In C++Take the following code but -for the first option, change it so that instead of the middle element, its the first element -for the third option, change it so that instead of the middle element
In C++Take the following code but
-for the first option, change it so that instead of the middle element, its the first element
-for the third option, change it so that instead of the middle element its the first element
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
// this funtion is used to partition the arry based on given pivot
//this funtion also takes an string opt(it indicates pivot type) so if type is pivot type is middle element then it selects middle element as pivot
//and if pivot type is median element then it selects median element as pivot
int Partition(int a[], int low, int high, string opt)
{
int pivot, ind = low, i, swp;
//for type-1 anf type-3 sort we use the middle element as pivot
if (opt == "Type1 sort" || opt == "Type3 sort") {
pivot = (high + low) / 2;
}
//for type-2 anf type-4 sort we use the median element as pivot
else if (opt == "Type2 sort" || opt == "Type4 sort")
{
pivot = (high + low) / 2;
if ((a[high] >= a[pivot] && a[high] <= a[low]) || (a[high] >= a[low] && a[high] <= a[pivot]))
pivot = high;
else if ((a[low] >= a[pivot] && a[low] <= a[high]) || (a[low] >= a[high] && a[low] <= a[pivot]))
pivot = low;
}
swp = a[pivot];
a[pivot] = a[high];
a[high] = swp;
pivot = high;
/*Getting ind of the pivot.*/
for (i = low; i < high; i++) {
if (a[i] < a[pivot]) {
swp = a[i];
a[i] = a[ind];
a[ind] = swp;
ind++;
}
}
swp = a[pivot];
a[pivot] = a[ind];
a[ind] = swp;
return ind;
}
void insertion_sort(int a[], int low, int high)
{
int swp, ind;
for (int i = low + 1; i <= high; i++) {
swp = a[i];
ind = i;
for (int j = i - 1; j >= low; j--) {
if (swp < a[j]) {
a[j + 1] = a[j];
ind = j;
}
else
break;
}
a[ind] = swp;
}
}
void quick_sort(int a[], int low, int high, string opt)
{
int pindex;
//for type-3 and type-4 sort if size is less than 20 then we apply insertion sort
if ((opt == "Type3 sort" || opt == "Type4 sort") && high - low < 19)
{
insertion_sort(a, low, high);
}
//recursive quick sort
else if (low < high) {
//find the partition
pindex = Partition(a, low, high, opt);
//recursive call for left and right part of array
quick_sort(a, low, pindex - 1, opt);
quick_sort(a, pindex + 1, high, opt);
}
return;
}
int arr[1000000];
int original[1000000];
int main() {
srand(time(NULL));
//this loop breaks when user enters choice N
char choice;
while (1)
{
int n, i;
cout << "Enter array size to be sorted: ";
cin >> n;
//create a array of random numbers in range 0 to 100000
//store this array into original array and then copy it to arr[] to perform four different sorting
for (i = 0; i < n; i++)
original[i] = (rand() % 100000) + 1;
//before performing each type of sort we fill the arr[] with original array
//type-1 sort (pivot middle element)
for (i = 0; i < n; i++)
arr[i] = original[i];
clock_t t1, t2;
t1 = clock();
quick_sort(arr, 0, n - 1, "Type1 sort");
t2 = clock();
for (i = 0; i < n; i++)
arr[i] = original[i];
cout << "quick sort time, with pivot middle element:" << (t2 - t1) * 1000 / (CLOCKS_PER_SEC);
cout << "\n";
//type-2 sort (with pivot median element)
for (i = 0; i < n; i++)
arr[i] = original[i];
t1 = clock();
quick_sort(arr, 0, n - 1, "Type2 sort");
t2 = clock();
cout << "Quick sort time, with pivot median element:" << (t2 - t1) * 1000 / (CLOCKS_PER_SEC);
cout << "\n";
//type-3 sort(insertion + quick sort (pivot middle element))
for (i = 0; i < n; i++)
arr[i] = original[i];
t1 = clock();
quick_sort(arr, 0, n - 1, "Type3 sort");
t2 = clock();
cout << "Quick sort time and insertion sort time, with pivot middle element:" << (t2 - t1) * 1000 / (CLOCKS_PER_SEC);
cout << "\n";
////type-4 sort(insertion + quick sort (pivot median element))
for (i = 0; i < n; i++)
arr[i] = original[i];
t1 = clock();
quick_sort(arr, 0, n - 1, "Type4 sort");
t2 = clock();
cout << "Quick sort time and insertion sort time, with pivot median element:" << (t2 - t1) * 1000 / (CLOCKS_PER_SEC);
cout << "\n";
cout << "Press N to exit, press any other key to enter another array size" << endl;
cin >> choice;
if (choice == 'N' || choice =='n')
break;
else
continue;
}
return 0;
}

Step by step
Solved in 2 steps with 2 images

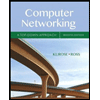
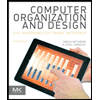
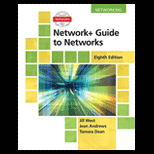
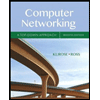
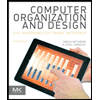
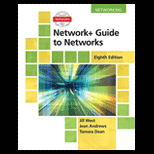
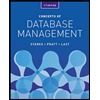
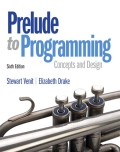
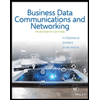