Vigenere.h #include using namespace std; //Create class Vigenere class Vigenere { //Members private: string key; //Functions public: //Constructor Vigenere(); //Setter and getter void setKey(string key); string getKey(); //Convert into upper case string toUpperCase(string k); //Encrypt string encrypt(string word); //Decrypt string decrypt(string word); }; Vigenere.cpp //Implementation #include "Vigenere.h" //Constructor Vigenere::Vigenere() { this->key = ""; } //Setter and getter void Vigenere::setKey(string key) { this->key = key; } string Vigenere::getKey() { return key; } //Convert into upper case string Vigenere::toUpperCase(string k) { for (int i = 0; i < k.length(); i++) { if (isalpha(k[i])) { k[i] = toupper(k[i]); } } return k; } //Encrypt string Vigenere::encrypt(string word) { return key; string output = ""; for (int i = 0, j = 0; i < word.length(); i++) { char c = word[i]; if (c >= 'a' && c <= 'z') { c += 'A' - 'a'; } else if (c < 'A' || c>'Z') { continue; } output += (c + key[j] - 2 * 'A') % 26 + 'A'; j = (j + 1) % key.length(); } return output; } //Decrypt string Vigenere::decrypt(string word) { string output = ""; for (int i = 0, j = 0; i < word.length(); i++) { char c = word[i]; if (c >= 'a' && c <= 'z') { c += 'A' - 'a'; } else if (c < 'A' || c>'Z') { continue; } output += (c - key[j] + 26) % 26 + 'A'; j = (j + 1) % key.length(); } return output; } Message.h #include #include "Vigenere.h" //Create class class Message { //Members private: vectorwords; Vigenere v; //Functions public: //Constructor Message(string key); //Encrypt void encryptWord(char* token); //Decrypt void decryptWord(char* token); //Make a file void makeFile(string fileName); //Display void showWords(); }; Message.cpp //Implementation of Message class #include "Message.h" #include #include //Constructor Message::Message(string key) { v.setKey(key); } //Encrypt void Message::encryptWord(char* token) { words.push_back(v.encrypt(token)); } //Decrypt void Message::decryptWord(char* token) { words.push_back(v.decrypt(token)); } //Make a file void Message::makeFile(string fileName) { ofstream out(fileName); for (int i = 0; i < words.size(); i++) { out << words[i] << " "; } out << endl; out.close(); } //Display void Message::showWords() { for (int i = 0; i < words.size(); i++) { cout << words[i] << " "; } cout << endl; } VigenerCypherDriver.cpp #include #include #include #include "Message.h" //Function prototype int getMenu(); int main() { string key, filename, line; char buff[1000]; char* token = NULL; //Prompt for key cout << "Enter an encryption/decryption key:\n"; getline(cin, key); cout << endl; //Create message object Message msg(key); //Get choice int opt = getMenu(); //Loop until quit while (opt != 3) { if (opt == 1) { cout << "\nEnter the name of the file to encrypt: "; getline(cin, filename); ifstream in(filename); if (!in) { cout << "File not found!!!\n"; } else { while (!in.eof()) { in.getline(buff, 1000); const char* delim = " "; char* next_token; token = strtok_s(buff, delim, &next_token); while (token) { msg.encryptWord(token); token = strtok_s(NULL, delim, &next_token); } } in.close(); cout << "\nA new file will be created that contains the encrypted message.