public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; // Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; } // Mutator for itemName public void setName(String itemName) { this.itemName = itemName; } // Accessor for itemName public String getName() { return itemName; } // Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } // Accessor for itemPrice public int getPrice() { return itemPrice; } // Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } // Accessor for itemQuantity public int getQuantity() { return itemQuantity; } } import java.util.Scanner; public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); // Creating instances of ItemToPurchase ItemToPurchase item1 = new ItemToPurchase(); ItemToPurchase item2 = new ItemToPurchase(); // Setting properties for item1 item1.setName("Laptop"); item1.setPrice(800); item1.setQuantity(2); // Setting properties for item2 item2.setName("Mouse"); item2.setPrice(20); item2.setQuantity(5); // Displaying item information System.out.println("Item 1:"); System.out.println("Name: " + item1.getName()); System.out.println("Price: $" + item1.getPrice()); System.out.println("Quantity: " + item1.getQuantity()); System.out.println("Total Cost: $" + item1.getPrice() * item1.getQuantity()); System.out.println(); System.out.println("Item 2:"); System.out.println("Name: " + item2.getName()); System.out.println("Price: $" + item2.getPrice()); System.out.println("Quantity: " + item2.getQuantity()); System.out.println("Total Cost: $" + item2.getPrice() * item2.getQuantity()); } }
public class ItemToPurchase {
private String itemName;
private int itemPrice;
private int itemQuantity;
// Default constructor
public ItemToPurchase() {
itemName = "none";
itemPrice = 0;
itemQuantity = 0;
}
// Mutator for itemName
public void setName(String itemName) {
this.itemName = itemName;
}
// Accessor for itemName
public String getName() {
return itemName;
}
// Mutator for itemPrice
public void setPrice(int itemPrice) {
this.itemPrice = itemPrice;
}
// Accessor for itemPrice
public int getPrice() {
return itemPrice;
}
// Mutator for itemQuantity
public void setQuantity(int itemQuantity) {
this.itemQuantity = itemQuantity;
}
// Accessor for itemQuantity
public int getQuantity() {
return itemQuantity;
}
}
import java.util.Scanner;
public class ShoppingCartPrinter {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
// Creating instances of ItemToPurchase
ItemToPurchase item1 = new ItemToPurchase();
ItemToPurchase item2 = new ItemToPurchase();
// Setting properties for item1
item1.setName("Laptop");
item1.setPrice(800);
item1.setQuantity(2);
// Setting properties for item2
item2.setName("Mouse");
item2.setPrice(20);
item2.setQuantity(5);
// Displaying item information
System.out.println("Item 1:");
System.out.println("Name: " + item1.getName());
System.out.println("Price: $" + item1.getPrice());
System.out.println("Quantity: " + item1.getQuantity());
System.out.println("Total Cost: $" + item1.getPrice() * item1.getQuantity());
System.out.println();
System.out.println("Item 2:");
System.out.println("Name: " + item2.getName());
System.out.println("Price: $" + item2.getPrice());
System.out.println("Quantity: " + item2.getQuantity());
System.out.println("Total Cost: $" + item2.getPrice() * item2.getQuantity());
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

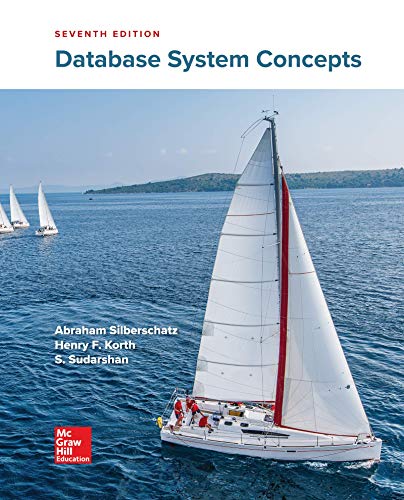
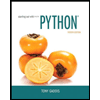
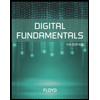
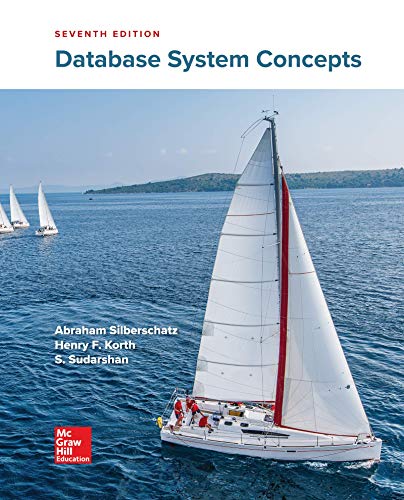
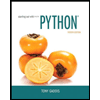
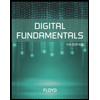
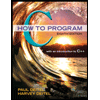
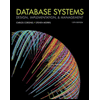
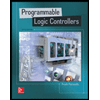