lecture last week. The erase function for vectors works the same way as it does for a map:
// Removes the element pointed to by the iterator pos from the map
iterator std::map::erase(iterator pos);
For example,
std::map<std::string, int>::iterator it = grades.find("JC");
grades.erase(it);
Other instructions
Complete the main function as described. Place the Bank class in bank.h. Member functions that take more than ten lines or use complex
constructs should have their function prototype in the respective .h header file and implementation in the respective .cc implementation
file.
Sample Output:
Frank: $1234.56
Oreo: $140.12
![README.md
Insecure Bank Accounts
For this exercise you will create a Bank class that represents a bank that doesn't care about privacy. This bank allows anyone to create an
account, delete an account, and view all the accounts registered with the bank and their associated balances.
We've provided the Account class for you already. An Account represents an individual's bank account - which stores the name of the
account holder and the current balance in US dollars.
Bank Class
The Bank class stores all the accounts that have been created with this bank. It allows people to create and delete accounts with the bank, get
the total accounts that the bank currently has, and display every account holder's total balance in US Dollars.
Member Variables
Create the following private member variables:
1. bank_name_- a string representing the name of this bank.
2. accounts - a map whose key is an int representing the account ID, and whose value is the Account object representing the account
associated with that ID.
Constructor
Create one non-default constructor, which accepts a const reference to a string, which specifies the name of this bank.
Accessor Functions
Create two accessor functions for the two member variables. Please use these names for your accessors: GetBankName() and GetAccounts ().
Use const references where appropriate (i.e. to avoid expensive copies when returning large objects!)
Create Account
Create a member function createAccount, which accepts a const string reference representing the name of the account holder, and a double
for the initial balance of the account. Create Account should use these inputs to create a new Account object. Use the provided
GenerateAccountId() function to create a random ID between [1000, 9999] and use that as the new account's ID, and store the mapping from
that ID to the Account object into the accounts map.
TotalAccounts
Create a member function TotalAccounts, which accepts no input and returns the total number of accounts in the map.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7b779863-aa72-4537-9750-69366ddc9e6e%2F183a0d67-8bd1-4c06-865c-0cdca4568f36%2F0v9imlzm_processed.png&w=3840&q=75)
Transcribed Image Text:README.md
Insecure Bank Accounts
For this exercise you will create a Bank class that represents a bank that doesn't care about privacy. This bank allows anyone to create an
account, delete an account, and view all the accounts registered with the bank and their associated balances.
We've provided the Account class for you already. An Account represents an individual's bank account - which stores the name of the
account holder and the current balance in US dollars.
Bank Class
The Bank class stores all the accounts that have been created with this bank. It allows people to create and delete accounts with the bank, get
the total accounts that the bank currently has, and display every account holder's total balance in US Dollars.
Member Variables
Create the following private member variables:
1. bank_name_- a string representing the name of this bank.
2. accounts - a map whose key is an int representing the account ID, and whose value is the Account object representing the account
associated with that ID.
Constructor
Create one non-default constructor, which accepts a const reference to a string, which specifies the name of this bank.
Accessor Functions
Create two accessor functions for the two member variables. Please use these names for your accessors: GetBankName() and GetAccounts ().
Use const references where appropriate (i.e. to avoid expensive copies when returning large objects!)
Create Account
Create a member function createAccount, which accepts a const string reference representing the name of the account holder, and a double
for the initial balance of the account. Create Account should use these inputs to create a new Account object. Use the provided
GenerateAccountId() function to create a random ID between [1000, 9999] and use that as the new account's ID, and store the mapping from
that ID to the Account object into the accounts map.
TotalAccounts
Create a member function TotalAccounts, which accepts no input and returns the total number of accounts in the map.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
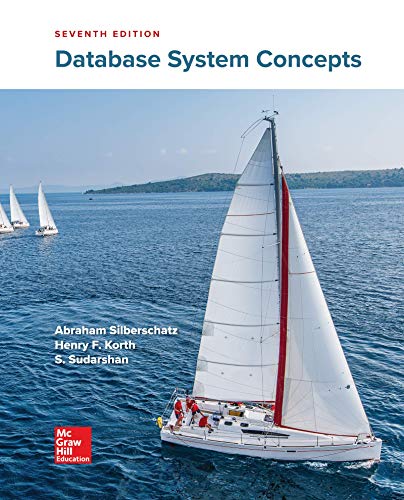
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
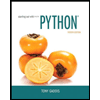
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
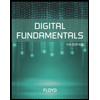
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
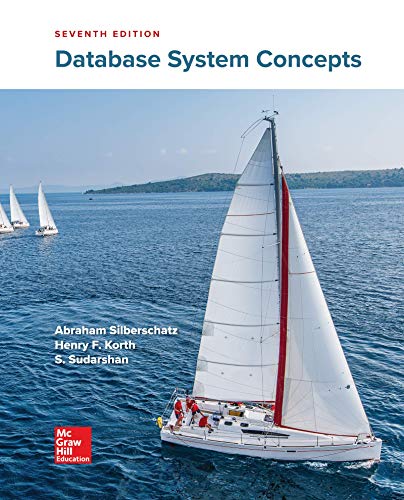
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
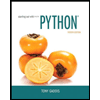
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
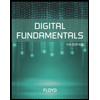
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
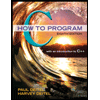
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
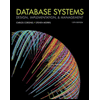
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
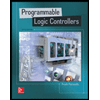
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education