In C++ class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setQuantityInStock (int noOfCopies); //sets the private member quantityInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the quantityInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantityInStock void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original value plus the parameter sent to this function private: string bookTitle; string bookISBN; double bookPrice; int quantityInStock; }; Write the full function definition for updateQuantity();
In C++
class bookType
{
public:
void setBookTitle(string s); //sets the bookTitle to s
void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter
void setBookPrice(double cost); //sets the private member bookPrice to cost
void setQuantityInStock (int noOfCopies); //sets the private member quantityInStock to noOfCopies
void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the quantityInStock
string getBookISBN() const; //returns the bookISBN
double getBookPrice() const; //returns the bookPrice
int showQuantityInStock() const; //returns the quantityInStock
void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original value plus the parameter sent to this function
private:
string bookTitle;
string bookISBN;
double bookPrice;
int quantityInStock;
};
Write the full function definition for updateQuantity();

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

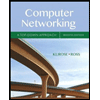
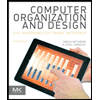
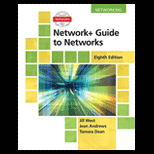
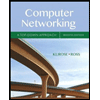
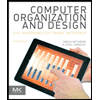
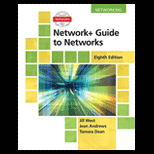
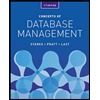
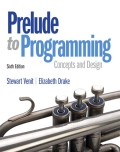
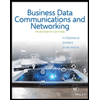