#include #include #include #include using namespace std::chrono; using namespace std; void randomVector(int vector[], int size) { for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; } } int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast(stop - start); cout << "Time taken by function: " << duration.count() << " microseconds" << endl; return 0;
#include <iostream>
#include <cstdlib>
#include <time.h>
#include <chrono>
using namespace std::chrono;
using namespace std;
void randomVector(int
{
for (int i = 0; i < size; i++)
{
//ToDo: Add Comment
vector[i] = rand() % 100;
}
}
int main(){
unsigned long size = 100000000;
srand(time(0));
int *v1, *v2, *v3;
//ToDo: Add Comment
auto start = high_resolution_clock::now();
//ToDo: Add Comment
v1 = (int *) malloc(size * sizeof(int *));
v2 = (int *) malloc(size * sizeof(int *));
v3 = (int *) malloc(size * sizeof(int *));
randomVector(v1, size);
randomVector(v2, size);
//ToDo: Add Comment
for (int i = 0; i < size; i++)
{
v3[i] = v1[i] + v2[i];
}
auto stop = high_resolution_clock::now();
//ToDo: Add Comment
auto duration = duration_cast<microseconds>(stop - start);
cout << "Time taken by function: "
<< duration.count() << " microseconds" << endl;
return 0;
}
Please answer the below answers theoritically and not in a code form.
1. Add the default(none)attribute to the #pargma omp parallel directive. Compile and run your code.
If you get any compilation error, try to identify the reason. As required, add any of the shared,
private or firstprivate attributes to fix the compilation error. Try different variations of data
sharing (e.g. shared(size) private(v1) or private(size) shared(v1) or ...). Is the outcome
of your program different? Explain why.
2. Compute the total sum of all the elements in v3 using a shared variable called total and atomic
update directive.
3. Use the reduction clause to compute the total sum of all the elements in v3.
4. Implement an alternative version where each thread computes its own part to a private variable and
then use a critical section (#pragma omp critical) after the loop to calculate the total sum. Do you
get the exact same results in all cases?
5. Try different OpenMP Scheduling techniques by adding schedule(type[,chunk]) attribute to
#pargma omp for directive. Experiment with the chunk size to understand how each Scheduling
technique works. Does changing the scheduling techniques or chunk size impact the execution time.
Briefly explain your observations.

Step by step
Solved in 5 steps with 3 images

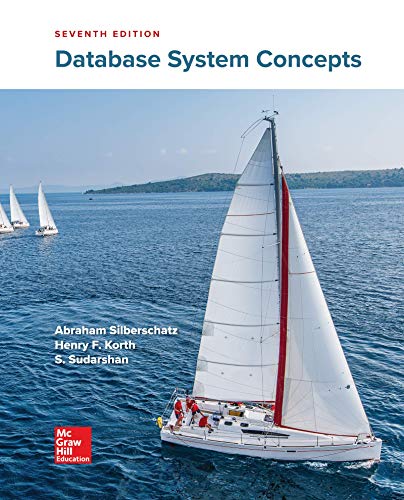
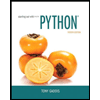
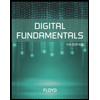
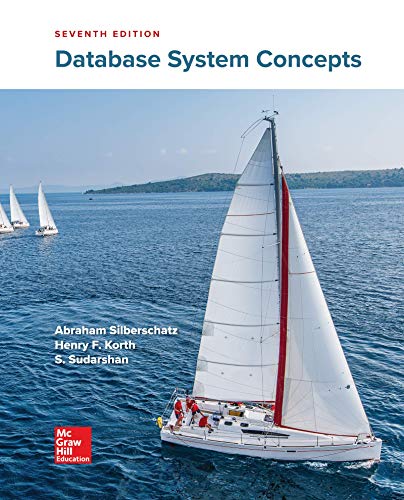
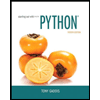
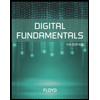
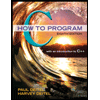
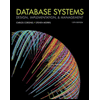
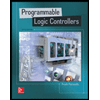