Modify the existing vector's contents, by erasing the element at index 1 (initially 200), then inserting 100 and 102 in the shown locations. Use Vector ADT's erase() and insert() only, and remember that the first argument of those functions is special, involving an iterator and not just an integer. Sample output of below program with input 33 200 10: #include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) {unsigned int i; for (i = 0; i < numsList.size(); ++i) {cout << numsList.at(i) << " ";}cout << endl;} int main() {vector<int> numsList;int userInput;int i; for (i = 0; i < 3; ++i) {cin >> userInput;numsList.push_back(userInput);} /* Your solution goes here */ PrintVectors(numsList); return 0;} Please help me with this problem using c++.
Modify the existing
#include <iostream>
#include <vector>
using namespace std;
void PrintVectors(vector<int> numsList) {
unsigned int i;
for (i = 0; i < numsList.size(); ++i) {
cout << numsList.at(i) << " ";
}
cout << endl;
}
int main() {
vector<int> numsList;
int userInput;
int i;
for (i = 0; i < 3; ++i) {
cin >> userInput;
numsList.push_back(userInput);
}
/* Your solution goes here */
PrintVectors(numsList);
return 0;
}
Please help me with this problem using c++.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

#include <iostream>
#include <
using namespace std;
void PrintVectors(vector<int> numsList) {
unsigned int i;
for (i = 0; i < numsList.size(); ++i) {
cout << numsList.at(i) << " ";
}
cout << endl;
}
int main() {
vector<int> numsList;
int userInput;
int i;
for (i = 0; i < 3; ++i) {
cin >> userInput;
numsList.push_back(userInput);
}
numsList.erase(numsList.begin()+1);
numsList.insert(numsList.begin()+1, 102);
numsList.insert(numsList.begin()+1, 100);
PrintVectors(numsList);
return 0;
}
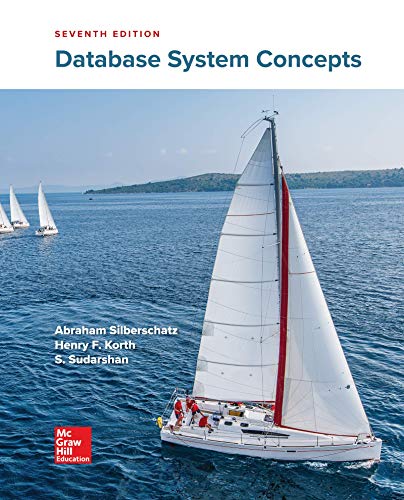
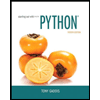
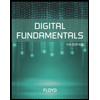
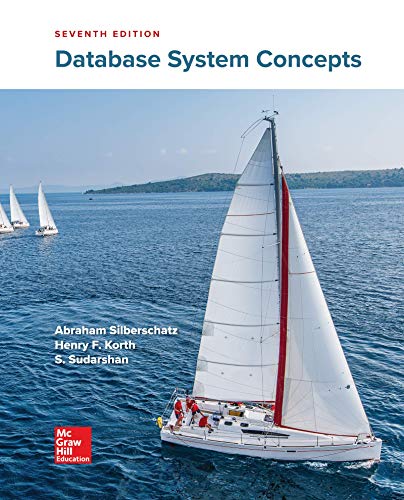
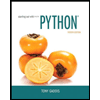
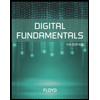
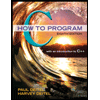
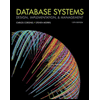
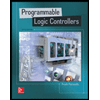