Problem Statement Design a Palm class that has the following member: • A virtual printName) function that displays the class information which is the Class's name. Design a KingPalm class that is derived from the Palm class. The KingPalm class should have the following: • A printName function that overrides from the base class. The King Palm class' print function should display only the class's name. Design a QueenPalm class that is derived from the Palm class. The QueenPalm class should have the following: • A printName function that overrides from the base class. The QueenPalm class' print function should display only the class's name. Write a program that has an array of Palm pointers. The array elements should be initialized to Palm, KingPalm, and QueenPalm objects. The program should then step through the array with a loop and call each object's printName function. Partial Solution #include using namespace std; }; [Select] }; [Select] class [Select] [Select] } [Select] } cout << "Palm\n"; public: } [Select] class QueenPalm [Select] cout << "KingPalm\n"; public: void printName() { cout << "QueenPalm\n"; int main() { V [Select] Palm [Select] } return 0; void [Select] :public [Select] printName() { for(int i = 0; i < 3; i++){ V public Palm { new [Select] { palmPtr[i] [Select] ✓ 0) { palmPtr[3] = { [Select] new QueenPalm [Select] { (0), printName(); }; ✓ Palm(),
Problem Statement Design a Palm class that has the following member: • A virtual printName) function that displays the class information which is the Class's name. Design a KingPalm class that is derived from the Palm class. The KingPalm class should have the following: • A printName function that overrides from the base class. The King Palm class' print function should display only the class's name. Design a QueenPalm class that is derived from the Palm class. The QueenPalm class should have the following: • A printName function that overrides from the base class. The QueenPalm class' print function should display only the class's name. Write a program that has an array of Palm pointers. The array elements should be initialized to Palm, KingPalm, and QueenPalm objects. The program should then step through the array with a loop and call each object's printName function. Partial Solution #include using namespace std; }; [Select] }; [Select] class [Select] [Select] } [Select] } cout << "Palm\n"; public: } [Select] class QueenPalm [Select] cout << "KingPalm\n"; public: void printName() { cout << "QueenPalm\n"; int main() { V [Select] Palm [Select] } return 0; void [Select] :public [Select] printName() { for(int i = 0; i < 3; i++){ V public Palm { new [Select] { palmPtr[i] [Select] ✓ 0) { palmPtr[3] = { [Select] new QueenPalm [Select] { (0), printName(); }; ✓ Palm(),
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
C++
![# Problem Statement
Design a `Palm` class that has the following member:
- A `virtual` `printName` function that displays the class information which is the Class's name.
Design a `KingPalm` class that is derived from the `Palm` class. The `KingPalm` class should have the following:
- A `printName` function that overrides from the base class. The `KingPalm` class's print function should display only the class's name.
Design a `QueenPalm` class that is derived from the `Palm` class. The `QueenPalm` class should have the following:
- A `printName` function that overrides from the base class. The `QueenPalm` class's print function should display only the class’s name.
Write a program that has an array of `Palm` pointers. The array elements should be initialized to `Palm`, `KingPalm`, and `QueenPalm` objects. The program should then step through the array with a loop and call each object’s `printName` function.
# Partial Solution
```cpp
#include <iostream>
using namespace std;
class Palm {
public:
virtual void printName() {
cout << "Palm\n";
}
};
class KingPalm : public Palm {
public:
void printName() {
cout << "KingPalm\n";
}
};
class QueenPalm : public Palm {
public:
void printName() {
cout << "QueenPalm\n";
}
};
int main() {
Palm *palmPtr[3] = { new Palm(), new KingPalm(), new QueenPalm() };
for (int i = 0; i < 3; i++) {
palmPtr[i]->printName();
}
return 0;
}
```
This C++ code demonstrates object-oriented programming concepts such as class inheritance and polymorphism. It includes:
- A base class `Palm` with a virtual method `printName`.
- Derived classes `KingPalm` and `QueenPalm` that override the `printName` method.
