public class Stadium extends baseStadium{ public int typeTicket; int floors; int seating; String type; public float ticketPrice; public String leagueName=""; public void SetTecketName(String val) { leagueName=val; } public void setType() { if (typeTicket == 1) { type = "VIP"; } else { type = "Regular"; } } public String getType() { return type; } public void setPrice() { if (typeTicket == 1) { ticketPrice = 250; } else { ticketPrice = 100; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
public class Stadium extends baseStadium{ public int typeTicket; int floors; int seating; String type; public float ticketPrice; public String leagueName=""; public void SetTecketName(String val) { leagueName=val; } public void setType() { if (typeTicket == 1) { type = "VIP"; } else { type = "Regular"; } } public String getType() { return type; } public void setPrice() { if (typeTicket == 1) { ticketPrice = 250; } else { ticketPrice = 100; } } public void setFloors(int floors) { this.floors = floors; } public int getFloors() { return floors; } public void setSeating(int seating) { this.seating = seating; } public int getSeating() { return seating; } public void printDetails() { System.out.println("Ticket Details:"); System.out.println("Ticket Type: " + getType()); System.out.println("Floors: " + getFloors()); System.out.println("Seating: " + getSeating()); } public void printMatch() { System.out.println("Match: Manchester United vs Liverpool"); } public void printLeagueNane() { System.out.println("League Name:"+leagueName); } public void printData() { System.out.println("Date: 10th February 2023"); } public void printTime() { System.out.println("Time: 8:00 PM"); } public void printStadium() { System.out.println("Stadium: Old Trafford"); } public void printLocation() { System.out.println("Location: Manchester, England"); } } public class ticket implements TicketService { public int ticketNumber = 1234; public void PlusTictNo() { ticketNumber+=1; } //hrer Overloading on GenerateRandomNo method public int GenerateRandomNo() { Random rand = new Random(); int upperbound = 2515; int int_random = rand.nextInt(upperbound); return int_random; } public int GenerateRandomNo(int upper) { Random rand = new Random(); int int_random = rand.nextInt(upper); return int_random; } @Override public void PrintData(String val) { System.out.println(val); } } public interface TicketService { void PrintData(String val); } public abstract class baseStadium { abstract void printDetails() ; }//I want to draw an environmental scheme for this code, the example of the image, every separate class


Step by step
Solved in 2 steps

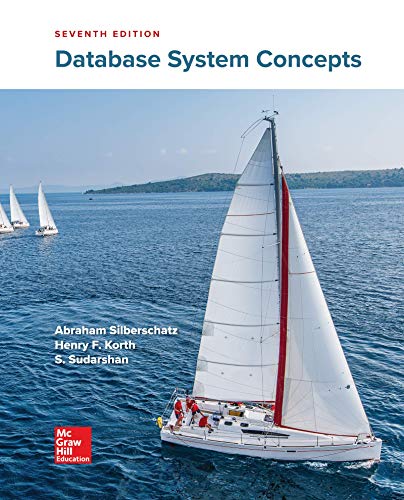
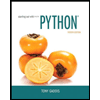
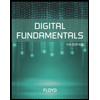
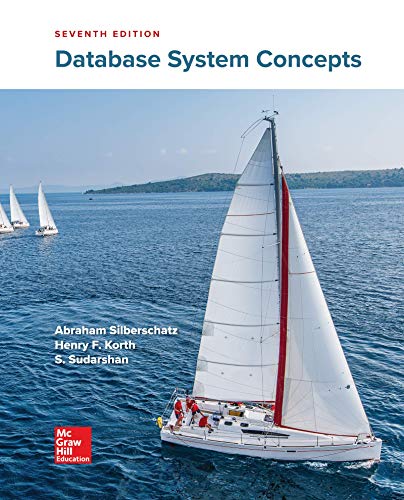
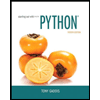
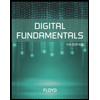
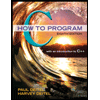
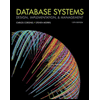
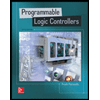