Two integers, babies 1 and babies2, are read from input as the number of babies of two turtles. headObj has the default value of -1. Create a new node first Turtle with integer babies1 and insert firstTurtle after headObj. Then, create a second node second Turtle with integer babies2 and insert second Turtle after first Turtle. Ex: If the input is 12 19, then the output is: -1 12 19
#include <iostream>
using namespace std;
class TurtleNode {
public:
TurtleNode(int babiesInit = 0, TurtleNode* nextLoc = nullptr);
void InsertAfter(TurtleNode* nodeLoc);
TurtleNode* GetNext();
void PrintNodeData();
private:
int babiesVal;
TurtleNode* nextNodePtr;
};
TurtleNode::TurtleNode(int babiesInit, TurtleNode* nextLoc) {
this->babiesVal = babiesInit;
this->nextNodePtr = nextLoc;
}
void TurtleNode::InsertAfter(TurtleNode* nodeLoc) {
TurtleNode* tmpNext = nullptr;
tmpNext = this->nextNodePtr;
this->nextNodePtr = nodeLoc;
nodeLoc->nextNodePtr = tmpNext;
}
TurtleNode* TurtleNode::GetNext() {
return this->nextNodePtr;
}
void TurtleNode::PrintNodeData() {
cout << this->babiesVal << endl;
}
int main() {
TurtleNode* headObj = nullptr;
TurtleNode* firstTurtle = nullptr;
TurtleNode* secondTurtle = nullptr;
TurtleNode* currTurtle = nullptr;
int babies1;
int babies2;
cin >> babies1;
cin >> babies2;
headObj = new TurtleNode(-1);
***********MISSING CODE NEEDS TO GO HERE WITH NO OTHER CHANGES TO EXISTING CODE*************
currTurtle = headObj;
while (currTurtle != nullptr) {
currTurtle->PrintNodeData();
currTurtle = currTurtle->GetNext();
}
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

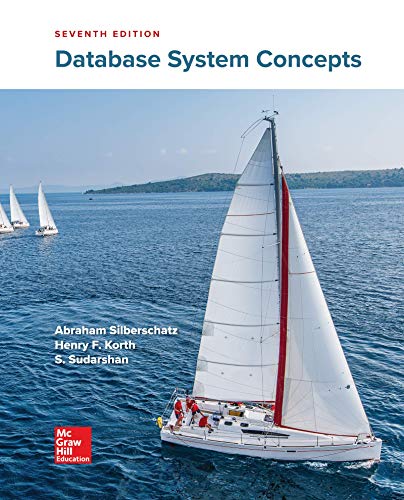
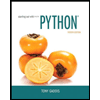
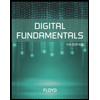
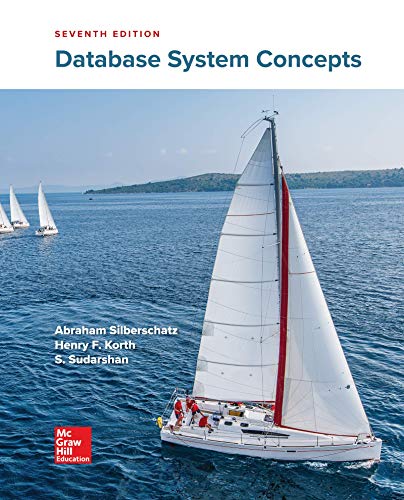
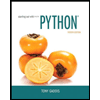
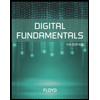
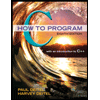
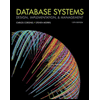
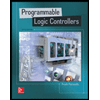