class Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could I remove the printStats() member function from BasketballPlayer? Why or why not? d. Would the following line in main() compile: Player *ptr; -- why or why not? e. Write code which creates a Player pointer (e.g. Player *p), creates an object of class BasketballPlayer, and assigns the address of the object to the pointer. Why does this work?
class Player
{
protected:
string name;
double weight;
double height;
public:
Player(string n, double w, double h)
{ name = n; weight = w; height = h; }
string getName() const
{ return name; }
virtual void printStats() const = 0;
};
class BasketballPlayer : public Player
{
private:
int fieldgoals;
int attempts;
public:
BasketballPlayer(string n, double w,
double h, int fg, int a) : Player(n, w, h)
{ fieldgoals = fg; attempts = a; }
void printStats() const
{ cout << name << endl;
cout << "Weight: " << weight;
cout << " Height: " << height << endl;
cout << "FG: " << fieldgoals;
cout << " attempts: " << attempts;
cout << " Pct: " << (double) fieldgoals / attempts << endl; }
};
a. What does = 0 after function printStats() do?
b. Would the following line in main() compile: Player p; -- why or why not?
c. Could I remove the printStats() member function from BasketballPlayer? Why or why not?
d. Would the following line in main() compile: Player *ptr; -- why or why not?
e. Write code which creates a Player pointer (e.g. Player *p), creates an object of class BasketballPlayer, and assigns the address of the object to the pointer. Why does this work?

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

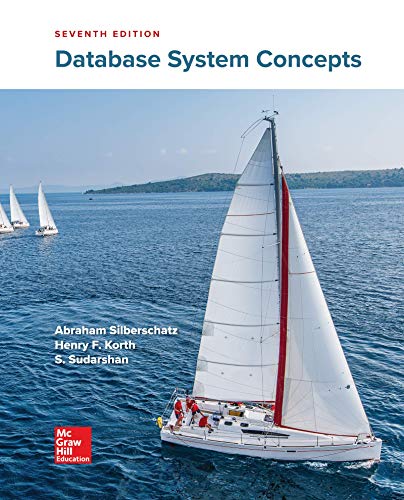
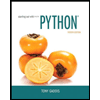
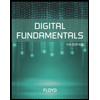
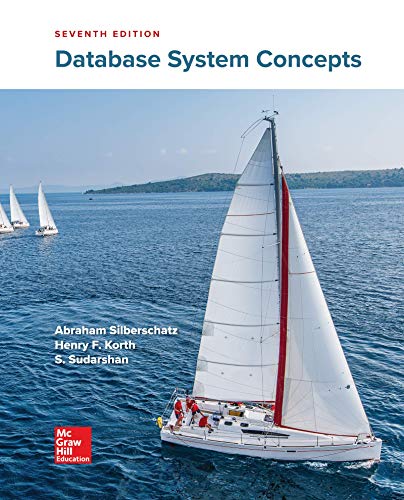
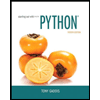
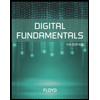
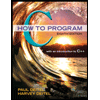
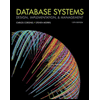
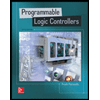