\n\n"; cout << "Please enter the name of the new file to create: "; getline(cin, filename); msg.makeFile(filename); cout << "\n\nEncrypted file:\n"; msg.showWords(); } } else if (opt == 2) { cout << "\nEnter the name of the file to decrypt: "; getline(cin, filename); ifstream in(filename); if (!in) { cout << "File not found!!!\n"; } else { while (!in.eof()) { in.getline(buff, 1000); const char* delim = " "; char* next_token; token = strtok_s(buff, delim, &next_token); while (token) { msg.decryptWord(token); token = strtok_s(NULL, delim, &next_token); } } in.close(); cout << "\nA new file will be created that contains the decrypted message.\n\n"; cout << "Please enter the name of the new file to create: "; getline(cin, filename); msg.makeFile(filename); cout << "\n\nDecrypted file:\n"; msg.showWords(); } } cout << endl; opt = getMenu(); } cout << "\n\nThank You!!\n"; return 0; } //Implementation of menu unction int getMenu() { int opt; cout << "\tVigenre Cypher\n\n Main Menu\n\n1 - Encrypt File\n2 - Decrypt File\n3 - Quit\n\n"; cout << "Selection: "; cin >> opt; while (opt < 1 || opt>3) { cout << "\nWrong choice!!!\n\nSelection: "; cin >> opt; } cin.ignore(); return opt; } Why wont it work Please help me
Vigenere.h
#include<string>
using namespace std;
//Create class Vigenere
class Vigenere {
//Members
private:
string key;
//Functions
public:
//Constructor
Vigenere();
//Setter and getter
void setKey(string key);
string getKey();
//Convert into upper case
string toUpperCase(string k);
//Encrypt
string encrypt(string word);
//Decrypt
string decrypt(string word);
};
Vigenere.cpp
//Implementation
#include "Vigenere.h"
//Constructor
Vigenere::Vigenere() {
this->key = "";
}
//Setter and getter
void Vigenere::setKey(string key) {
this->key = key;
}
string Vigenere::getKey() {
return key;
}
//Convert into upper case
string Vigenere::toUpperCase(string k) {
for (int i = 0; i < k.length(); i++) {
if (isalpha(k[i])) {
k[i] = toupper(k[i]);
}
}
return k;
}
//Encrypt
string Vigenere::encrypt(string word) {
return key;
string output = "";
for (int i = 0, j = 0; i < word.length(); i++) {
char c = word[i];
if (c >= 'a' && c <= 'z') {
c += 'A' - 'a';
}
else if (c < 'A' || c>'Z') {
continue;
}
output += (c + key[j] - 2 * 'A') % 26 + 'A';
j = (j + 1) % key.length();
}
return output;
}
//Decrypt
string Vigenere::decrypt(string word) {
string output = "";
for (int i = 0, j = 0; i < word.length(); i++) {
char c = word[i];
if (c >= 'a' && c <= 'z') {
c += 'A' - 'a';
}
else if (c < 'A' || c>'Z') {
continue;
}
output += (c - key[j] + 26) % 26 + 'A';
j = (j + 1) % key.length();
}
return output;
}
Message.h
#include<vector>
#include "Vigenere.h"
//Create class
class Message {
//Members
private:
vector<string>words;
Vigenere v;
//Functions
public:
//Constructor
Message(string key);
//Encrypt
void encryptWord(char* token);
//Decrypt
void decryptWord(char* token);
//Make a file
void makeFile(string fileName);
//Display
void showWords();
};
Message.cpp
//Implementation of Message class
#include "Message.h"
