Structute Using c++ Queue The Full Question is on the Picture I need to continue on this code please ::: #include using namespace std; struct node { int data; node *next; node(int d,node *n=0) { data=d; next=n;
Data Structute Using c++ Queue
The Full Question is on the Picture I need to continue on this code please :::
#include <iostream>
using namespace std;
struct node
{
int data;
node *next;
node(int d,node *n=0)
{
data=d;
next=n;
}
};
class queue
{
node *front,*rear;
public:
queue();
bool empty();
void append(int el);
bool serve();
bool retrieve(int &el);
//destructor ...
};
bool queue::empty()
{
return front==0;
}
queue::queue()
{
front=rear=0;
}
void queue::append(int el)
{
if(front==0)
front=rear=new node(el);
else
rear=rear->next=new node(el);
}
bool queue::serve()
{
if(front==0)
return false;
if(front==rear)
{
delete front;
front=rear=0;
}
else
{
node *t=front;
front=front->next;
delete t;
}
return true;
}
bool queue::retrieve(int &el)
{
if(front==0)
return false;
el=front->data;
return true;
}
int main ()
{
QueueLinked<int> custQ; // Line (queue) of customers containing the
QueueArray<int> custQ; // Line (queue) of customers containing the
// time that each customer arrived and
// joined the line
int simLength, // Length of simulation (minutes)
minute, // Current minute
timeArrived, // Time dequeued customer arrived
waitTime, // How long dequeued customer waited
totalServed = 0, // Total customers served
totalWait = 0, // Total waiting time
maxWait = 0, // Longest wait
numArrivals = 0; // Number of new arrivals
// Seed the random number generator. Equally instructive to run the
// simulation with the generator seeded and not seeded.
srand(7);
cout << endl << "Enter the length of time to run the simulator : ";
cin >> simLength;
// Put your code here to run this simulation. Use "rand()" to get
// a pseudorandom number that you can use to calculate probabilities.
///starting a minute zero the
minute = 0;
int k;
while(minute != simLength)
{
///check to see if queue is empty
if(!custQ.isEmpty())
{
///if not empty, dequeue customer and record data
timeArrived = custQ.dequeue();
waitTime = minute - timeArrived;
totalWait += waitTime;
totalServed++;
if(waitTime > maxWait)
{
maxWait = waitTime;
}
}
///generate a random number using mod 4 to get appropriate number
k = rand() % 4;
///if the number is one add one person to the queue and increment numArrivals
if(k == 1)
{
custQ.enqueue(minute);
numArrivals++;
}
///if the number is two add two people to the queue and add two to numArrivals
if(k == 2)
{
custQ.enqueue(minute);
custQ.enqueue(minute);
numArrivals += 2;
}
///update the while loop by incrementing the minute variable
minute++;
}
// Print out simulation results
cout << endl;
cout << "Customers served : " << totalServed << endl;
cout << "Average wait : " << setprecision(2)
<< double(totalWait)/totalServed << endl;
cout << "Longest wait : " << maxWait << endl;
system("pause");
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

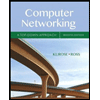
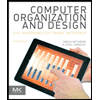
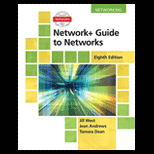
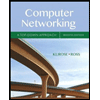
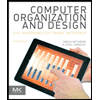
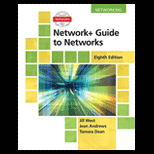
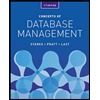
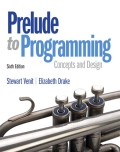
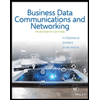