C++ language Alter the code found in main.cpp Using the file, add/change the code in the file, but only where indicating you can add or change code. main.cpp #include using namespace std; /* The function binarySearch accepts a sorted array data with no duplicates, and the range within that array to search, defined by first and last
C++ language
Alter the code found in main.cpp
Using the file, add/change the code in the file, but only where indicating you can add or change code.
main.cpp
#include <iostream>
using namespace std;
/*
The function binarySearch accepts a sorted array data with no duplicates,
and the range within that array to search, defined by first and last.
Finally, goal is the value that is searched for within the array.
If the goal can be found within the array, the function returns the
index position of the goal value in the array. If the goal value does
not exist in the array, the function returns -1.
*/
int binarySearch(int data[], int first, int last, int goal)
{
cout << "first: " << first << ", last: " << last << endl;
// YOU CAN ONLY ADD OR CHANGE CODE BELOW THIS COMMENT
return -1;
// YOU CAN ONLY ADD OR CHANGE CODE ABOVE THIS COMMENT
}
int main()
{
const int ARRAY_SIZE = 20;
int searchValue;
/* generates an array data that contains:
0, 10, 20, 30, .... 170, 180, 190
*/
int data[ARRAY_SIZE];
for(int i = 0; i < ARRAY_SIZE; i++)
data[i] = i * 10;
cout << "Enter Search Value: ";
cin >> searchValue;
int answer = binarySearch(data, 0, ARRAY_SIZE-1, searchValue);
cout << "Answer: " << answer << endl;
return 0;
Create a function binarySearch that accepts 4 inputs:
- data[] - A sorted (low to high) array of integers with no duplicates.
- first - the smallest index position within the array that should be searched.
- last - the largest index position within the array that should be searched.
- goal - the value we are searching for within the array.
The function will return the index position of the value goal in the array. If the value goal does not exist in the array, the function returns -1. You must use recursion to solve this problem efficiently.
Sample Output:
Enter Search Value:
165
first: 0, last: 19
first: 10, last: 19
first: 15, last: 19
first: 15, last: 16
first: 16, last: 16
first: 17, last: 16
Answer: -1
[cathy]$ ./a.out
Enter Search Value: 40
first: 0, last: 19
first: 0, last: 8
Answer: 4
[cathy]$ ./a.out
Enter Search Value: 10
first: 0, last: 19
first: 0, last: 8
first: 0, last: 3
Answer: 1

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

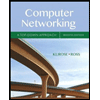
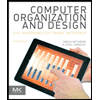
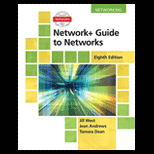
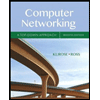
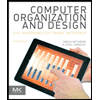
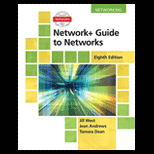
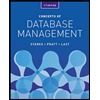
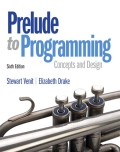
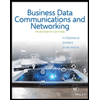