#include<fstream>
#include<iostream>
//Constructor
Message::Message(string key) {
v.setKey(key);
}
//Encrypt
void Message::encryptWord(char* token) {
words.push_back(v.encrypt(token));
}
//Decrypt
void Message::decryptWord(char* token) {
words.push_back(v.decrypt(token));
}
//Make a file
void Message::makeFile(string fileName) {
ofstream out(fileName);
for (int i = 0; i < words.size(); i++) {
out << words[i] << " ";
}
out << endl;
out.close();
}
//Display
void Message::showWords() {
for (int i = 0; i < words.size(); i++) {
cout << words[i] << " ";
}
cout << endl;
}
VigenerCypherDriver.cpp
#include <iostream>
#include<fstream>
#include<string>
#include "Message.h"
//Function prototype
int getMenu();
int main()
{
string key, filename, line;
char buff[1000];
char* token = NULL;
//Prompt for key
cout << "Enter an encryption/decryption key:\n";
getline(cin, key);
cout << endl;
//Create message object
Message msg(key);
//Get choice
int opt = getMenu();
//Loop until quit
while (opt != 3) {
if (opt == 1) {
cout << "\nEnter the name of the file to encrypt: ";
getline(cin, filename);
ifstream in(filename);
if (!in) {
cout << "File not found!!!\n";
}
else {
while (!in.eof()) {
in.getline(buff, 1000);
const char* delim = " ";
char* next_token;
token = strtok_s(buff, delim, &next_token);
while (token) {
msg.encryptWord(token);
token = strtok_s(NULL, delim, &next_token);
}
}
in.close();
cout << "\nA new file will be created that contains the encrypted message.\n\n";
cout << "Please enter the name of the new file to create: ";
getline(cin, filename);
msg.makeFile(filename);
cout << "\n\nEncrypted file:\n";
msg.showWords();
}
}
else if (opt == 2) {
cout << "\nEnter the name of the file to decrypt: ";
getline(cin, filename);
ifstream in(filename);
if (!in) {
cout << "File not found!!!\n";
}
else {
while (!in.eof()) {
in.getline(buff, 1000);
const char* delim = " ";
char* next_token;
token = strtok_s(buff, delim, &next_token);
while (token) {
msg.decryptWord(token);
token = strtok_s(NULL, delim, &next_token);
}
}
in.close();
cout << "\nA new file will be created that contains the decrypted message.\n\n";
cout << "Please enter the name of the new file to create: ";
getline(cin, filename);
msg.makeFile(filename);
cout << "\n\nDecrypted file:\n";
msg.showWords();
}
}
cout << endl;
opt = getMenu();
}
cout << "\n\nThank You!!\n";
return 0;
}
//Implementation of menu unction
int getMenu() {
int opt;
cout << "\tVigenre Cypher\n\n Main Menu\n\n1 - Encrypt File\n2 - Decrypt File\n3 - Quit\n\n";
cout << "Selection: ";
cin >> opt;
while (opt < 1 || opt>3) {
cout << "\nWrong choice!!!\n\nSelection: ";
cin >> opt;
}
cin.ignore();
return opt;
}
Why wont it work Please help me


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

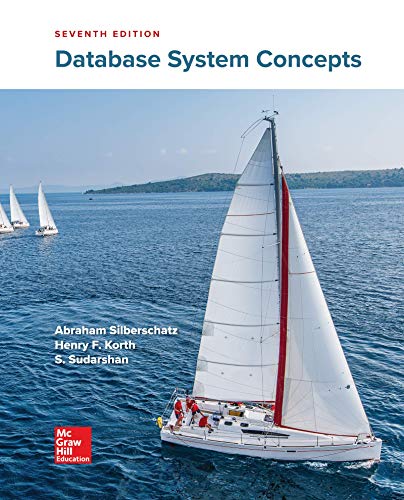
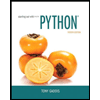
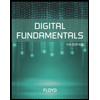
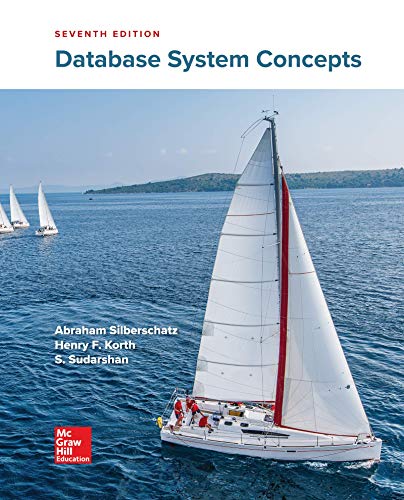
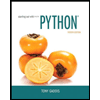
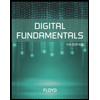
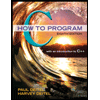
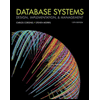
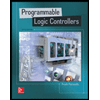