package assignment; public class Circle2D2 { //data fields specifying the center of the circle private double x, y; //data field radius private double radius; //default circle with (0, 0) for (x, y) and 1 for radius Circle2D2() { this(0, 0, 1); } //circle with the specified x, y, and radius Circle2D2(double x, double y, double radius) { this.x = x; this.y = y; this.radius = radius; } //return x public double getX() { return x; } //return y public double getY() { return y; } //return radius public double getRadius() { return radius; } //return the area of the circle public double getArea() { return Math.PI * Math.pow(radius, 2); } //return the perimeter of the circle public double getPerimeter() { return 2 * Math.PI * radius; } //return true if the specified point (x, y) is inside this circle public boolean contains(double x, double y) { return Math.sqrt(Math.pow(x - this.x, 2) + Math.pow(y - this.y, 2)) < radius; } //return true if the specified circle is inside this circle public boolean contains(Circle2D circle) { return Math.sqrt(Math.pow(circle.getX() - x, 2) + Math.pow(circle.getY() - y, 2)) <= Math.abs(radius - circle.getRadius()); } //return true if the specified circle overlaps with this circle public boolean overlaps(Circle2D circle) { return Math.sqrt(Math.pow(circle.getX() - x, 2) + Math.pow(circle.getY() - y, 2)) <= radius + circle.getRadius(); } //test program public static void main(String[] args) { //creating object of Circle2D Circle2D c1 = new Circle2D(2, 2, 5.5); //print result System.out.printf("The area of circle is %.5f\n" , c1.getArea()); System.out.printf("The perimeter of a citcle is %.5f\n" , c1.getPerimeter()); System.out.println("Does circle contain the point(3,3)? " + c1.contains(3, 3)); System.out.println("Does circle contain the circle centered at point(13, 20) and radius 10.5? " + c1.contains(new Circle2D(13, 20, 10.5))); System.out.println("Does circle1 overlap the circle centered at point(3, 5) and radius 2.3? " + c1.overlaps(new Circle2D(3, 5, 2.3))); } } I ran this program and I'm getting a different result as that is shown in the solution which was given. This is the output which I got The area of circle is 94.98500 The perimeter of a citcle is 34.54000 Does circle contain the point(3,3)? true Does circle contain the circle centered at point(13, 20) and radius 10.5? false Does circle1 overlap the circle centered at point(3, 5) and radius 2.3? false Can you please check if this is the correct resu
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
package assignment;
public class Circle2D2 {
//data fields specifying the center of the circle
private double x, y;
//data field radius
private double radius;
//default circle with (0, 0) for (x, y) and 1 for radius
Circle2D2() {
this(0, 0, 1);
}
//circle with the specified x, y, and radius
Circle2D2(double x, double y, double radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
//return x
public double getX() {
return x;
}
//return y
public double getY() {
return y;
}
//return radius
public double getRadius() {
return radius;
}
//return the area of the circle
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
//return the perimeter of the circle
public double getPerimeter() {
return 2 * Math.PI * radius;
}
//return true if the specified point (x, y) is inside this circle
public boolean contains(double x, double y) {
return Math.sqrt(Math.pow(x - this.x, 2) +
Math.pow(y - this.y, 2))
< radius;
}
//return true if the specified circle is inside this circle
public boolean contains(Circle2D circle) {
return Math.sqrt(Math.pow(circle.getX() - x, 2) +
Math.pow(circle.getY() - y, 2))
<= Math.abs(radius - circle.getRadius());
}
//return true if the specified circle overlaps with this circle
public boolean overlaps(Circle2D circle) {
return Math.sqrt(Math.pow(circle.getX() - x, 2) +
Math.pow(circle.getY() - y, 2))
<= radius + circle.getRadius();
}
//test program
public static void main(String[] args) {
//creating object of Circle2D
Circle2D c1 = new Circle2D(2, 2, 5.5);
//print result
System.out.printf("The area of circle is %.5f\n" , c1.getArea());
System.out.printf("The perimeter of a citcle is %.5f\n" , c1.getPerimeter());
System.out.println("Does circle contain the point(3,3)? " + c1.contains(3, 3));
System.out.println("Does circle contain the circle centered at point(13, 20) and radius 10.5? "
+ c1.contains(new Circle2D(13, 20, 10.5)));
System.out.println("Does circle1 overlap the circle centered at point(3, 5) and radius 2.3? "
+ c1.overlaps(new Circle2D(3, 5, 2.3)));
}
}
I ran this program and I'm getting a different result as that is shown in the solution which was given. This is the output which I got
The area of circle is 94.98500
The perimeter of a citcle is 34.54000
Does circle contain the point(3,3)? true
Does circle contain the circle centered at point(13, 20) and radius 10.5? false
Does circle1 overlap the circle centered at point(3, 5) and radius 2.3? false
Can you please check if this is the correct result

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

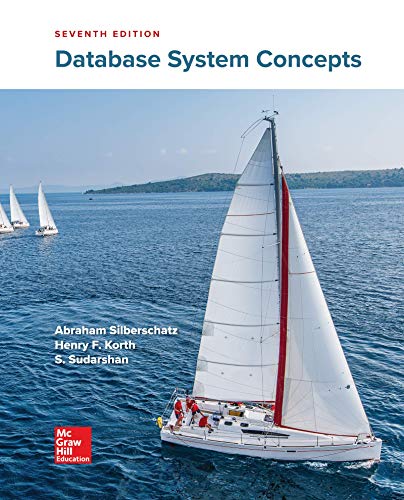
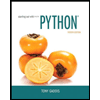
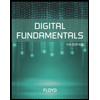
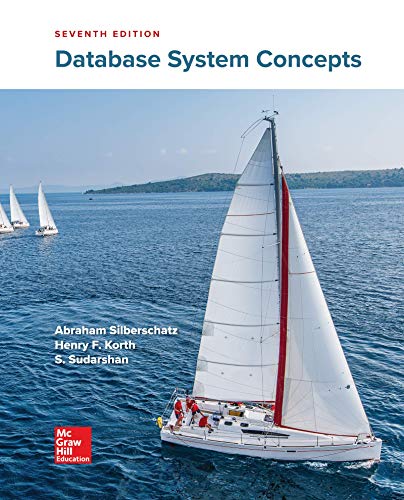
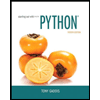
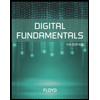
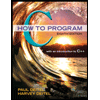
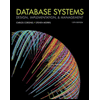
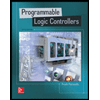