- An array of pointers to `Palm` objects initialized to instances of `Palm`, `KingPalm`, and `QueenPalm`.
- A loop that iterates through the array and calls the `printName` method, demonstrating polymorphism.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdd27f7e0-834e-40ed-ac12-9ff71783ae4a%2F84089506-4bc2-405d-bbfd-ef4edf371503%2Fwonhr4e_processed.png&w=3840&q=75)
Transcribed Image Text:# Problem Statement
Design a `Palm` class that has the following member:
- A `virtual` `printName` function that displays the class information which is the Class's name.
Design a `KingPalm` class that is derived from the `Palm` class. The `KingPalm` class should have the following:
- A `printName` function that overrides from the base class. The `KingPalm` class's print function should display only the class's name.
Design a `QueenPalm` class that is derived from the `Palm` class. The `QueenPalm` class should have the following:
- A `printName` function that overrides from the base class. The `QueenPalm` class's print function should display only the class’s name.
Write a program that has an array of `Palm` pointers. The array elements should be initialized to `Palm`, `KingPalm`, and `QueenPalm` objects. The program should then step through the array with a loop and call each object’s `printName` function.
# Partial Solution
```cpp
#include <iostream>
using namespace std;
class Palm {
public:
virtual void printName() {
cout << "Palm\n";
}
};
class KingPalm : public Palm {
public:
void printName() {
cout << "KingPalm\n";
}
};
class QueenPalm : public Palm {
public:
void printName() {
cout << "QueenPalm\n";
}
};
int main() {
Palm *palmPtr[3] = { new Palm(), new KingPalm(), new QueenPalm() };
for (int i = 0; i < 3; i++) {
palmPtr[i]->printName();
}
return 0;
}
```
This C++ code demonstrates object-oriented programming concepts such as class inheritance and polymorphism. It includes:
- A base class `Palm` with a virtual method `printName`.
- Derived classes `KingPalm` and `QueenPalm` that override the `printName` method.
- An array of pointers to `Palm` objects initialized to instances of `Palm`, `KingPalm`, and `QueenPalm`.
- A loop that iterates through the array and calls the `printName` method, demonstrating polymorphism.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
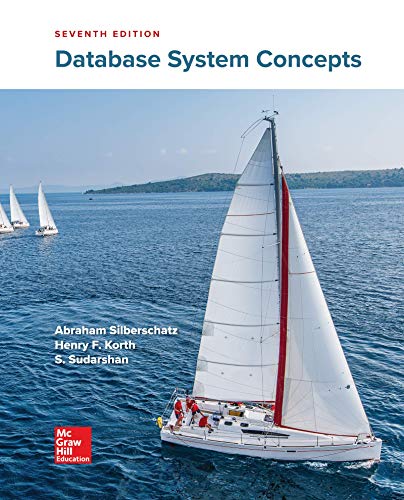
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
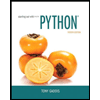
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
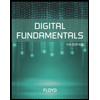
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
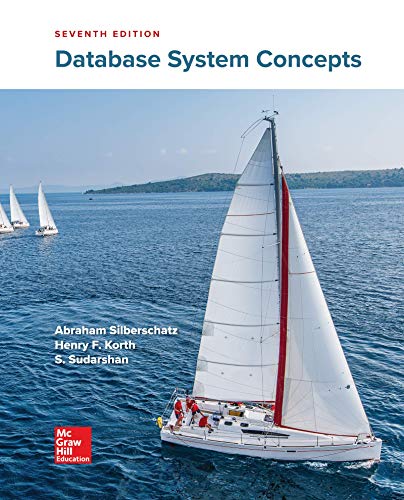
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
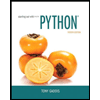
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
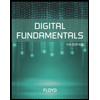
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
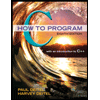
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
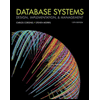
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
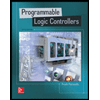
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education