Main.cpp #include #include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0; } Card.h #ifndef CARD_H #define CARD_H class Card { public: Card(); Card(char r, char s); int getValue(); void showCard(); private: char rank; char suit; }; #endif Card.cpp #include "Card.h" #include Card::Card() {} Card::Card(char r, char s) { rank = r; suit = s; } int Card::getValue() { // Implement the logic to assign values to each rank of the card // and return the corresponding value return 0; } void Card::showCard() { // Display the rank and suit of the card std::cout << rank << suit; } arrow_forward Step 3: Deck.h and Deck.cpp Deck.h #ifndef DECK_H #define DECK_H #include "Card.h" class Deck { public: Deck(); void refreshDeck(); Card Deal(); void shuffle(); int cardsLeft(); void showDeck(); private: Card cards[52]; int numCards; }; #endif Deck.cpp #include "Deck.h" #include #include #include Deck::Deck() { numCards = 52; refreshDeck(); } void Deck::refreshDeck() { char ranks[] = {'A', '2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K'}; char suits[] = {'H', 'D', 'C', 'S'}; int index = 0; for (int suit = 0; suit < 4; suit++) { for (int rank = 0; rank < 13; rank++) { cards[index] = Card(ranks[rank], suits[suit]); index++; } } } Card Deck::Deal() { if (numCards > 0) { numCards--; return cards[numCards]; } return Card(); } void Deck::shuffle() { std::srand(static_cast(std::time(0))); for (int i = numCards - 1; i > 0; i--) { int j = std::rand() % (i + 1); std::swap(cards[i], cards[j]); } } int Deck::cardsLeft() { return numCards; } void Deck::showDeck() { for (int i = 0; i < numCards; i++) { cards[i].showCard(); std::cout << " "; } std::cout << std::endl; } UML Class Diagrams: A class diagram for each class with appropriate symbols for composition/aggregation and inheritance.
Main.cpp
#include <iostream>
#include "Deck.h"
int main() {
Deck deck;
deck.shuffle();
std::cout << "WAR Card Game\n\n";
std::cout << "Dealing cards...\n\n";
Card player1Card = deck.Deal();
Card player2Card = deck.Deal();
std::cout << "Player 1's card: ";
player1Card.showCard();
std::cout << std::endl;
std::cout << "Player 2's card: ";
player2Card.showCard();
std::cout << std::endl;
int player1Value = player1Card.getValue();
int player2Value = player2Card.getValue();
if (player1Value > player2Value) {
std::cout << "Player 1 wins!" << std::endl;
} else if (player1Value < player2Value) {
std::cout << "Player 2 wins!" << std::endl;
} else {
std::cout << "It's a tie!" << std::endl;
}
return 0;
}
Card.h
#ifndef CARD_H
#define CARD_H
class Card {
public:
Card();
Card(char r, char s);
int getValue();
void showCard();
private:
char rank;
char suit;
};
#endif
Card.cpp
#include "Card.h"
#include <iostream>
Card::Card() {}
Card::Card(char r, char s) {
rank = r;
suit = s;
}
int Card::getValue() {
// Implement the logic to assign values to each rank of the card
// and return the corresponding value
return 0;
}
void Card::showCard() {
// Display the rank and suit of the card
std::cout << rank << suit;
}
Deck.h
#ifndef DECK_H
#define DECK_H
#include "Card.h"
class Deck {
public:
Deck();
void refreshDeck();
Card Deal();
void shuffle();
int cardsLeft();
void showDeck();
private:
Card cards[52];
int numCards;
};
#endif
Deck.cpp
#include "Deck.h"
#include <iostream>
#include <cstdlib>
#include <ctime>
Deck::Deck() {
numCards = 52;
refreshDeck();
}
void Deck::refreshDeck() {
char ranks[] = {'A', '2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K'};
char suits[] = {'H', 'D', 'C', 'S'};
int index = 0;
for (int suit = 0; suit < 4; suit++) {
for (int rank = 0; rank < 13; rank++) {
cards[index] = Card(ranks[rank], suits[suit]);
index++;
}
}
}
Card Deck::Deal() {
if (numCards > 0) {
numCards--;
return cards[numCards];
}
return Card();
}
void Deck::shuffle() {
std::srand(static_cast<unsigned int>(std::time(0)));
for (int i = numCards - 1; i > 0; i--) {
int j = std::rand() % (i + 1);
std::swap(cards[i], cards[j]);
}
}
int Deck::cardsLeft() {
return numCards;
}
void Deck::showDeck() {
for (int i = 0; i < numCards; i++) {
cards[i].showCard();
std::cout << " ";
}
std::cout << std::endl;
}
UML Class Diagrams: A class diagram for each class with appropriate symbols for composition/aggregation and inheritance.

Step by step
Solved in 3 steps

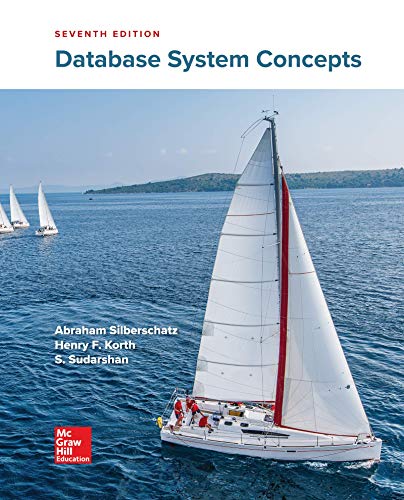
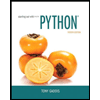
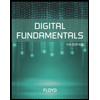
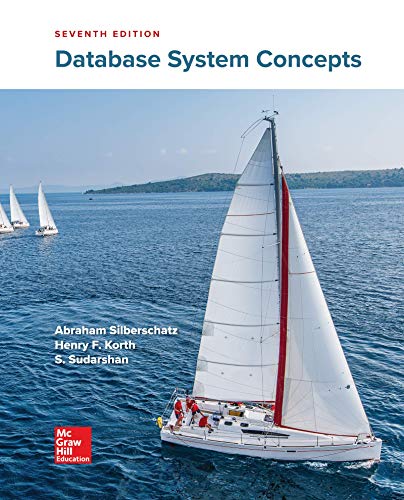
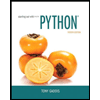
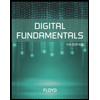
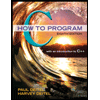
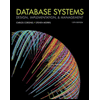
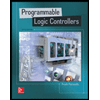