Smoothie constructor should accept a list of ingredients. • Should not be empty => throw ArgumentException error o Ingredients should not be repeated => throw ArgumentException error o Ingredients that doesn't exist (ingredients are CASE SENSITIVE) => throw ArgumentException error • Ingredients should not be null => throw Argument NullException error Implement Ingredients Property o Will return a list of all supplied ingredients in the constructor o Ingredients should be in alphabetical order A->Z o Users should NOT be able to set Ingredients explicitly smoothie. Ingredients = new List {"Bananana"}; X Implement Name Property gets the ingredients and puts them in alphabetical order into a nice descriptive sentence. If there are multiple ingredients, add the word "Fusion" to the end but otherwise, add "Smoothie". Remember to change "-berries" to "-berry". See the examples below. o Users should NOT be able to set Name explicitly smoothie.Name = "IllegalName"; X Implement GetCost method which calculates the total cost of the ingredients used to make the smoothie o Round to two decimal places. Implement GetPrice method which returns the number from GetCost plus the number from GetCost multiplied by 1.5. • Round to two decimal places. Ingredient Price Strawberries Banana $1.50 $0.50 Mango Blueberries $1.00 $2.50 Raspberries Apple Pineapple $3.50 $1.00 $1.75
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
C#
Please see the attached photo for the instructions
Complete this code:
namespace Smoothies
{
public class Smoothie
{
// DO NOT MODIFY THIS FIELD
private readonly Dictionary<string, string> _prices = new()
{
{ "Strawberries", "$1.50" },
{ "Banana", "$0.50" },
{ "Mango", "$2.50" },
{ "Blueberries", "$1.00" },
{ "Raspberries", "$1.00" },
{ "Apple", "$1.75" },
{ "Pineapple", "$3.50" }
};
// TODO: Implement me!
public Smoothie (IEnumerable<string> ingredients)
{
throw new NotImplementedException();
}
/// <summary>
/// Ingredients used for the smoothie
/// TODO: Implement me!
/// </summary>
public IEnumerable<string> Ingredients
{
get => throw new NotImplementedException();
set => throw new NotImplementedException();
}
/// <summary>
/// Name of the smoothie
/// TODO: Implement me!
/// </summary>
public string Name
{
get => throw new NotImplementedException();
set => throw new NotImplementedException();
}
/// <summary>
/// Method which calculates the total cost of the ingredients used to make the smoothie.
/// TODO: Implement me!
/// </summary>
public string GetCost()
{
throw new NotImplementedException();
}
/// <summary>
/// Method which returns the number from <c>GetCost() + (GetCost() x 1.5)</c>. Round to two decimal places.
/// TODO: Implement me!
/// </summary>
public string GetPrice()
{
throw new NotImplementedException();
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

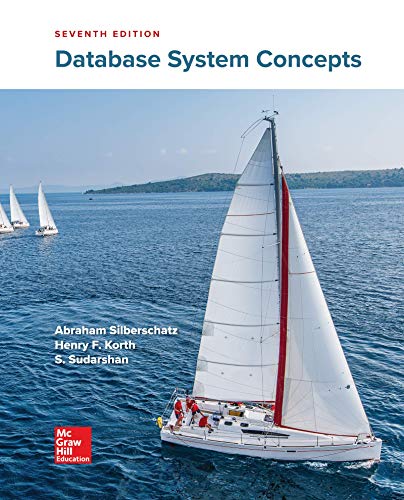
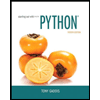
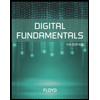
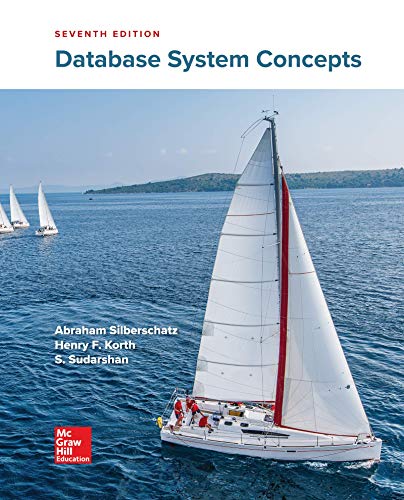
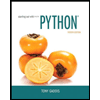
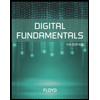
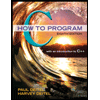
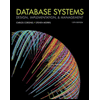
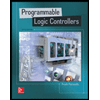