Design an Essay class that is derived from the GradedActivity class: class GradedActivity{ private: double score; public: GradedActivity() {score = 0.0;} GradedActivity(double s) {score = s;} void setScore(double s) {score = s;} double getScore() const {return score;} char getLetterGrade() const; }; char GradedActivity::getLetterGrade() const{ char letterGrade; if (score > 89) { letterGrade = 'A'; } else if (score > 79) { letterGrade = 'B'; } else if (score > 69) { letterGrade = 'C'; } else if (score > 59) { letterGrade = 'D'; } else { letterGrade = 'F'; } return letterGrade; } The Essay class should determine the grade a student receives on an essay. The student's essay score can be up to 100, and is made up of four parts: Grammar: up to 30 points Spelling: up to 20 points Correct length: up to 20 points Content: up to 30 points The Essay class should have a double member variable for each of these sections, as well as a mutator that sets the values of these variables. It should add all of these values to get the student's total score on an Essay. Demonstrate your class in a program that prompts the user to input points received for grammar, spelling, length, and content, and then prints the numeric and letter grade received by the student. I just need the numeric grade my code: #include using namespace std; class GradedActivity { private: double score; public: GradedActivity() { score = 0.0; } GradedActivity(double s) { score = s; } void setScore(double s) { score = s; } double getScore() const { return score; } char getLetterGrade() const; }; char GradedActivity::getLetterGrade() const { char letterGrade; if (score > 89) { letterGrade = 'A'; } else if (score > 79) { letterGrade = 'B'; } else if (score > 69) { letterGrade = 'C'; } else if (score > 59) { letterGrade = 'D'; } else { letterGrade = 'F'; } return letterGrade; } class Essay :public GradedActivity { private: //scores for various sections double grammar_Score,spelling_Score,length_Score,content_Score; public: double getgrammar_Score()const { return grammar_Score; } void setgrammar_Score(double s) { grammar_Score = s; } double getspelling_Score()const { return spelling_Score; } void setspelling_Score(double s) { spelling_Score = s; } double getlength_Score()const { return length_Score; } void setlength_Score(double s) { length_Score = s; } double getcontent_Score()const { return content_Score; } void setcontent_Score(double s) { content_Score = s; } char getGrade() { double score = grammar_Score + spelling_Score + length_Score + content_Score; setScore(score); char grade = getLetterGrade(); return grade; } }; int main() { Essay essay; double grammar_Score,spelling_Score,length_Score,content_Score; cout<<"Enter points received for grammar:"; cin>>grammar_Score; essay.setgrammar_Score(grammar_Score); cout<<"Enter points received for spelling:"; cin>>spelling_Score; essay.setspelling_Score(spelling_Score); cout<<"Enter points received for correct length:"; cin>>length_Score; essay.setlength_Score(length_Score); cout<<"Enter points received for content:"; cin>>content_Score; essay.setcontent_Score(content_Score); cout<<"Numeric Grade: " <
Design an Essay class that is derived from the GradedActivity class:
class GradedActivity{
private:
double score;
public:
GradedActivity()
{score = 0.0;}
GradedActivity(double s)
{score = s;}
void setScore(double s)
{score = s;}
double getScore() const
{return score;}
char getLetterGrade() const;
};
char GradedActivity::getLetterGrade() const{
char letterGrade;
if (score > 89) {
letterGrade = 'A';
} else if (score > 79) {
letterGrade = 'B';
} else if (score > 69) {
letterGrade = 'C';
} else if (score > 59) {
letterGrade = 'D';
} else {
letterGrade = 'F';
}
return letterGrade;
}
The Essay class should determine the grade a student receives on an essay. The student's essay score can be up to 100, and is made up of four parts:
- Grammar: up to 30 points
- Spelling: up to 20 points
- Correct length: up to 20 points
- Content: up to 30 points
The Essay class should have a double member variable for each of these sections, as well as a mutator that sets the values of these variables. It should add all of these values to get the student's total score on an Essay.
Demonstrate your class in a program that prompts the user to input points received for grammar, spelling, length, and content, and then prints the numeric and letter grade received by the student.
I just need the numeric grade
my code:
#include <iostream>
using namespace std;
class GradedActivity
{
private:
double score;
public:
GradedActivity()
{
score = 0.0;
}
GradedActivity(double s)
{
score = s;
}
void setScore(double s)
{
score = s;
}
double getScore() const
{
return score;
}
char getLetterGrade() const;
};
char GradedActivity::getLetterGrade() const
{
char letterGrade;
if (score > 89)
{
letterGrade = 'A';
}
else if (score > 79)
{
letterGrade = 'B';
}
else if (score > 69)
{
letterGrade = 'C';
}
else if (score > 59)
{
letterGrade = 'D';
}
else
{
letterGrade = 'F';
}
return letterGrade;
}
class Essay :public GradedActivity
{
private:
//scores for various sections
double grammar_Score,spelling_Score,length_Score,content_Score;
public:
double getgrammar_Score()const
{
return grammar_Score;
}
void setgrammar_Score(double s)
{
grammar_Score = s;
}
double getspelling_Score()const
{
return spelling_Score;
}
void setspelling_Score(double s)
{
spelling_Score = s;
}
double getlength_Score()const
{
return length_Score;
}
void setlength_Score(double s)
{
length_Score = s;
}
double getcontent_Score()const
{
return content_Score;
}
void setcontent_Score(double s)
{
content_Score = s;
}
char getGrade()
{
double score = grammar_Score + spelling_Score + length_Score + content_Score;
setScore(score);
char grade = getLetterGrade();
return grade;
}
};
int main()
{
Essay essay;
double grammar_Score,spelling_Score,length_Score,content_Score;
cout<<"Enter points received for grammar:";
cin>>grammar_Score;
essay.setgrammar_Score(grammar_Score);
cout<<"Enter points received for spelling:";
cin>>spelling_Score;
essay.setspelling_Score(spelling_Score);
cout<<"Enter points received for correct length:";
cin>>length_Score;
essay.setlength_Score(length_Score);
cout<<"Enter points received for content:";
cin>>content_Score;
essay.setcontent_Score(content_Score);
cout<<"Numeric Grade: " <<essay.getScore();
cout<<"Letter Grade: "<<essay.getGrade();
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

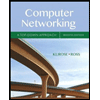
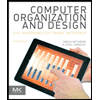
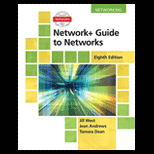
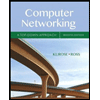
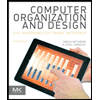
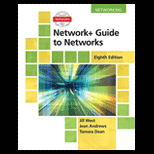
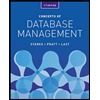
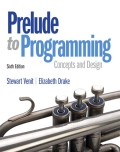
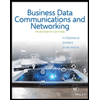