Conver the class To UML diagram class Clint { private String accNumber; private String holderName; private long currBalance; public Clint() { currBalance = 0; } public Clint(String acc, String name, long bal) { accNumber = acc;
Conver the class To UML diagram
class Clint {
private String accNumber;
private String holderName;
private long currBalance;
public Clint() {
currBalance = 0;
}
public Clint(String acc, String name, long bal) {
accNumber = acc;
holderName = name;
currBalance = bal;
}
Scanner sc = new Scanner(System.in);
// deposit money into the bank account by given amount amt
public void deposit(long amt) {
currBalance += amt;
}
// if amount is not given ask the user for the amount
public void deposit() {
System.out.print("Enter Amount(to deposit): $");
long amt = sc.nextLong();
currBalance += amt;
}
// withdraw money from the bank account
public void withdraw(long amt) {
if (currBalance >= amt) {
currBalance -= amt;
} else {
System.out.println("Insufficient funds!");
}
}
// if amt is not given ask the user for the amount
public void withdraw() {
System.out.print("Enter Amount(to withdraw): $");
long amt = sc.nextLong();
if (currBalance >= amt) {
currBalance -= amt;
} else {
System.out.println("Insufficient funds!");
}
}
// getters and setters for all the private members
public String getAccNumber() {
return accNumber;
}
public String getHolderName() {
return holderName;
}
public long getBalance() {
return currBalance;
}
public void setBalance(long amt) {
currBalance = amt;
}
public void setHolderName(String n) {
holderName = n;
}
public void setAccNumber(String acc) {
accNumber = acc;
}
// method to display account details
public void printDetails() {
System.out.println("[" + accNumber + "-" + holderName + ": $" + currBalance + "]");
}
}
import java.util.ArrayList;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author SAYTECH
*/
class Account {
private String bankName;
private ArrayList<Clint> customers;
// default constructor
public Account() {
bankName = "NA";
customers = new ArrayList<Clint>();
}
// parameterized constructor
public Account(String name) {
bankName = name;
customers = new ArrayList<Clint>();
}
// method inserts new BankAccount in the Bank
public void insert(Clint acc) {
customers.add(acc);
}
// this method searches for the accNo and if present deletes it
public void delete(String accNo) {
// delete the bank account associated with the given accNo
boolean found = false;
for (int i = 0; i < customers.size(); i++) {
if (customers.get(i).getAccNumber().equals(accNo)) {
// found the account
found = true;
customers.remove(i);
break;
}
}
if (found) {
System.out.println("Found and Deleted!");
} else {
System.out.println("No such account exists in the bank!");
}
}
// prints all the details of the bank
public void printDetails() {
System.out.println(bankName + " has " + customers.size() + " customers.\nBelow are the deatils:");
for (Clint acc : customers) {
System.out.println("[" + acc.getAccNumber() + "-" + acc.getHolderName() + ": $" + acc.getBalance() + "]");
}
}
}

Step by step
Solved in 6 steps with 1 images

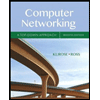
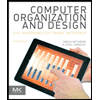
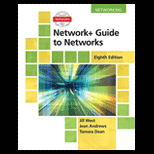
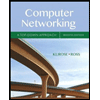
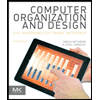
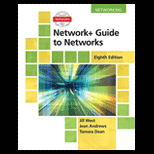
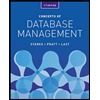
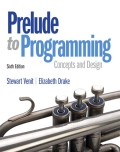
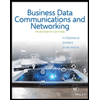