1 Description of the Program In this assignment, you will make two classes, FullTime Employee and PartTimeEmployee, that inherit from a superclass Employee. The implementation of class Employee is given. You will also need to write a test program to test the methods you write for these two classes. The implementation details are described as follows. Stage1: In the first file Full TimeEmployee.java, you should include the following addi- tional instance variables and methods (other than all instance variables and methods inher- ited from class Employee): Private instance variable weeklySalary: • A constructor takes four inputs (firstname, lastname, SSN and salary); • One additional getter method to return the instance variable (accessor); One setter method to set the instance variable (mutator); • One overriding method earnings () that returns the weekly earnings (should be as same as the getSalary() method); A method toString that converts an fulltime employee's information into string form (including firstname, last name, ssn, earning and class name). The string should have a neat output format as shown in Figure 1. You should override superclass toString() method. Stage2: In the second file Part Time Employee.java, you should include the following addi- tional instance variables and methods (other than all instance variables and methods inher- ited from class Employee): Private instance variables wage (wage per hour) and hours (hours worked for week); • A constructor takes five inputs: firstname, firstname, SSN, hours, and wage; Two additional getter methods to return the instance variables (accessor); • Two setter methods to set the instance variables (mutator);
Go off this code:
public abstract class Employee
{
private String firstName;
private String lastName;
private String SSN;
double salary;
public Employee( String first, String last, String ssn )
{
firstName = first;
lastName = last;
SSN = ssn;
}
public void setfirstName( String first )
{
firstName = first; // should validate
}
public String getfirstName()
{
return firstName;
}
public void setlastName( String last )
{
lastName = last; // should validate
}
public String getlastName()
{
return lastName;
}
public void setSSN( String ssn )
{
this.SSN = ssn;
}
public String getSSN()
{
return SSN;
}
@Override
public String toString()
{
return String.format( "%-12s%-12s%-12s", getfirstName(), getlastName(), getSSN() );
}
public abstract double earnings();
}



As per the given question, given the Employee class, we need to implement FullTimeEmployee, PartTimeEmployee, and EmployeeTester classes.
Both FullTimeEmployee and PartTimeEmployee classes inherit the Employee class.
Inside the EmployeeTester class, create an array of Employee class objects and then based on the first value in each line of the file.
Step by step
Solved in 6 steps with 5 images

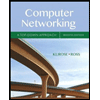
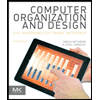
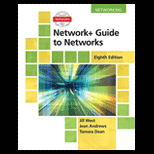
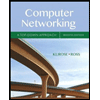
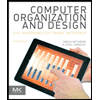
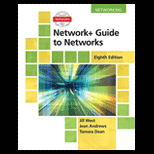
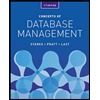
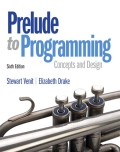
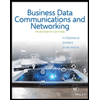