Given the following class: class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setCopiesInStock(int noOfCopies); //sets the private member copiesInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the copiesInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantity in stock void updateQuantity(int addBooks); // adds addBooks to the quantityInStock, so that the quantity // in stock now has it original value plus the parameter sent to this function private: string bookName; string bookISBN; double price; int quantity; }; Write the FULL FUNCTION DEFINTION (that means, HEADER and BODY) for the DEFAULT CONSTRUCTOR for the Class, bookType, described above.
Given the following class:
class bookType
{ public:
void setBookTitle(string s);
//sets the bookTitle to s
void setBookISBN(string ISBN);
//sets the private member bookISBN to the parameter
void setBookPrice(double cost);
//sets the private member bookPrice to cost
void setCopiesInStock(int noOfCopies);
//sets the private member copiesInStock to noOfCopies
void printInfo() const;
//prints the bookTitle, bookISBN, the bookPrice and the copiesInStock
string getBookISBN() const;
//returns the bookISBN
double getBookPrice() const;
//returns the bookPrice
int showQuantityInStock() const;
//returns the quantity in stock
void updateQuantity(int addBooks);
// adds addBooks to the quantityInStock, so that the quantity
// in stock now has it original value plus the parameter sent to this function
private:
string bookName;
string bookISBN;
double price;
int quantity;
};
Write the FULL FUNCTION DEFINTION (that means, HEADER and BODY) for the DEFAULT CONSTRUCTOR for the Class, bookType, described above.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

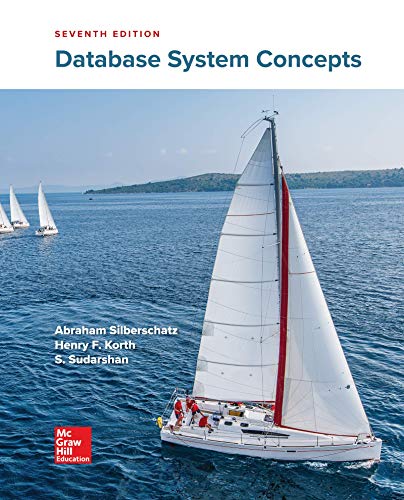
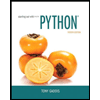
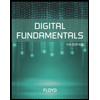
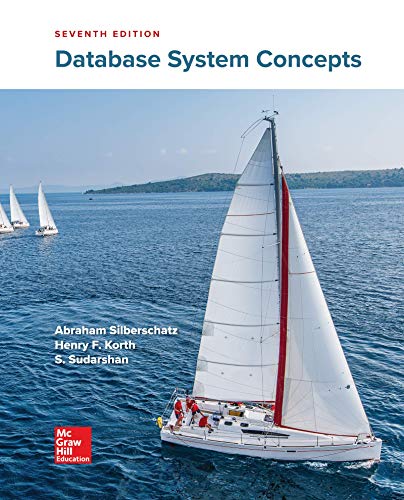
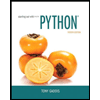
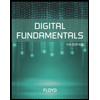
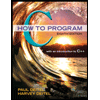
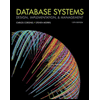
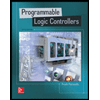