This code will be used to answer the question at the bottom. public class AccountBalance { private long accountNumber; private String ownersName; private double balance; public AccountBalance(long accountNumber, String ownersName, double balance) { this.accountNumber = accountNumber; this.ownersName = ownersName; setBalance(balance); } public long getAccountNumber() { return accountNumber; } public void setAccountNumber(long accountNumber) { this.accountNumber = accountNumber; } public String getOwnersName() { return ownersName; } public void setOwnersName() { this.ownersName = ownersName; } public double getBalance() { return balance; } public void setBalance(double balance) { if (balance < 0) { System.out.println("Balance Cannot be Negative."); balance = 0; } this.balance = balance; } } class CheckingAccount extends AccountBalance{ CheckingAccount(long a, String b, double c){ super(a,b,c); displayAccount(); } public boolean deposit(double a) { if(a<=0) System.out.println("Invalid amount"); else super.setBalance(super.getBalance()+a); return false; } public boolean withdraw(double a) { if (a<=0 || a>super.getBalance()) { return false; } else { super.setBalance(super.getBalance()-a); return true; } } public void displayAccount() { System.out.println("Account Number: " + super.getAccountNumber()); System.out.println("Owner Name: " + super.getOwnersName()); System.out.println("Balance: " + super.getBalance()); } } import java.util.Scanner; public class AccountBalanceDemo { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the name of the account holder: "); String name = sc.nextLine(); System.out.print("Enter the Account Number: "); long an = sc.nextLong(); System.out.println("Enter the account balance: "); double balance = sc.nextDouble(); CheckingAccount ab = new CheckingAccount(an,name, balance); ab.deposit(100); ab.displayAccount(); if(ab.deposit(1000)) ab.displayAccount(); else System.out.println("Invalid."); if(ab.withdraw(100)); ab.displayAccount(); } } 1. Use your Account class codes as in your Lab 8. 2. Modify the main method in your CheckingAccountDemo class: Take out all previous code. Declare an object array with 10 accounts. Create a for loop to ask user to input each account’s information (name, account number, and initial balance) from keyboard and then initialize for each account object. Create a while loop to allow one to work on depositing and withdrawing operations on any account till one want to exit. To work on an account, the user needs to input the owner name of the account. Then you write code to search the account object array to find the object that has the right owner name (you will get bonus points if you write the account searching part as a static method and simply call this method). If an account is found, ask user to choose withdrawing or depositing operation and also indicate the amount. Then perform the corresponding operation on the selected account. Outside of the while loop for the account operations, write a for loop to print the summary for each account.
This code will be used to answer the question at the bottom.
public class AccountBalance {
private long accountNumber;
private String ownersName;
private double balance;
public AccountBalance(long accountNumber, String ownersName, double balance) {
this.accountNumber = accountNumber;
this.ownersName = ownersName;
setBalance(balance);
}
public long getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(long accountNumber) {
this.accountNumber = accountNumber;
}
public String getOwnersName() {
return ownersName;
}
public void setOwnersName() {
this.ownersName = ownersName;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
if (balance < 0) {
System.out.println("Balance Cannot be Negative.");
balance = 0;
}
this.balance = balance;
}
}
class CheckingAccount extends AccountBalance{
CheckingAccount(long a, String b, double c){
super(a,b,c);
displayAccount();
}
public boolean deposit(double a) {
if(a<=0)
System.out.println("Invalid amount");
else
super.setBalance(super.getBalance()+a);
return false;
}
public boolean withdraw(double a) {
if (a<=0 || a>super.getBalance()) {
return false;
}
else {
super.setBalance(super.getBalance()-a);
return true;
}
}
public void displayAccount() {
System.out.println("Account Number: " + super.getAccountNumber());
System.out.println("Owner Name: " + super.getOwnersName());
System.out.println("Balance: " + super.getBalance());
}
}
import java.util.Scanner;
public class AccountBalanceDemo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the name of the account holder: ");
String name = sc.nextLine();
System.out.print("Enter the Account Number: ");
long an = sc.nextLong();
System.out.println("Enter the account balance: ");
double balance = sc.nextDouble();
CheckingAccount ab = new CheckingAccount(an,name, balance);
ab.deposit(100);
ab.displayAccount();
if(ab.deposit(1000))
ab.displayAccount();
else
System.out.println("Invalid.");
if(ab.withdraw(100));
ab.displayAccount();
}
}
1. Use your Account class codes as in your Lab 8.
2. Modify the main method in your CheckingAccountDemo class:
- Take out all previous code.
- Declare an object array with 10 accounts.
- Create a for loop to ask user to input each account’s information (name, account number, and initial balance) from keyboard and then initialize for each account object.
- Create a while loop to allow one to work on depositing and withdrawing operations on any account till one want to exit.
- To work on an account, the user needs to input the owner name of the account. Then you write code to search the account object array to find the object that has the right owner name (you will get bonus points if you write the account searching part as a static method and simply call this method).
- If an account is found, ask user to choose withdrawing or depositing operation and also indicate the amount. Then perform the corresponding operation on the selected account.
- Outside of the while loop for the account operations, write a for loop to print the summary for each account.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

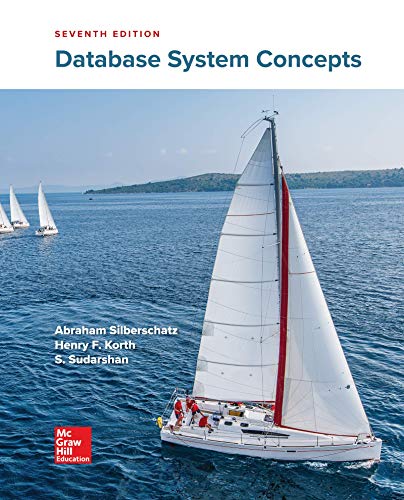
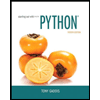
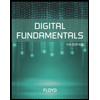
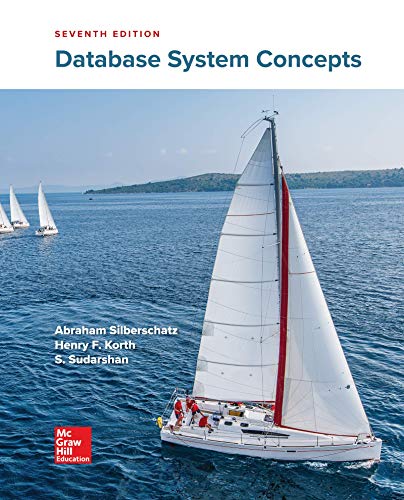
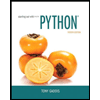
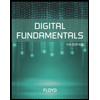
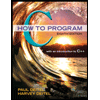
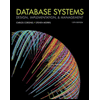
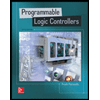