1. In the CheckingAccount class: a. Create one method for depositing. The deposit method should have one parameter to indicate the amount to be deposited. You must make sure the amount to be deposited is positive. b. Create one method for withdrawing. The withdraw method should have one parameter to indicate the amount to be withdrawn and use Boolean return type to show the success of the operation. If the amount to be withdrawn is negative or one tries to over-withdraw (i.e the withdrawing amount is more than the balance), make no change on the balance and return false. If the withdrawn amount is allowed, update the balance by subtracting this withdrawing amount from it and then return true. c. Create a displayAccount() method.i. Print a nice head that includes the customer’s name (similar to the Employee class example)ii. Display the account number, owner’s name, and current balance, each at a separate line 2. Modify the main method in the CheckingAccountDemo class a. Take out all codes from Lab 7. b. Ask for the name, account number, and initial balance for one account from the keyboard input. Create a CheckingAccount object and initialize it using the constructor and the keyboard input values on name, account number, and initial balance. Call the displayAccount() method to print the summary of this account. c. Ask user to input the deposit amount from keyboard, then deposit it into the account you created by calling the deposit method of the CheckingAccount object you have created. Call the displayAccount() method to print the summary of this account. d. Ask user to input the withdraw amount from keyboard, then try to withdraw that amount from the account object. If the operation is successful, call the displayAccount() method to print the summary of this account. Otherwise print the error information that the withdrawing operation failed.
This code is used for the question being asked at the bottom.
public class AccountBalance {
private long accountNumber;
private String ownersName;
private double balance;
public AccountBalance(long accountNumber, String ownersName, double balance) {
this.accountNumber = accountNumber;
this.ownersName = ownersName;
setBalance(balance);
}
public long getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(long accountNumber) {
this.accountNumber = accountNumber;
}
public String getOwnersName() {
return ownersName;
}
public void setOwnersName() {
this.ownersName = ownersName;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
if (balance < 0) {
System.out.println("Balance Cannot be Negative.");
balance = 0;
}
this.balance = balance;
}
}
import java.util.Scanner;
public class AccountBalanceDemo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the name of the account holder: ");
String name = sc.nextLine();
System.out.print("Enter the Account Number: ");
long an = sc.nextLong();
System.out.println("Enter the account balance: ");
double balance = sc.nextDouble();
AccountBalance ab = new AccountBalance(an, name, balance);
System.out.println("Name: " + ab.getOwnersName());
System.out.println("Account Number: " + ab.getAccountNumber());
System.out.println("Balance: " + ab.getBalance());
}
}
Continue your work on Lab7.
1. In the CheckingAccount class:
a. Create one method for depositing. The deposit method should have one parameter to indicate the amount to be deposited. You must make sure the amount to be deposited is positive.
b. Create one method for withdrawing. The withdraw method should have one parameter to indicate the amount to be withdrawn and use Boolean return type to show the success of the operation. If the amount to be withdrawn is negative or one tries to over-withdraw (i.e the withdrawing amount is more than the balance), make no change on the balance and return false. If the withdrawn amount is allowed, update the balance by subtracting this withdrawing amount from it and then return true.
c. Create a displayAccount() method.i. Print a nice head that includes the customer’s name (similar to the Employee class example)ii. Display the account number, owner’s name, and current balance, each at a separate line
2. Modify the main method in the CheckingAccountDemo class
a. Take out all codes from Lab 7.
b. Ask for the name, account number, and initial balance for one account from the keyboard input. Create a CheckingAccount object and initialize it using the constructor and the keyboard input values on name, account number, and initial balance. Call the displayAccount() method to print the summary of this account.
c. Ask user to input the deposit amount from keyboard, then deposit it into the account you created by calling the deposit method of the CheckingAccount object you have created. Call the displayAccount() method to print the summary of this account.
d. Ask user to input the withdraw amount from keyboard, then try to withdraw that amount from the account object. If the operation is successful, call the displayAccount() method to print the summary of this account. Otherwise print the error information that the withdrawing operation failed.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

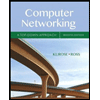
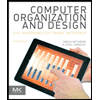
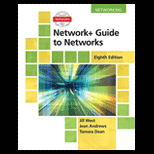
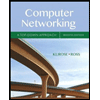
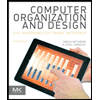
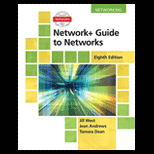
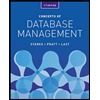
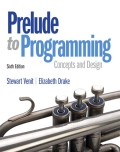
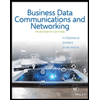