Java Programming Please do not change anything in Student class or Course class class John_Smith extends Student{ public John_Smith() { setFirstName("John"); setLastName("Smith"); setEmail("jsmith@jaguar.tamu.edu"); setGender("Male"); setPhoneNumber("(200)00-0000"); setJNumber("J0101459"); } class MyCourse extends Course { public MyCourse { class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String courseName) { this.courseName = courseName; } public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; } } class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList courseList; public String getFullName() { return firstName + " " + lastName; } public void setFirstName(String fName) { firstName = fName; } public void setLastName(String lName) { lastName = lName; } public String getGender() { return gender; } public void setGender(String gen) { gender = gen; } public String getPhoneNumber() { return phoneNumber; } public void setPhoneNumber(String pNumber) { phoneNumber = pNumber; } public String getEmail() { return email; } public void setEmail(String e_mail) { email = e_mail; } public String getJNumber() { return jNumber; } public void setJNumber(String jNum) { jNumber = jNum; } /* OVERRIDE! Print student's basic information following the format in the assignment's description*/ public void printBasicInfo() { } /* OVERRIDE! Print all courses following the format in the assignment's description */ public void printCourseList() { } /* OVERRIDE! Enroll a new course by adding it into the courseList Do NOT add a course if it is already in the courseList */ public void addCourse(MyCourse course) { } /* OVERRIDE! If the course exists, remove it from the courseList throw CourseNotFoundException if it doesn't exist*/ public void dropCourse(MyCourse course) { } /* OVERRIDE! Return true if the course is currently enrolled, otherwise, false */ public boolean isEnrolled(MyCourse course) { return false; } /* OVERRIDE! Return total credit hours for all enrolled courses */ public int getTotalCredits() { return 0; } }
Java Programming
Please do not change anything in Student class or Course class
class John_Smith extends Student{
public John_Smith() {
setFirstName("John");
setLastName("Smith");
setEmail("jsmith@jaguar.tamu.edu");
setGender("Male");
setPhoneNumber("(200)00-0000");
setJNumber("J0101459");
}
class MyCourse extends Course {
public MyCourse {
class Course {
private String courseNumber;
private String courseName;
private int creditHrs;
public Course (String number, String name, int creditHrs){
this.courseNumber = number;
this.courseName = name;
this.creditHrs = creditHrs;
}
public String getNumber() {
return courseNumber;
}
public String getName() {
return courseName;
}
public int getCreditHrs() {
return creditHrs;
}
public void setCourseNumber(String courseNumber) {
this.courseNumber = courseNumber;
}
public void setCourseName(String courseName) {
this.courseName = courseName;
}
public void setCreditHrs(int creditHrs) {
this.creditHrs = creditHrs;
}
}
class Student {
private String firstName;
private String lastName;
private String gender;
private String phoneNumber;
private String email;
private String jNumber;
protected ArrayList<MyCourse> courseList;
public String getFullName() {
return firstName + " " + lastName;
}
public void setFirstName(String fName) {
firstName = fName;
}
public void setLastName(String lName) {
lastName = lName;
}
public String getGender() {
return gender;
}
public void setGender(String gen) {
gender = gen;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String pNumber) {
phoneNumber = pNumber;
}
public String getEmail() {
return email;
}
public void setEmail(String e_mail) {
email = e_mail;
}
public String getJNumber() {
return jNumber;
}
public void setJNumber(String jNum) {
jNumber = jNum;
}
/* OVERRIDE!
Print student's basic information following the format in the assignment's description*/
public void printBasicInfo() {
}
/* OVERRIDE!
Print all courses following the format in the assignment's description */
public void printCourseList() {
}
/* OVERRIDE!
Enroll a new course by adding it into the courseList
Do NOT add a course if it is already in the courseList */
public void addCourse(MyCourse course) {
}
/* OVERRIDE!
If the course exists, remove it from the courseList
throw CourseNotFoundException if it doesn't exist*/
public void dropCourse(MyCourse course) {
}
/* OVERRIDE!
Return true if the course is currently enrolled, otherwise, false */
public boolean isEnrolled(MyCourse course) {
return false;
}
/* OVERRIDE!
Return total credit hours for all enrolled courses */
public int getTotalCredits() {
return 0;
}
}
![[1] Description: You're asked to create a basic Student's Enrollment System with the provided
Student and Course classes. Please download the source code files from Blackboard. Create two
new classes: (i) FirstName_LastName (replace by your name) that extends the Student class and
(i) MyCourse that extends the Course class.
12] Requirements:
a. You're NOT allowed to change or add ANYTHING in the provided Student and Course
classes.
b. Provide a no-arg constructor for your FirstName_LastName class. The no-arg
constructor should initialize all the data fields in Student class based on your
information and your current semester's enrolled courses.
c. Override 6 methods in Student class, as marked in the source code comments. Please
read the comment sections carefully for descriptions.
d. Override the equals() method in MyCourse class. Two courses are equal if they have the
same course number, course name, and credit hours. You should use this method for all
courses comparisons.
e. Create a customized exception class CourseNotFoundException, throw this exception in
the dropCourse() method if the dropping course doesn't exist in the courseList. The
thrown exception object should contain a meaningful message, including course number,
course name, and credit hours for the not found course. (hint: I demo the
InvalidSideException in Feb 24th lecture, please review for reference)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0e20848f-885a-4953-aa62-49de56df484f%2F96c656fc-debd-492c-8715-653626700df9%2Fj1nzogf_processed.jpeg&w=3840&q=75)
![|3] Sample outputs for printBasicInfo() and printCourseList() methods:
John_Smith js = new John_Smith();
js.printBasicInfo();
Full Name: John Smith
Gender: Male
Phone Number: (210)000-0000
Email: jsmith@jaguar.tamu.edu
JNumber: JO00000
js.printCourseList();
John Smith's Course List
CSCI 1437 Programming Fundamentals II
4 hrs
CISA 2306 Computer Networks
3 hrs
CISA 2356 Systems Analysis and Design
3 hrs
Total
10 hrs](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0e20848f-885a-4953-aa62-49de56df484f%2F96c656fc-debd-492c-8715-653626700df9%2F7lrpvn8_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

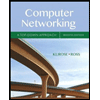
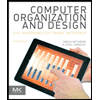
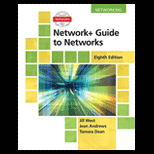
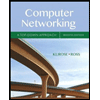
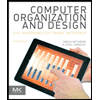
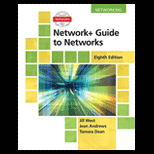
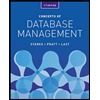
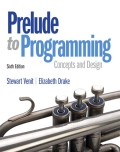
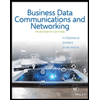